Both are greedy algorithm to Find the MST. How ever let me show the difference with the help of table:
Both are greedy algorithm to Find the MST. How ever let me show the difference with the help of table:
The basic difference is in which edge you choose to add next to the spanning tree in each step.
In Prim's, you always keep a connected component, starting with a single vertex. You look at all edges from the current component to other vertices and find the smallest among them. You then add the neighbouring vertex to the component, increasing its size by 1. In N-1 steps, every vertex would be merged to the current one if we have a connected graph.
In Kruskal's, you do not keep one connected component but a forest. At each stage, you look at the globally smallest edge that does not create a cycle
The basic difference is in which edge you choose to add next to the spanning tree in each step.
In Prim's, you always keep a connected component, starting with a single vertex. You look at all edges from the current component to other vertices and find the smallest among them. You then add the neighbouring vertex to the component, increasing its size by 1. In N-1 steps, every vertex would be merged to the current one if we have a connected graph.
In Kruskal's, you do not keep one connected component but a forest. At each stage, you look at the globally smallest edge that does not create a cycle in the current forest. Such an edge has to necessarily merge two trees in the current forest into one. Since you start with N single-vertex trees, in N-1 steps, they would all have merged into one if the graph was connected.
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
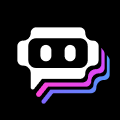
Kruskal's and Prim's algorithms are both used to find the Minimum Spanning Tree (MST) of a graph, but they employ different approaches to achieve this goal. Here’s a concise comparison of the two:
Kruskal's Algorithm
- Approach: Edge-centric.
- Process:
- Sort all edges in the graph by their weights.
- Initialize an empty forest (a collection of trees).
- Add edges to the forest, starting with the smallest, ensuring that no cycles are formed (using a union-find data structure).
- Continue adding edges until there are [math]V-1[/math] edges in the forest (where [math]V[/math] is the number of vertices). - Complexity: [math][/math]
Kruskal's and Prim's algorithms are both used to find the Minimum Spanning Tree (MST) of a graph, but they employ different approaches to achieve this goal. Here’s a concise comparison of the two:
Kruskal's Algorithm
- Approach: Edge-centric.
- Process:
- Sort all edges in the graph by their weights.
- Initialize an empty forest (a collection of trees).
- Add edges to the forest, starting with the smallest, ensuring that no cycles are formed (using a union-find data structure).
- Continue adding edges until there are [math]V-1[/math] edges in the forest (where [math]V[/math] is the number of vertices). - Complexity: [math]O(E \log E)[/math], where [math]E[/math] is the number of edges (dominated by the sorting step).
- Best for: Sparse graphs, as it focuses on edges.
Prim's Algorithm
- Approach: Vertex-centric.
- Process:
- Start with a single vertex and grow the MST one edge at a time.
- Maintain a priority queue (or a min-heap) to select the smallest edge connecting the growing MST to any vertex not yet included.
- Add the selected edge and the connected vertex to the MST.
- Repeat until all vertices are included. - Complexity: [math]O(E \log V)[/math] with a priority queue, where [math]V[/math] is the number of vertices.
- Best for: Dense graphs, as it focuses on vertices.
Summary
- Kruskal's is more efficient for sparse graphs and works by adding edges, while Prim's is better for dense graphs and works by expanding a growing tree of vertices. Both algorithms ultimately achieve the same result: the minimum spanning tree of a graph.
Both Prim's algorithm and Kruskal's algorithm are greedy algorithmsfor finding the Minimum Spanning Tree. For Prim's algorithm, the graph has to be connected, but that is not true in the case of Kruskal's algorithm. In Prim's algorithm, the next edge in the MST shall be the cheapest edge in the current vertex.
What is the difference between Kruskal’s and Prim’s Algorithm?
• Prim’s algorithm initializes with a node, whereas Kruskal’s algorithm initiates with an edge.
• Prim’s algorithms span from one node to another while Kruskal’s algorithm select the edges in a way that the position of the edge
Both Prim's algorithm and Kruskal's algorithm are greedy algorithmsfor finding the Minimum Spanning Tree. For Prim's algorithm, the graph has to be connected, but that is not true in the case of Kruskal's algorithm. In Prim's algorithm, the next edge in the MST shall be the cheapest edge in the current vertex.
What is the difference between Kruskal’s and Prim’s Algorithm?
• Prim’s algorithm initializes with a node, whereas Kruskal’s algorithm initiates with an edge.
• Prim’s algorithms span from one node to another while Kruskal’s algorithm select the edges in a way that the position of the edge is not based on the last step.
• In prim’s algorithm, graph must be a connected graph while the Kruskal’s can function on disconnected graphs too.
• Prim’s algorithm has a time complexity of O(V^2), and Kruskal’s time complexity is O(logV).
Here is the code for implementation of prim's algorithm:
Here is the code for implementation of prim's algorithm:
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is e
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is expanded at each step by adding the least weighted edge possible.
The prim's algorithm performs admirably on the dense graph (Graph having a large number of edges). It only generates a connected tree. The algorithm may include three types: tree vertices, fringe vertices, and unseen vertices. Tree vertices are the vertices that make up the Minimum spanning tree' T.' Fringe vertices are adjacent tree vertex vertices that are not part of the 'T.' Unseen vertices do not fall into the preceding categories (tree and fringe).
Steps involved in a Prim's Algorithm
Choose a root vertex.
Select the edge with the lowest weight that connects the tree and fringe vertex.
Add the newly chosen vertex and edge to the Minimum spanning tree T.
Repeat steps 2 and 3 until the MST has n-1 (where n is the number of vertices) edges.
Kruskal's Algorithm Definition
Kruskal's algorithm is yet another greedy method for producing the MST (Minimum Spanning Tree). Joseph Kruskal came up with it. The algorithm's goal is to find the subset of the graph that includes every vertex. It works by first treating each node as a set of 'n' distinct partial trees. Following each successive step, two disjoint partial trees are connected into a single partial tree via an edge with the lowest weight. If the edge does not form any cycles, it is added. This process is repeated until n-1 iterations are completed.
The algorithm's time complexity is O. (log V). There is no requirement to create a connected graph. When the generating Minimum spanning tree is disconnected, it is referred to as a minimum spanning forest. A forest is a grouping of trees. Kruskal's algorithm is preferred when the graph is sparse, meaning it has fewer edges.
The steps in Kruskal's Algorithm
A forest with m trees is created.
Choose the cheapest edge that connects two trees without forming a cycle.
Remove the newly added edge from the list.
Repeat the steps until (n-1) edges have been added.
Differences Between the Prim and Kruskal Algorithms
- Prim's algorithm works by selecting adjacent vertices from a set of vertices. Kruskal's algorithm, on the other hand, selects the edges with the lowest weights rather than using an adjacency list.
- Prim's algorithm generates the Minimum spanning tree by selecting graph vertices and starting with a vertex, whereas Kruskal's algorithm depends on edges and starts with an edge.
- Prim's algorithm always generates MST with connected components, whereas Kruskal's algorithm may generate MST without connected components (i.e., Minimum spanning forest).
- In the sparse graph, Kruskal's algorithm runs faster. On the other hand, Prim's algorithm outperforms the dense graph.
- Prim's algorithm has a time complexity O(V^2). On the other hand, Kruskal's algorithm runs in O(log V) time.
- Prim's algorithm requires the selection of adjacent vertices, whereas Kruskal's algorithm does not have such restrictions on selection criteria.
Prim’s algorithm results in a minimum spanning tree, a minimum weight connected graph with no cycles. Create an array A[] for the nodes that have been visited. Pick an arbitrary node, x, and start the algorithm at x. Add A[x]. Prims is a greedy algorithm so we choose the smallest edge that connects to the new unvisited node. Add this new node to the list, expanding A[]. Now we look at all the nodes reachable from A[] and pick the shortest edge. If all edges are the same length then we pick one. We continue this process, picking the smallest edge connecting to an unvisited node until the larges
Prim’s algorithm results in a minimum spanning tree, a minimum weight connected graph with no cycles. Create an array A[] for the nodes that have been visited. Pick an arbitrary node, x, and start the algorithm at x. Add A[x]. Prims is a greedy algorithm so we choose the smallest edge that connects to the new unvisited node. Add this new node to the list, expanding A[]. Now we look at all the nodes reachable from A[] and pick the shortest edge. If all edges are the same length then we pick one. We continue this process, picking the smallest edge connecting to an unvisited node until the largest edge has been chosen and node has been added to A[]. By the end, all the nodes are connected in a tree giving the minimum spanning tree’s total edge weight when adding up the nodes in A[]. The total running time is O(m log n) where m=number of entries.
Kruskal’s uses a fairly similar idea to Prim’s. We use a heap H to store the edges, with their weight as the key. We use the Find data structure for maintaining disjoint sets to keep track of the connectivity of nodes via paths containing only edges in F. Two nodes are in the same set if and only if there is a path that connects them and contains only edges in F. At most running time is O(m log n) where m=number of entries
Both Prim's algorithm and Kruskal's algorithm are greedy algorithms for finding the Minimum Spanning Tree. For Prim's algorithm, the graph has to be connected, but that is not true in the case of Kruskal's algorithm.
In Prim's algorithm, the next edge in the MST shall be the cheapest edge in the current vertex. In Kruskal's algorithm, we shall choose the cheapest edge, but it may not be in the curr
Both Prim's algorithm and Kruskal's algorithm are greedy algorithms for finding the Minimum Spanning Tree. For Prim's algorithm, the graph has to be connected, but that is not true in the case of Kruskal's algorithm.
In Prim's algorithm, the next edge in the MST shall be the cheapest edge in the current vertex. In Kruskal's algorithm, we shall choose the cheapest edge, but it may not be in the curre...
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Mos
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Most people just stick with the same insurer year after year, but that’s what the companies are counting on. This guy used tools like Coverage.com to compare rates every time his policy came up for renewal. It only took him a few minutes, and he said he’d saved hundreds each year by letting insurers compete for his business.
Click here to try Coverage.com and see how much you could save today.
2. Take advantage of safe driver programs
He mentioned that some companies reward good drivers with significant discounts. By signing up for a program that tracked his driving habits for just a month, he qualified for a lower rate. “It’s like a test where you already know the answers,” he joked.
You can find a list of insurance companies offering safe driver discounts here and start saving on your next policy.
3. Bundle your policies
He bundled his auto insurance with his home insurance and saved big. “Most companies will give you a discount if you combine your policies with them. It’s easy money,” he explained. If you haven’t bundled yet, ask your insurer what discounts they offer—or look for new ones that do.
4. Drop coverage you don’t need
He also emphasized reassessing coverage every year. If your car isn’t worth much anymore, it might be time to drop collision or comprehensive coverage. “You shouldn’t be paying more to insure the car than it’s worth,” he said.
5. Look for hidden fees or overpriced add-ons
One of his final tips was to avoid extras like roadside assistance, which can often be purchased elsewhere for less. “It’s those little fees you don’t think about that add up,” he warned.
The Secret? Stop Overpaying
The real “secret” isn’t about cutting corners—it’s about being proactive. Car insurance companies are counting on you to stay complacent, but with tools like Coverage.com and a little effort, you can make sure you’re only paying for what you need—and saving hundreds in the process.
If you’re ready to start saving, take a moment to:
- Compare rates now on Coverage.com
- Check if you qualify for safe driver discounts
- Reevaluate your coverage today
Saving money on auto insurance doesn’t have to be complicated—you just have to know where to look. If you'd like to support my work, feel free to use the links in this post—they help me continue creating valuable content.
Almost every difference between the two is already mentioned in the other answers.
One advantage of Prim's algorithm is that it has a version which runs in O(V^2). Consider a graph with V vertices and V*(V-1)/2 edges (complete graph). Then Kruskal's runs in O(ElogV) = O(V^2logV), while Prim's runs in O(V^2) when we don't use binary heap. So if E ~ V^2 (the graph is dense) then this version of Prim's algorithm which is O(V^2) can be used.
Also, at any instant, prim's algorithm gives connected component as well as it works only on connected graph in its basic form but kruskal's algorithm can gener
Almost every difference between the two is already mentioned in the other answers.
One advantage of Prim's algorithm is that it has a version which runs in O(V^2). Consider a graph with V vertices and V*(V-1)/2 edges (complete graph). Then Kruskal's runs in O(ElogV) = O(V^2logV), while Prim's runs in O(V^2) when we don't use binary heap. So if E ~ V^2 (the graph is dense) then this version of Prim's algorithm which is O(V^2) can be used.
Also, at any instant, prim's algorithm gives connected component as well as it works only on connected graph in its basic form but kruskal's algorithm can generate forest(disconnected components) at any instant as well as it can work on disconnected components without any modification. Prim's can also be easily modified to work for disconnected graph, but Kruskal works without modifications. Minimum spanning tree of the entire graph is equal to sum of minimum spanning tree's of each of it's disconnected components, therefore Prim's works in this case.
In terms of visualizing the process of construction the difference is....
In Prim's algorithm at any point of time, the set of selected edges will form a single tree.
In Kruskal's algorithm at any point of time, the set of selected edges need not belong to the same tree. But at the end we will have a single spanning tree.
In terms of implementation the difference is...
In Kruskal's every edge is considered only once. Either it is selected or rejected.
In Prim's certain edges are considered more than once. So in Kruskal's implementation we can use heap structure for selecting the next best edg
In terms of visualizing the process of construction the difference is....
In Prim's algorithm at any point of time, the set of selected edges will form a single tree.
In Kruskal's algorithm at any point of time, the set of selected edges need not belong to the same tree. But at the end we will have a single spanning tree.
In terms of implementation the difference is...
In Kruskal's every edge is considered only once. Either it is selected or rejected.
In Prim's certain edges are considered more than once. So in Kruskal's implementation we can use heap structure for selecting the next best edge efficiently where as in Prim's it is not possible.
Kruskal's and Prims are two of the very popular methods for finding minimum spanning trees (MST) in a graph. A spanning tree refers to a subgraph of the original graph, which is a tree in nature and connects all the vertices of the graph. Kruskal's and Prims are two very popular methods for finding the minimum spanning tree in a graph.
Kruskal's Algorithm
The Kruskal algorithm is a greedy algorithm for finding the minimum spanning tree. Kruskal's algorithm starts with an empty set of edges and continuously adds edges to this set until all the vertices form a tree. The tree obtained by following
Kruskal's and Prims are two of the very popular methods for finding minimum spanning trees (MST) in a graph. A spanning tree refers to a subgraph of the original graph, which is a tree in nature and connects all the vertices of the graph. Kruskal's and Prims are two very popular methods for finding the minimum spanning tree in a graph.
Kruskal's Algorithm
The Kruskal algorithm is a greedy algorithm for finding the minimum spanning tree. Kruskal's algorithm starts with an empty set of edges and continuously adds edges to this set until all the vertices form a tree. The tree obtained by following this approach is one of the minimum spanning trees of the graph. The steps are:
- Sort the edges in the non-decreasing order of their weights.
- Initialize an empty set of edges to store the minimum spanning tree.
- Repeat the following steps until the edge set contains n-1 edges (n is the number of vertices).
- Pick the edge with the lowest weight and check if it creates a cycle in the MST. If it does not create a cycle, add the edge to our set. Otherwise, ignore it.
- Merge the sets of vertices on both ends of the edges.
The time complexity of Kruskal's algorithms is determined by the sorting operation done on the edges, and it comes out to be E*log(E), where E is the number of edges in the graph.
Prim's Algorithm
Prim's algorithm is also a greedy algorithm that constructs the MST by adding vertices one by one until all the vertices are done. In Prim's algorithms, we can start with any random vertex and continuously add the minimum weight edge that connects to a vertex that is not in our vertex set. The steps are:
- Choose an arbitrary starting vertex.
- Initialize a set to store the MST.
- Initialize the min heap to store the edges of the graph and add all the edges associated to the starting vertex in the priority queue.
- Repeat the following steps until the edge set contains n-1 edges (n is the number of vertices).
- Pick up the lowest-weight edge from the priority queue and check if it forms a cycle in the tree. If not, then add it to the MST.
- Add all the edges connected to the new vertex in the priority queue.
The time complexity of Prim's algorithm is determined by the priority queue operations and comes out to be E+ V*log(V), where E is the number of edges and V is the number of vertices in the graph.
The choice between Kruskal's and Prim's depends on various factors like the density of the graph, edge weights, etc. Kruskal's algorithm is best suited if the graph is sparse (few edges in the graph). On the other hand, if the graph is dense and has a lot of edges, it is best to use Prim's algorithms as its complexity does not depend largely on the number of edges.
In Kruskal’s algorithm it begins with an edge, but in Prim's algorithm it start with a node.
- In Kruskal’s algorithm it begins with an edge, but in Prim's algorithm it start with a node.
- In Kruskal's Algorithm select the next edge in a haphazard way but in increasing order while in Prim's algorithm it move from one node to another.
- Kruskal's algorithm works on both connected and disconnected graph while in Prim's algorithm restricted on connected graph.
- Kruskal's has a time complexity of O(logV) while Prim's time complexity is O(V2).
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is e
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is expanded at each step by adding the least weighted edge possible.
The prim's algorithm performs admirably on the dense graph (Graph having a large number of edges). It only generates a connected tree. The algorithm may include three types: tree vertices, fringe vertices, and unseen vertices. Tree vertices are the vertices that make up the Minimum spanning tree' T.' Fringe vertices are adjacent tree vertex vertices that are not part of the 'T.' Unseen vertices do not fall into the preceding categories (tree and fringe).
Steps involved in a Prim's Algorithm
Choose a root vertex.
Select the edge with the lowest weight that connects the tree and fringe vertex.
Add the newly chosen vertex and edge to the Minimum spanning tree T.
Repeat steps 2 and 3 until the MST has n-1 (where n is the number of vertices) edges.
Kruskal's Algorithm Definition
Kruskal's algorithm is yet another greedy method for producing the MST (Minimum Spanning Tree). Joseph Kruskal came up with it. The algorithm's goal is to find the subset of the graph that includes every vertex. It works by first treating each node as a set of 'n' distinct partial trees. Following each successive step, two disjoint partial trees are connected into a single partial tree via an edge with the lowest weight. If the edge does not form any cycles, it is added. This process is repeated until n-1 iterations are completed.
The algorithm's time complexity is O. (log V). There is no requirement to create a connected graph. When the generating Minimum spanning tree is disconnected, it is referred to as a minimum spanning forest. A forest is a grouping of trees. Kruskal's algorithm is preferred when the graph is sparse, meaning it has fewer edges.
The steps in Kruskal's Algorithm
A forest with m trees is created.
Choose the cheapest edge that connects two trees without forming a cycle.
Remove the newly added edge from the list.
Repeat the steps until (n-1) edges have been added.
Differences Between the Prim and Kruskal Algorithms
- Prim's algorithm works by selecting adjacent vertices from a set of vertices. Kruskal's algorithm, on the other hand, selects the edges with the lowest weights rather than using an adjacency list.
- Prim's algorithm generates the Minimum spanning tree by selecting graph vertices and starting with a vertex, whereas Kruskal's algorithm depends on edges and starts with an edge.
- Prim's algorithm always generates MST with connected components, whereas Kruskal's algorithm may generate MST without connected components (i.e., Minimum spanning forest).
- In the sparse graph, Kruskal's algorithm runs faster. On the other hand, Prim's algorithm outperforms the dense graph.
- Prim's algorithm has a time complexity O(V^2). On the other hand, Kruskal's algorithm runs in O(log V) time.
- Prim's algorithm requires the selection of adjacent vertices, whereas Kruskal's algorithm does not have such restrictions on selection criteria.
The shortest spanning tree for a linked, weighted graph with no cycles is what Prim's algorithm looks for. The method was developed in 1938 by Vojtek Jarnik, and Robert Prim later rediscovered it. The procedure starts by choosing a root node, r, then spreads along neighbouring vertices by selecting the edges with the lowest weight, and so on until all vertices have been visited. The algorithm for prim has a worst-case time complexity of O. (log V2). From the collection of edges with the lowest cost, a single tree is built. The greedy technique is used by the algorithm, which expands the tree a
The shortest spanning tree for a linked, weighted graph with no cycles is what Prim's algorithm looks for. The method was developed in 1938 by Vojtek Jarnik, and Robert Prim later rediscovered it. The procedure starts by choosing a root node, r, then spreads along neighbouring vertices by selecting the edges with the lowest weight, and so on until all vertices have been visited. The algorithm for prim has a worst-case time complexity of O. (log V2). From the collection of edges with the lowest cost, a single tree is built. The greedy technique is used by the algorithm, which expands the tree at each step by include the fewest weighted edges.
On the dense graph, the prim's algorithm performs superbly (Graph having a large number of edges). Only a connected tree is produced by it. Tree vertices, fringe vertices, and unseen vertices are three types of vertices that the method may use. The vertices that make up the Minimum spanning tree' T' are known as tree vertices. The surrounding tree vertex vertices known as fringe vertices are not a part of the "T." The aforementioned categories do not apply to unseen vertices (tree and fringe).
Decide on a root vertex.
Choose the edge connecting the tree and fringe vertex that has the lowest weight.
To the Minimum spanning tree T, add the recently selected vertex and edge.
Till the MST has n-1 (where n is the number of vertices) edges, repeat steps 2 and 3.
Kruskal's algorithm is yet another opportunistic way to create the MST (Minimum Spanning Tree). It was created by Joseph Kruskal. The objective of the algorithm is to identify the subset of the graph that contains each vertex. It functions by initially seeing each node as a collection of 'n' unique incomplete trees. Two separate partial trees are joined into a single partial tree by the edge with the lowest weight after each succeeding step. The edge is added if no cycles are formed along it. Up until n-1 repetitions have been finished, this process is repeated.
The time complexity of the algorithm is O. (log V). Making a connected graph is not necessary. A minimum spanning tree is what is created when the generating minimum spanning tree is disconnected.
The method used by Prim's algorithm is to choose nearby vertices from a group of vertices. As opposed to using an adjacency list, Kruskal's algorithm chooses the edges with the lowest weights.
In contrast to Kruskal's algorithm, which depends on edges and starts with an edge, Prim's approach builds the Minimum spanning tree by choosing graph vertices and starting with a vertex.
Kruskal's approach has the potential to produce MST without connected components, while Prim's technique always produces MST with connected components (i.e., Minimum spanning forest).
The Kruskal's algorithm operates more quickly in the sparse graph. However, Prim's algorithm performs better than the dense graph.
The temporal complexity of Prim's algorithm is O(V2). Kruskal's approach, on the other hand, takes O(log V) time to complete.
In order to use Prim's algorithm, adjacent vertices must be chosen,
While searching the answer for this question, I stumbled through a video. I think that could be helpful fot you:
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is e
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is expanded at each step by adding the least weighted edge possible.
The prim's algorithm performs admirably on the dense graph (Graph having a large number of edges). It only generates a connected tree. The algorithm may include three types: tree vertices, fringe vertices, and unseen vertices. Tree vertices are the vertices that make up the Minimum spanning tree' T.' Fringe vertices are adjacent tree vertex vertices that are not part of the 'T.' Unseen vertices do not fall into the preceding categories (tree and fringe).
Steps involved in a Prim's Algorithm
Choose a root vertex.
Select the edge with the lowest weight that connects the tree and fringe vertex.
Add the newly chosen vertex and edge to the Minimum spanning tree T.
Repeat steps 2 and 3 until the MST has n-1 (where n is the number of vertices) edges.
Kruskal's Algorithm Definition
Kruskal's algorithm is yet another greedy method for producing the MST (Minimum Spanning Tree). Joseph Kruskal came up with it. The algorithm's goal is to find the subset of the graph that includes every vertex. It works by first treating each node as a set of 'n' distinct partial trees. Following each successive step, two disjoint partial trees are connected into a single partial tree via an edge with the lowest weight. If the edge does not form any cycles, it is added. This process is repeated until n-1 iterations are completed.
The algorithm's time complexity is O. (log V). There is no requirement to create a connected graph. When the generating Minimum spanning tree is disconnected, it is referred to as a minimum spanning forest. A forest is a grouping of trees. Kruskal's algorithm is preferred when the graph is sparse, meaning it has fewer edges.
The steps in Kruskal's Algorithm
A forest with m trees is created.
Choose the cheapest edge that connects two trees without forming a cycle.
Remove the newly added edge from the list.
Repeat the steps until (n-1) edges have been added.
Differences Between the Prim and Kruskal Algorithms
- Prim's algorithm works by selecting adjacent vertices from a set of vertices. Kruskal's algorithm, on the other hand, selects the edges with the lowest weights rather than using an adjacency list.
- Prim's algorithm generates the Minimum spanning tree by selecting graph vertices and starting with a vertex, whereas Kruskal's algorithm depends on edges and starts with an edge.
- Prim's algorithm always generates MST with connected components, whereas Kruskal's algorithm may generate MST without connected components (i.e., Minimum spanning forest).
- In the sparse graph, Kruskal's algorithm runs faster. On the other hand, Prim's algorithm outperforms the dense graph.
- Prim's algorithm has a time complexity O(V^2). On the other hand, Kruskal's algorithm runs in O(log V) time.
- Prim's algorithm requires the selection of adjacent vertices, whereas Kruskal's algorithm does not have such restrictions on selection criteria.
Prim always joins a "new" vertex to an "old" vertex, so that every stage is a tree. Kruskal's allows both "new" to "new" and "old" to "old" to get connected, so it risks creating a circuit and must check for them every time. So Kruskal's has a larger complexity than Prim.
Prim’s algorithm adds the edge with least cost that connects a new vertex to the MST. Kruskal’s algorithm, adds the edge with least cost that doesn’t form a circle. Prim’s algorithm takes O(|V|log(|V|)) for adding all the vertices in the min heap and O(|E|) for examining all edges. Kruskal needs O(|E|) to heapify all edges and O(|E|log(|E|)) for selecting vertices (at the worst case) plus O(|E|) to check for cycles.
- Order of time
Computing time of kruskal’s algorithm is O(E log n) where E is the no. of edge and prime algorithms is O(n square)…The algorithms spend most of its time in finding the smallest edge. So,time of the algorithm basically depend on how do we search this edge.
First, the similarities: Prim’s and Kruskal’s algorithms both find the minimum spanning tree in a weighted, undirected graph. They are both considered greedy algorithms, because at each they add the smallest edge from a given set of edges. The best implementations of each also have the same big O time complexity: [math]O(E\mbox{ log}(V))[/math].
The two algorithms are different in how they find this tree.
Prim’s algorithm grows a single tree. The tree starts as a single node. At each step, it adds an edge from this tree to a new node; it always chooses the smallest edge that can be added without creating a c
First, the similarities: Prim’s and Kruskal’s algorithms both find the minimum spanning tree in a weighted, undirected graph. They are both considered greedy algorithms, because at each they add the smallest edge from a given set of edges. The best implementations of each also have the same big O time complexity: [math]O(E\mbox{ log}(V))[/math].
The two algorithms are different in how they find this tree.
Prim’s algorithm grows a single tree. The tree starts as a single node. At each step, it adds an edge from this tree to a new node; it always chooses the smallest edge that can be added without creating a cycle, i.e. it adds the closest node that isn't in the tree. If you were to visualize this, it would look like a tree is growing branches, ultimately reaching all nodes on the graph. At each step, the tree would grow a branch to the closest node.
Kruskal’s algorithm instead incrementally connects a forest, or a set of trees. It begins with no edges, and at each step, adds the smallest edge that can be added without creating a cycle. The difference from Prim’s is that in Kruskal’s, the edge doesn’t need to be connected to a particular subtree; instead, it is chosen from the set of all edges. If you were to visualize this, it would look like increasingly large segments are drawn onto the graph, until at the last step every node is connected. At each step, you would see multiple smaller trees get connected to form a larger tree.
Here’s a quick analysis of runtimes showing both can be done in [math]O(E\mbox{ log}(V))[/math].
With Kruskal’s, we can take the list of all edges and sort it. This happens in [math]O(E\mbox{ log}(E))[/math]. Then, we loop through the sorted edges in order, at each step adding the edge if it does not form a cycle. When using a Union-Find data structure, the complexity of checking cycles is the inverse Ackermann function, which is much faster than [math]log E[/math] for each edge, so this term is irrelevant. Therefore, Kruskal’s runs in [math]O(E\mbox{ log}(E)).[/math] We also know that the number of edges is at most [math]V*(V-1)[/math]. [math]O(E\mbox{ log}(E))[/math] is then equivalent to [math]O(E\mbox{ log}(V^2)),[/math] which is equivalent to [math]O(E\mbox{ log}(V))[/math], since an exponent in a log factors out as a constant.
With Prim’s, we start with one point. In total, we run the following [math]V-1[/math] times since that is how many edges need to be added to form a minimum spanning tree. From a queue, we take the closest node. For each of its neighbors, if the edge from this point is its closest path to the tree, it is added to the queue. Using a binary heap-based priority queue, adding neighbors can be done in [math]O(log V)[/math] time and the closest node can be accessed in constant time. Since the sum of the number of neighbors for each node is 2 times the number of edges (each edge gets added twice, once for each endpoint), this [math]O(log V) [/math]operation is run at most 2E
times. Therefore, Prim’s runs in [math]O(E\mbox{ log}(V))[/math].
It is a good exercise to try to implement both. If you have used Dijkstra’s algorithm, you will find that the implementation of Prim’s is almost identical. Implementing Kruskal’s is also a good way to get to know the Disjoint Set Union (also called Union-Find) data structure/algorithms.
Images from Wikipedia.
Use Prim's algorithm when you have a graph with lots of edges.
For a graph with V vertices E edges, Kruskal's algorithm runs in O(E log V) time and Prim's algorithm can run in O(E + V log V) amortized time, if you use a Fibonacci Heap.
Prim's algorithm is significantly faster in the limit when you've got a really dense graph with many more edges than vertices. Kruskal performs better in typical situations (sparse graphs) because it uses simpler data structures.
In kruskal’s algorithm until we have got the whole MST at each stage we get a set of forests while in prim’s algorithm at each stage set of selected edges form a tree.
Prim always joins a "new" vertex to an "old" vertex, so that every stage is a tree. Kruskal's allows both "new" to "new" and "old" to "old" to get connected, so it risks creating a circuit and must check for them every time. So Kruskal's has a larger complexity than Prim.
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is e
Definition of Prim's Algorithm
Prim's algorithm seeks the shortest spanning tree for a connected weighted graph with no cycles. Vojtek Jarnik created the algorithm in 1938, and Robert Prim later rediscovered it. The algorithm begins by selecting a root node r, then expands along adjacent vertices by choosing the lowest weight edges, and the process is repeated until all vertices are visited. The worst-case time complexity of the prim's algorithm is O(log V2). It constructs a single tree from the set of edges with the lowest cost. The algorithm employs the greedy strategy, in which the tree is expanded at each step by adding the least weighted edge possible.
The prim's algorithm performs admirably on the dense graph (Graph having a large number of edges). It only generates a connected tree. The algorithm may include three types: tree vertices, fringe vertices, and unseen vertices. Tree vertices are the vertices that make up the Minimum spanning tree' T.' Fringe vertices are adjacent tree vertex vertices that are not part of the 'T.' Unseen vertices do not fall into the preceding categories (tree and fringe).
Steps involved in a Prim's Algorithm
Choose a root vertex.
Select the edge with the lowest weight that connects the tree and fringe vertex.
Add the newly chosen vertex and edge to the Minimum spanning tree T.
Repeat steps 2 and 3 until the MST has n-1 (where n is the number of vertices) edges.
Kruskal's Algorithm Definition
Kruskal's algorithm is yet another greedy method for producing the MST (Minimum Spanning Tree). Joseph Kruskal came up with it. The algorithm's goal is to find the subset of the graph that includes every vertex. It works by first treating each node as a set of 'n' distinct partial trees. Following each successive step, two disjoint partial trees are connected into a single partial tree via an edge with the lowest weight. If the edge does not form any cycles, it is added. This process is repeated until n-1 iterations are completed.
The algorithm's time complexity is O. (log V). There is no requirement to create a connected graph. When the generating Minimum spanning tree is disconnected, it is referred to as a minimum spanning forest. A forest is a grouping of trees. Kruskal's algorithm is preferred when the graph is sparse, meaning it has fewer edges.
The steps in Kruskal's Algorithm
A forest with m trees is created.
Choose the cheapest edge that connects two trees without forming a cycle.
Remove the newly added edge from the list.
Repeat the steps until (n-1) edges have been added.
Differences Between the Prim and Kruskal Algorithms
- Prim's algorithm works by selecting adjacent vertices from a set of vertices. Kruskal's algorithm, on the other hand, selects the edges with the lowest weights rather than using an adjacency list.
- Prim's algorithm generates the Minimum spanning tree by selecting graph vertices and starting with a vertex, whereas Kruskal's algorithm depends on edges and starts with an edge.
- Prim's algorithm always generates MST with connected components, whereas Kruskal's algorithm may generate MST without connected components (i.e., Minimum spanning forest).
- In the sparse graph, Kruskal's algorithm runs faster. On the other hand, Prim's algorithm outperforms the dense graph.
- Prim's algorithm has a time complexity O(V^2). On the other hand, Kruskal's algorithm runs in O(log V) time.
- Prim's algorithm requires the selection of adjacent vertices, whereas Kruskal's algorithm does not have such restrictions on selection criteria.
BFS and DFS are graph traversal algorithms, while Kruskal's and Prim's are minimum spanning tree (MST) algorithms.
BFS stands for breadth-first search. It is an iterative algorithm that explores all of the nodes at a given level before moving on to the next level. BFS is typically implemented using a queue.
DFS stands for depth-first search. It is also an iterative algorithm, but it explores all of the nodes along a given path before backtracking and exploring other paths. DFS is typically implemented using a stack.
Kruskal's algorithm is a greedy algorithm that finds the MST of a graph by repeat
BFS and DFS are graph traversal algorithms, while Kruskal's and Prim's are minimum spanning tree (MST) algorithms.
BFS stands for breadth-first search. It is an iterative algorithm that explores all of the nodes at a given level before moving on to the next level. BFS is typically implemented using a queue.
DFS stands for depth-first search. It is also an iterative algorithm, but it explores all of the nodes along a given path before backtracking and exploring other paths. DFS is typically implemented using a stack.
Kruskal's algorithm is a greedy algorithm that finds the MST of a graph by repeatedly adding the edge with the lowest weight that does not create a cycle.
Prim's algorithm is another greedy algorithm that finds the MST of a graph by starting at a single node and repeatedly adding the edge with the lowest weight that connects the MST to a new node.
Here is a table that summarizes the key differences between the four algorithms:
Applications of BFS and DFS:
- BFS is often used to find the shortest path between two nodes in a graph.
- DFS is often used to find all of the nodes in a connected component of a graph.
- DFS is also used to perform topological sorting of a directed acyclic graph (DAG).
Applications of Kruskal's and Prim's:
- Kruskal's and Prim's algorithms are both used to find the MST of a graph.
- The MST of a graph is a subset of the graph's edges that connects all of the nodes in the graph with the minimum total weight.
- The MST can be used to solve a variety of problems, such as finding the minimum-cost network to connect a set of cities or finding the minimum-cost routing for a network of routers.
Which algorithm to use:
BFS and DFS are both efficient algorithms for graph traversal. BFS is generally better for finding the shortest path between two nodes, while DFS is generally better for finding all of the nodes in a connected component or performing topological sorting.
Kruskal's and Prim's algorithms are both efficient algorithms for finding the MST of a graph. Kruskal's algorithm is generally better for sparse graphs (graphs with few edges), while Prim's algorithm is generally better for dense graphs (graphs with many edges).
In general, the best algorithm to use depends on the specific problem you are trying to solve.
One thing to note here is that you may want to change your terminology.
Let's start with what you call your implementation of Kruskal. Here, you actually implemented a minimum spanning tree algorithm. Your step "permute the order of all edges" is equivalent to, for example, "we assign a random real number from [0,1] to each edge".
Now, given that no two edges have the same weight (which happens with probability 1 for our random reals), the minimum spanning tree is always unique. If you were to run Prim's algorithm (*) given the same set of edge weights, you would end up with exactly the same sp
One thing to note here is that you may want to change your terminology.
Let's start with what you call your implementation of Kruskal. Here, you actually implemented a minimum spanning tree algorithm. Your step "permute the order of all edges" is equivalent to, for example, "we assign a random real number from [0,1] to each edge".
Now, given that no two edges have the same weight (which happens with probability 1 for our random reals), the minimum spanning tree is always unique. If you were to run Prim's algorithm (*) given the same set of edge weights, you would end up with exactly the same spanning tree.
What you call your implementation of Prim is actually a different algorithm that only looks similar.
(*1) A more proper name to use is Jarník-Prim's algorithm. Give credit where credit is due.
The prim's algorithm selects the root vertex in the beginning and then traverses from vertex to vertex adjacently. On the other hand, Krushal's algorithm helps in generating the minimum spanning tree, initiating from the smallest weighted edge.
Prim’s algorithm is basically used to get a minimum spanning tree.
A minimum spanning tree(MST) is an acyclic tree formed by joining all the nodes and has a minimum edge weight.
This Prim’s algorithm is a greedy approach to get a global optimal solution(MST) by selecting a local optimal solution.
So it works in this way:-
- Firstly any vertex is chosen
- Then the shortest edge connected to this vertex is selected
- This is followed by selecting the shortest edge connected to any vertex already connected
- the third step repeats till all the nodes are connected
A heap data structure is used to carry out this a
Prim’s algorithm is basically used to get a minimum spanning tree.
A minimum spanning tree(MST) is an acyclic tree formed by joining all the nodes and has a minimum edge weight.
This Prim’s algorithm is a greedy approach to get a global optimal solution(MST) by selecting a local optimal solution.
So it works in this way:-
- Firstly any vertex is chosen
- Then the shortest edge connected to this vertex is selected
- This is followed by selecting the shortest edge connected to any vertex already connected
- the third step repeats till all the nodes are connected
A heap data structure is used to carry out this algorithm and the complexity is O(ElogV) and with a fibonacci heap,it is O(E+VlogV).
Here E→Edges,V→Vertices
Let us implement it here:-
So first we take any vertex:-
vertex 6 is taken and the edge connecting with 1 is chosen because the edge weight is 10 which is less than 25 for 6–5 edge.
Then 6–5 edge is selected instead of 1–2 because 25<28.
After this,5–4 edge is selected over 1–2 because 22<28.
Then 4–3 edge has the smallest weight of 12
2–3 edge has the smallest weight of 16 after 4–3
finally 2–7 edge connects all the nodes. It is also an acyclic tree so it is an MST.
The total weight is:-
10+25+22+12+16+14=99
Hence this is how Prim’s algorithm works.
I can answer a slightly different, but related question.
It is easy to modify Prim's algorithm to produce spanning trees with smaller diameter than random trees. Recall that Prim's algorithm is very similar in structure to Dijkstra's single-source shortest-path algorithm (without edge weights, Breadth-First Search can be used), so instead of selecting a random edge at every step, you can select an edge that Dijkstra's algorithm (or BFS, in your case) would select. There does not seem to be an easy and computationally-efficient modification of Kruskal's algorithm with random initial permutation
I can answer a slightly different, but related question.
It is easy to modify Prim's algorithm to produce spanning trees with smaller diameter than random trees. Recall that Prim's algorithm is very similar in structure to Dijkstra's single-source shortest-path algorithm (without edge weights, Breadth-First Search can be used), so instead of selecting a random edge at every step, you can select an edge that Dijkstra's algorithm (or BFS, in your case) would select. There does not seem to be an easy and computationally-efficient modification of Kruskal's algorithm with random initial permutation to accomplish this.
In a more general case with nontrivial edge weights, you can form a hybrid of Prim's and Dijkstra's algorithms by calculating a convex linear combination of edge length (the priority in Prim's algo) and the prospective path length (the priority in Dijkstra's algorithm). The coefficient that controls the linear combination is a nice knob to tune if you are looking for useful spanning trees. In a recent project, we used this trick to find low-stretch spanning trees in large graphs.
The intuition to most minimum spanning tree (MST) algorithms is the cut property [1]. Let me illustrate that with an example.
Understanding the Cut Property
Let us consider a connected weighted graph G, with N vertices and E edges. Suppose we divide its vertices into two parts such that one part has N-1 vertices and the other exactly one. Now, any of the edges from that one vertex to the other (N-1) vertices can be used to connect the two parts of the graph.
Since a minimum spanning tree covers all vertices by definition, that implies that it should also cover our lone vertex. Then, it is eas
The intuition to most minimum spanning tree (MST) algorithms is the cut property [1]. Let me illustrate that with an example.
Understanding the Cut Property
Let us consider a connected weighted graph G, with N vertices and E edges. Suppose we divide its vertices into two parts such that one part has N-1 vertices and the other exactly one. Now, any of the edges from that one vertex to the other (N-1) vertices can be used to connect the two parts of the graph.
Since a minimum spanning tree covers all vertices by definition, that implies that it should also cover our lone vertex. Then, it is easy to see that any MST for G should necessarily include the edge with minimum weight between the lone vertex and the other (N-1) vertices. See why? Because if it does not, then we can easily replace that edge with the minimum cost edge and obtain a lower cost spanning tree.
Once, we have this intuitive understanding, it is easy to extend it to any partition of the graph G. Say one part of the graph contains k nodes and the other, (N-k).
Then, using the same argument as above, we can claim that an MST of the graph must connect these two components of the graph and do it in minimum cost, so the lowest cost edge will surely be in the MST.
Designing the Algorithm
Different algorithms for an MST can be designed based on the cut property. The Prim algorithm, essentially, works by creating two components of the graph at each iteration and finding the minimum cost edge between them, knowing that such an edge has to be in the MST. This ensures that all the edges added using Prim's algorithm are edges of a valid MST of the graph.
Prim's Algorithm:
- Choose an arbitrary node n.
- Initialize two sets, U={n} and V=G \ {n}.
- Repeat for (N-1) steps:
- Choose the minimum cost edge (a,b) between U and V such that a belongs to U and b belongs to V.
- Remove b from V and add b to U.
It still remains to be shown that the tree thus constructed is a spanning tree.
Spanning: At each iteration, the algorithm adds a new node to the current tree (which has 1 node initially), and we run the algorithm for N-1 steps. Thus, N unique nodes get added to the tree.
Tree: One intuitive way to see that the final graph is a tree is through induction. Consider the graph containing the first node; that is a tree of one node trivially. Then at each iteration, we add a new edge connecting the current tree to a new node. Each new edge consists of a new node, so there can be no cycles.
[1] A cut (Cut (graph theory)) of a connected graph is a set of edges that divides it into two disconnected components. That's why the name.
They are very similar and often get confused! The difference between Prim’s and the nearest neighbour can be summed up by implementing both:
Prim’s on a graph to find minimum spanning tree:
- Start at certain vertex
- Choose nearest unvisited vertex (repeat)
- This can be chosen from any vertex you have visited
- End when all vertices have been visited
Nearest neighbour when used for TSP solutions:
- Start at certain vertex
- Choose nearest unvisited vertex (repeat)
- This can only be chosen from the vertex you are currently at
- Return to start when all vertices have been visited
So the main difference is that in Neare
They are very similar and often get confused! The difference between Prim’s and the nearest neighbour can be summed up by implementing both:
Prim’s on a graph to find minimum spanning tree:
- Start at certain vertex
- Choose nearest unvisited vertex (repeat)
- This can be chosen from any vertex you have visited
- End when all vertices have been visited
Nearest neighbour when used for TSP solutions:
- Start at certain vertex
- Choose nearest unvisited vertex (repeat)
- This can only be chosen from the vertex you are currently at
- Return to start when all vertices have been visited
So the main difference is that in Nearest neighbour you can only expand your selection from the vertices immediately connected to the one where you are currently at, whereas in Prim’s you can expand your selection from any vertex you have already visited.
Also it should be noted that in the case of D2 which I believe you are talking about, Nearest Neighbour is only used in improving TSP solution bounds so you have to find a path hence the returning to start.
Hope I helped :)
Feel free to ask for any help if you want to see how this works on an adjacency matrix.
The root vertex is first chosen by the prim's method, after which it moves from vertex to adjacent vertex. Krushal's approach, on the other hand, starts from the shortest weighted edge and assists in creating the minimum spanning tree.
A minimal spanning tree is created using the Kruskal algorithm for a given graph. A minimum spanning tree, nevertheless, exactly what is it? A subset of a graph called a minimum spanning tree has edges equal to the number of vertices -1 and the same number of vertices as the original graph. For the total of all edge weights in a spanning tree, it likewise has a low cost.
What we do in Kruskal ? Firstly sort the edges according to their weight. Then we choose that edge which has minimal weight. We add that edge if it makes no cycle. Thus we go forward greedily. So it is greedy approach. :)
The gr
A minimal spanning tree is created using the Kruskal algorithm for a given graph. A minimum spanning tree, nevertheless, exactly what is it? A subset of a graph called a minimum spanning tree has edges equal to the number of vertices -1 and the same number of vertices as the original graph. For the total of all edge weights in a spanning tree, it likewise has a low cost.
What we do in Kruskal ? Firstly sort the edges according to their weight. Then we choose that edge which has minimal weight. We add that edge if it makes no cycle. Thus we go forward greedily. So it is greedy approach. :)
The greedy approach is called greedy because, it takes optimal choice in each stage expecting, that will give a total optimal solution.
Only if the selected edge does not form a cycle does Kruskal's method keep adding nodes to the tree. It sorts all edges in ascending order of their edge weights. Additionally, it chooses the edge with the lowest cost first and the highest cost in order to discover the optimal global solution, the Kruskal algorithm thus makes a locally optimal decision. Because of this, it is known as a greedy algorithm.
Let's look at the example below to grasp Kruskal's algorithm better.
- Do away with all loops and parallel edges.
- The given graph should be cleared of any loops and parallel edges.
- Keep the edge with the lowest cost when there are parallel edges and discard the rest.
- Place each edge in an increasing weight sequence.
- The following step is creating a set of edges and weights and placing them in ascending weight order (cost).
- The edge with the least weight is added.
- The graph is now expanded by adding edges, starting with the one that has the least weight. We'll maintain making sure the spanning qualities are still intact throughout. If, after adding one edge, the spanning tree property is violated, we will think twice before adding the edge to the graph.
Code-
KRUSKAL(G):
A = ∅
For each vertex v ∈ G.V:
MAKE-SET(v)
For each edge (u, v) ∈ G.E ordered by increasing order by weight(u, v):
if FIND-SET(u) ≠ FIND-SET(v):
A = A ∪ {(u, v)}
UNION(u, v)
return A
With the aid of straightforward data structures, it is possible to demonstrate that Kruskal's algorithm executes in O(E log E) time, or O(E log V) time, for a graph with E edges and V vertices.
These are all different algorithms. The first two are for traversing a graph, Kruskal’s algorithm and Prim’s algorithm solve the minimum spanning tree problem.
Prim's algorithm finds a minimum spanning tree for a weighted undirected graph . It finds a subset of the edges that forms a tree which includes every vertex, where the total weight of all the edges in the tree is minimized.
Consider u ,v as nodes in a graph. The Relaxation we do in prim’s algo is below.
If node_in_mst(v)=false and graph[u][v] < dist[v] :
dist[v] = graph[u][v]; // Note here , We are just updating the distance of v with the minimum neighbor edge weight
Dijkstra’s shortest path algorithm
Given a graph and a source vertex in graph, this algorithm finds shortest paths from given source
Prim's algorithm finds a minimum spanning tree for a weighted undirected graph . It finds a subset of the edges that forms a tree which includes every vertex, where the total weight of all the edges in the tree is minimized.
Consider u ,v as nodes in a graph. The Relaxation we do in prim’s algo is below.
If node_in_mst(v)=false and graph[u][v] < dist[v] :
dist[v] = graph[u][v]; // Note here , We are just updating the distance of v with the minimum neighbor edge weight
Dijkstra’s shortest path algorithm
Given a graph and a source vertex in graph, this algorithm finds shortest paths from given source to all other nodes in the graph, producing a shortest-path tree.
Consider u ,v as nodes in a graph. The Relaxation we do in Dijkstra’s algo is below.
if node_in_shortest_path_set(v)=false and dist[u]+ graph[u][v] < dist[v]:
dist[v] = dist[u] + graph[u][v]; // Note here , We are updating the distance of v with the minimum neighbor edge weight + distance of source .
Both are greedy algorithm and have same time complexity :
The difference between these 2 algorithm is the relaxation step.
below example will help more:
Graph:
Minimum spanning Tree using Prim's algorithm:
Shortest Path Tree using Dijkstra’s shortest path algorithm considering node A as source node:
source: Prim's algorithm - Wikipedia,Dijkstra's algorithm - Wikipedia
TL;DR: Prim's algorithm and Dijkstra's algorithm rely on the same idea but solve two different problems.
Details
Prim's algorithm finds a minimum spanning tree for a graph by starting with an arbitrary node and then repeatedly adding the shortest edge that connects a new node. Invariance to the choice of starting node is a property of the minimum spanning tree problem.
Dijkstra's algorithm finds the shortest paths from a selected node to all nodes in the graph. It does this by starting with the selected node and then repeatedly adding the shortest edge that leads to a new node. Note how the choic
TL;DR: Prim's algorithm and Dijkstra's algorithm rely on the same idea but solve two different problems.
Details
Prim's algorithm finds a minimum spanning tree for a graph by starting with an arbitrary node and then repeatedly adding the shortest edge that connects a new node. Invariance to the choice of starting node is a property of the minimum spanning tree problem.
Dijkstra's algorithm finds the shortest paths from a selected node to all nodes in the graph. It does this by starting with the selected node and then repeatedly adding the shortest edge that leads to a new node. Note how the choice of starting node is important this time; it's one of the inputs of the algorithm.
Prim's algorithm takes the graph as argument, and returns a tree:
prim :: Graph -> Tree
Dijkstra's algorithm takes the graph and the starting node as arguments, and returns a function that gives shortest paths for each node:
dijkstra :: Graph -> Node -> (Node -> [Node])
They have different type signatures, so they're not equal.
I think some other answers are confusing the “intuition” of solving the MST problem with the concept of an “intuitive explanation”. It’s not that one should be explaining the mathematical results that spit out Prim’s algorithm as a consequence, it should be an attempt to provide a simple, easy to imagine, understanding of what Prim’s Algorithm is. So my answer won’t be going into the cut property or using any mathematical notation.
I usually like to explain it like it is a blob that eats the graph. See, the blob lands on some vertex in the graph, eats that, then gets bigger by stretching itself
I think some other answers are confusing the “intuition” of solving the MST problem with the concept of an “intuitive explanation”. It’s not that one should be explaining the mathematical results that spit out Prim’s algorithm as a consequence, it should be an attempt to provide a simple, easy to imagine, understanding of what Prim’s Algorithm is. So my answer won’t be going into the cut property or using any mathematical notation.
I usually like to explain it like it is a blob that eats the graph. See, the blob lands on some vertex in the graph, eats that, then gets bigger by stretching itself to other vertices to consume them. It cannot stop doing this, so it seeks out more things to eat. What should the blob do (assuming the blob even can think)? Well, pick the next vertex outside itself that is the cheapest to eat. The blob just simply eats that cheapest to eat vertex, and continues.
It ends up this is an optimal strategy for the blob to consume the entire graph. The choices the blob makes underlie as a minimum spanning tree. You can easily see how the blob might want to be lazier if the blob wanted to instead focus on travelling a shorter distance; this simply would yield Dijkstra’s Algorithm as the blob’s decisions would correspond more formally to a shortest path tree.
Prim's algorithm is a minimum spanning tree algorithm that takes as input a graph and finds the subset of that graph's edges that are connected.
Among all the trees that can be formed from the graph, form a tree that includes every vertex and has the lowest sum of weights.
How the Prim algorithm works
It belongs to a class of algorithms known as greedy algorithms, which seek the local optimum in the hopes of finding the global optimum.
We begin with one vertex and continue to add edges with the lowest weight until we reach our goal.
The following are the steps for implementing Prim's algorithm:
Begi
Prim's algorithm is a minimum spanning tree algorithm that takes as input a graph and finds the subset of that graph's edges that are connected.
Among all the trees that can be formed from the graph, form a tree that includes every vertex and has the lowest sum of weights.
How the Prim algorithm works
It belongs to a class of algorithms known as greedy algorithms, which seek the local optimum in the hopes of finding the global optimum.
We begin with one vertex and continue to add edges with the lowest weight until we reach our goal.
The following are the steps for implementing Prim's algorithm:
Begin the minimum spanning tree with a randomly chosen vertex.
Find the minimum of all the edges that connect the tree to new vertices and add it to the tree.
Repeat step 2 until we have a minimum spanning tree.
Pseudocode for Prim's Algorithm
The pseudocode for prim's algorithm demonstrates how we generate two sets of vertices, U and V-U. U contains the list of visited vertices, while V-U contains the list of unvisited vertices. By connecting the least weight edge, we move vertices from set V-U to set U one at a time.
T = ∅;
U = { 1 };
while (U ≠ V)
let (u, v) be the lowest cost edge such that u ∈ U and v ∈ V - U;
T = T ∪ {(u, v)}
U = U ∪ {v}
Code in C++
// Prim's Algorithm in C++
#include <cstring>
#include <iostream>
using namespace std;
#define INF 9999999
// number of vertices in graph
#define V 5
// create a 2d array of size 5x5
//for adjacency matrix to represent graph
int G[V][V] = {
{0, 9, 75, 0, 0},
{9, 0, 95, 19, 42},
{75, 95, 0, 51, 66},
{0, 19, 51, 0, 31},
{0, 42, 66, 31, 0}};
int main() {
int no_edge; // number of edge
// create a array to track selected vertex
// selected will become true otherwise false
int selected[V];
// set selected false initially
memset(selected, false, sizeof(selected));
// set number of edge to 0
no_edge = 0;
// the number of egde in minimum spanning tree will be
// always less than (V -1), where V is number of vertices in
//graph
// choose 0th vertex and make it true
selected[0] = true;
int x; // row number
int y; // col number
// print for edge and weight
cout << "Edge"
<< " : "
<< "Weight";
cout << endl;
while (no_edge < V - 1) {
//For every vertex in the set S, find the all adjacent vertices
// , calculate the distance from the vertex selected at step 1.
// if the vertex is already in the set S, discard it otherwise
//choose another vertex nearest to selected vertex at step 1.
int min = INF;
x = 0;
y = 0;
for (int i = 0; i < V; i++) {
if (selected[i]) {
for (int j = 0; j < V; j++) {
if (!selected[j] && G[i][j]) { // not in selected and there is an edge
if (min > G[i][j]) {
min = G[i][j];
x = i;
y = j;
}
}
}
}
}
cout << x << " - " << y << " : " << G[x][y];
cout << endl;
selected[y] = true;
no_edge++;
}
return 0;
}
One of the greedy algorithms to determine a graph's smallest spanning tree is Kruskal's Algorithm. A subgraph with all the vertices of the original graph is called a spanning tree. The spanning tree of a weighted graph for which the sum of the spanning tree's weights is minimal is known as the minimum spanning tree.
For a linked weighted graph, the least spanning tree is determined using Kruskal's Algorithm. The algorithm's primary goal is to identify the subset of edges that will allow us to pass through each graph vertex. Instead of concentrating on a global optimum, it adopts a greedy strate
One of the greedy algorithms to determine a graph's smallest spanning tree is Kruskal's Algorithm. A subgraph with all the vertices of the original graph is called a spanning tree. The spanning tree of a weighted graph for which the sum of the spanning tree's weights is minimal is known as the minimum spanning tree.
For a linked weighted graph, the least spanning tree is determined using Kruskal's Algorithm. The algorithm's primary goal is to identify the subset of edges that will allow us to pass through each graph vertex. Instead of concentrating on a global optimum, it adopts a greedy strategy that seeks the best outcome at each stage.
However, we should first comprehend the fundamental concepts such as spanning tree and minimum spanning tree before continuing on to the technique.
Minimum Spanning tree : The spanning tree in which the total weights of the edges is minimum is known as a minimum spanning tree. The weight of the spanning tree is the total of the weights assigned to its edges.
With Kruskal's algorithm, we begin with the edges that have the lowest weight and keep adding edges until we reach the desired result. The following are the steps to apply Kruskal's algorithm:
- Sort all of the edges first from low weight to high weight.
- Add the edge with the lightest weight to the spanning tree at this point. Reject the edge if it would otherwise result in a cycle.
- Once we have included all of the edges, a minimal spanning tree will be produced.
Implementation of Kruskal's Algorithm :
- #include <bits/stdc++.h>
- using namespace std;
- class DSU {
- int* parent;
- int* rank;
- public:
- DSU(int n)
- {
- parent = new int[n];
- rank = new int[n];
- for (int i = 0; i < n; i++) {
- parent[i] = -1;
- rank[i] = 1;
- }
- }
- int find(int i)
- {
- if (parent[i] == -1)
- return i;
- return parent[i] = find(parent[i]);
- }
- void unite(int x, int y)
- {
- int s1 = find(x);
- int s2 = find(y);
- if (s1 != s2) {
- if (rank[s1] < rank[s2]) {
- parent[s1] = s2;
- rank[s2] += rank[s1];
- }
- else {
- parent[s2] = s1;
- rank[s1] += rank[s2];
- }
- }
- }
- };
- class Graph {
- vector<vector<int> > edgelist;
- int V;
- public:
- Graph(int V) { this->V = V; }
- void addEdge(int x, int y, int w)
- {
- edgelist.push_back({ w, x, y });
- }
- void kruskals_mst()
- {
- // 1. Sort all edges
- sort(edgelist.begin(), edgelist.end());
- // Initialize the DSU
- DSU s(V);
- int ans = 0;
- cout << "Following are the edges in the "
- "constructed MST"
- << endl;
- for (auto edge : edgelist) {
- int w = edge[0];
- int x = edge[1];
- int y = edge[2];
- // Take this edge in MST if it does
- // not forms a cycle
- if (s.find(x) != s.find(y)) {
- s.unite(x, y);
- ans += w;
- cout << x << " -- " << y << " == " << w
- << endl;
- }
- }
- cout << "Minimum Cost Spanning Tree: " << ans;
- }
- };
- // Driver's code
- int main()
- {
- Graph g(4);
- g.addEdge(0, 1, 10);
- g.addEdge(1, 3, 15);
- g.addEdge(2, 3, 4);
- g.addEdge(2, 0, 6);
- g.addEdge(0, 3, 5);
- // Function call
- g.kruskals_mst();
- return 0;
- }
- following weighted graph
- 10
- 0--------1
- | \ |
- 6| 5\ |15
- | \ |
- 2--------3
- 4
Time Complexity: O(ElogE) or O(ElogV)
Auxiliary Space: O(V + E)
Well alright I'm not very good at these things but I'll give it a go.
Kruskal's Algorithm is best visualized when you initially consider the nodes as part of different trees themselves. You construct the MST for the total graph by always taking the next minimum edge that connects two 'different' trees.
Suppose in case of five nodes. Initially think of all of them as different trees.
Take the minimum edge.
Does it join two different trees?
- If yes then take the edge and merge those two trees.
- If no then discard that edge and move on to the next lightest edge and repeat until all edges are exhausted
Well alright I'm not very good at these things but I'll give it a go.
Kruskal's Algorithm is best visualized when you initially consider the nodes as part of different trees themselves. You construct the MST for the total graph by always taking the next minimum edge that connects two 'different' trees.
Suppose in case of five nodes. Initially think of all of them as different trees.
Take the minimum edge.
Does it join two different trees?
- If yes then take the edge and merge those two trees.
- If no then discard that edge and move on to the next lightest edge and repeat until all edges are exhausted (and all nodes are connected ofcourse).
Well idk if this counts as intuitive but just don't kill me if it isn't. Thank you.
It depends on the graph.
If graph edges’ weights are unique, then the result will be the same. Prim’s algorithm (actually Prim-Jarnik’s algorithm) will in each step choose the “lightest” edge (the one with the lowest weight that hasn’t been added yet) and add it to minimum spanning tree. Unique weights will ensure that Kruskal’s algorithm will have only one possible permutation of the weights (imagine you have weights 3, 2, 4, 1, 2, 5
, there are 2 possible permutations in which the weights are sorted: 1, 2
(the one after 3), 2
(the one after 1), 3, 4, 5
and you can swap the two 2s and the weigh
It depends on the graph.
If graph edges’ weights are unique, then the result will be the same. Prim’s algorithm (actually Prim-Jarnik’s algorithm) will in each step choose the “lightest” edge (the one with the lowest weight that hasn’t been added yet) and add it to minimum spanning tree. Unique weights will ensure that Kruskal’s algorithm will have only one possible permutation of the weights (imagine you have weights 3, 2, 4, 1, 2, 5
, there are 2 possible permutations in which the weights are sorted: 1, 2
(the one after 3), 2
(the one after 1), 3, 4, 5
and you can swap the two 2s and the weights will still be sorted) and therefore there is only one possible minimum spanning tree. If there’s only one possible MST, Prim-Jarnik’s algorithm will surely find that one MST and the result will surely be the same. If the condition of unique weights isn’t satisfied the results might (but might not) be different.
For a given graph, the Kruskal algorithm generates a minimal spanning tree. What is a minimal spanning tree exactly, though? A subset of a graph known as a minimum spanning tree has the same number of vertices as the original graph and edges equal to the number of vertices minus one. It also has a minimal cost for the sum of all edge weights in a spanning tree. How do we live in Kruskal? The edges should first be sorted by weight. Then we select the edge with the least weight. When it doesn't form a cycle, we add that edge. Therefore, we advance with greed. So the strategy is greedy.
The greedy
For a given graph, the Kruskal algorithm generates a minimal spanning tree. What is a minimal spanning tree exactly, though? A subset of a graph known as a minimum spanning tree has the same number of vertices as the original graph and edges equal to the number of vertices minus one. It also has a minimal cost for the sum of all edge weights in a spanning tree. How do we live in Kruskal? The edges should first be sorted by weight. Then we select the edge with the least weight. When it doesn't form a cycle, we add that edge. Therefore, we advance with greed. So the strategy is greedy.
The greedy strategy is so-called because it makes the best decision at each stage in the hopes that it would result in the overall best answer.
Kruskal's approach only continues to add nodes to the tree if the chosen edge does not establish a cycle. It arranges all edges according to their edge weights in ascending order. Additionally, the Kruskal algorithm makes a locally optimal choice by selecting the edge with the lowest cost first and the greatest cost last in order to find the ideal global solution. It is said to as a greedy algorithm as a result.
In order to better understand Kruskal's algorithm, let's look at the example below.
There should be no loops or parallel edges in the given graph.
When there are parallel edges, keep the edge with the lowest cost and throw away the others.
Making a set of edges and weights, then organising them in ascending weight order, is the next stage (cost).
Now, edges are added to the graph, starting with the one with the lowest weight. We'll be checking to make sure the spanning qualities are kept throughout. We shall pause before adding an edge to the graph if, after one edge is added, the spanning tree property is broken.
It is possible to show that Kruskal's algorithm runs in O(E log E) time or O(E log V) time with the use of simple data structures.
Below is the pseudocode:
KRUSKAL(G):
A = ∅
For each vertex v ∈ G.V:
MAKE-SET(v)
For each edge (u, v) ∈ G.E ordered by increasing order by weight(u, v):
if FIND-SET(u) ≠ FIND-SET(v):
A = A ∪ {(u, v)}
UNION(u, v)
return A