JavaScript provides several higher-order functions such as `map()`, `reduce()`, and `filter()` that are widely used for array manipulation. These functions are optimized for performance and readability. Here’s a closer look at their optimization and benefits:
1. `map()` Function 🔄
Purpose: `map()` creates a new array populated with the results of calling a provided function on every element in the calling array.
Optimization:
Internally optimized for performance in most JavaScript engines.
Executes the callback function once for each element in the array in order.
Example:
- const numbers = [1, 2, 3,
JavaScript provides several higher-order functions such as `map()`, `reduce()`, and `filter()` that are widely used for array manipulation. These functions are optimized for performance and readability. Here’s a closer look at their optimization and benefits:
1. `map()` Function 🔄
Purpose: `map()` creates a new array populated with the results of calling a provided function on every element in the calling array.
Optimization:
Internally optimized for performance in most JavaScript engines.
Executes the callback function once for each element in the array in order.
Example:
- const numbers = [1, 2, 3, 4, 5];
- const squared = numbers.map(num => num 2);
- console.log(squared); // [2, 4, 6, 8, 10]
2. `reduce()` Function ➕
Purpose: `reduce()` executes a reducer function on each element of the array, resulting in a single output value.
Optimization:
Highly efficient for accumulating values, as it traverses the array only once.
Optimized for complex calculations and transformations.
Example:
- const numbers = [1, 2, 3, 4, 5];
- const sum = numbers.reduce((total, num) => total + num, 0);
- console.log(sum); // 15
3. `filter()` Function 🔍
Purpose: `filter()` creates a new array with all elements that pass the test implemented by the provided function.
Optimization:
Designed for efficiency in filtering out elements.
Executes the callback function once for each element in the array.
Example:
- const numbers = [1, 2, 3, 4, 5];
- const even = numbers.filter(num => num % 2 === 0);
- console.log(even); // [2, 4]
Advantages of Using `map()`, `reduce()`, and `filter()` 🌟
1. Readability: These functions provide a clear and concise way to express array operations.
2. Immutability: They return new arrays or values, avoiding side effects and preserving the original array.
3. Functional Programming: Encourage a functional programming style, which can lead to cleaner and more maintainable code.
4. Chainability: Can be chained together to perform multiple operations in a readable manner.
Performance Considerations ⚡
- Browser Optimizations: Modern JavaScript engines (like V8 in Chrome, SpiderMonkey in Firefox) have optimized these methods for performance.
- Single Pass: Methods like `reduce()` and `filter()` traverse the array in a single pass, making them efficient for large datasets.
- Callback Overhead: While the overhead of invoking a callback for each element is minimal, it’s worth noting that for extremely performance-critical applications, manual loops might be slightly faster due to reduced function call overhead.
Conclusion 🌟
JavaScript's `map()`, `reduce()`, and `filter()` functions are already optimized for traversing arrays. They offer significant advantages in terms of readability, immutability, and functional programming practices. Modern JavaScript engines ensure that these methods are highly efficient, making them suitable for most applications.
Embrace these higher-order functions to write cleaner and more maintainable code! 🚀✨
I don't know what specific techniques are being referred to, but it smells like premature-optimization-thinking.
Strictly, a plain for loop is slightly faster if you run the two against eachother in direct comparison. However, it is generally silly to view performance in such a narrow way. Your app is very unlikely to have performance problems due to stuff like that. In reality, your app FPS is going to tank because you're accessing offsetTop somewhere and causing a DOM reflow causing work equivalent to millions of filters and maps. Even if your problem can be helped by switching map to a f
I don't know what specific techniques are being referred to, but it smells like premature-optimization-thinking.
Strictly, a plain for loop is slightly faster if you run the two against eachother in direct comparison. However, it is generally silly to view performance in such a narrow way. Your app is very unlikely to have performance problems due to stuff like that. In reality, your app FPS is going to tank because you're accessing offsetTop somewhere and causing a DOM reflow causing work equivalent to millions of filters and maps. Even if your problem can be helped by switching map to a for loop because you're iterating thousands of items per second, you could probably ask yourself if the problem is that you're... well, iterating thousands of items per second in the first place.
Fast applications are born by spending time in the profiler, not by speculating about tiny parts of ones software.
Oh, and if you like my writing, don't miss out on more of it -
follow me on Quora and Twitter (http://twitter.com/mpjme)
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
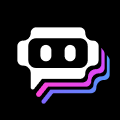
Yes, JavaScript functions like map()
, reduce()
, and filter()
are optimized for traversing arrays. These higher-order functions are implemented in the JavaScript engine and are generally efficient for their intended use cases.
Optimization Overview:
- Native Implementation: Since these methods are part of the ECMAScript specification, they are implemented in the underlying engine (like V8 for Chrome and Node.js). This means that they benefit from optimizations that can be made by the engine's developers.
- Performance: While they are optimized, the performance can vary based on the context in which t
Yes, JavaScript functions like map()
, reduce()
, and filter()
are optimized for traversing arrays. These higher-order functions are implemented in the JavaScript engine and are generally efficient for their intended use cases.
Optimization Overview:
- Native Implementation: Since these methods are part of the ECMAScript specification, they are implemented in the underlying engine (like V8 for Chrome and Node.js). This means that they benefit from optimizations that can be made by the engine's developers.
- Performance: While they are optimized, the performance can vary based on the context in which they are used. For example:
-map()
creates a new array by applying a function to each element.
-filter()
creates a new array containing elements that pass the test implemented by the provided function.
-reduce()
accumulates a single result by applying a function against an accumulator and each element. - Use Cases: These methods are generally more readable and expressive compared to traditional
for
loops, which can lead to better maintainability of the code. However, in performance-critical scenarios (like processing very large arrays), a traditional loop might outperform these methods due to reduced overhead.
Conclusion:
While map()
, reduce()
, and filter()
are optimized for traversing arrays and are typically efficient for most use cases, it's always good to measure performance in the specific context of your application if you're working with large datasets or require high performance.
Maybe this meme will help you remember the uses of Map, Filter and Reduce, for Life. :D
Pretty simple, right?
Maybe this meme will help you remember the uses of Map, Filter and Reduce, for Life. :D
Pretty simple, right?
Here we go
Filter:- The filter()
method creates a shallow copy of a portion of a given array, that contains the same elements as the ones from the original array (with some filtered out).
- const arr = [3, 5, 8, 10, 6, 1];
- // return all the elements from the array which is >= 5
- const newArr = arr.filter(element => element >= 5);
- console.log(newArr);
- // expected output:- [5, 8, 10, 6];
Map:- The map()
method is a copying method. It calls a provided callbackFn
function once for each element in an array and constructs a new array from the results.
- const arr = [2, 5, 3, 9];
- // double each element present in
Here we go
Filter:- The filter()
method creates a shallow copy of a portion of a given array, that contains the same elements as the ones from the original array (with some filtered out).
- const arr = [3, 5, 8, 10, 6, 1];
- // return all the elements from the array which is >= 5
- const newArr = arr.filter(element => element >= 5);
- console.log(newArr);
- // expected output:- [5, 8, 10, 6];
Map:- The map()
method is a copying method. It calls a provided callbackFn
function once for each element in an array and constructs a new array from the results.
- const arr = [2, 5, 3, 9];
- // double each element present in the array
- const newArr = arr.map(elem => elem * 2);
- console.log(newArr);
- // expected output:- [4, 10, 6, 81]
Reduce:- The reduce()
method executes a user-supplied "reducer" callback function on each element of the array, in order, passing in the return value from the calculation on the preceding element. The final result of running the reducer across all elements of the array is a single value.
The first time that the callback is run there is no "return value of the previous calculation". If supplied, an initial value may be used in its place. Otherwise the array element at index 0 is used as the initial value and iteration starts from the next element (index 1 instead of index 0).
- const arr = [2, 5, 3, 9];
- const initial = 0;
- const sumWithInitial = arr.reduce(
- (prev_val, curr_val) => prev_val + curr_val,
- initial
- );
- console.log(sumWithInitial);
- // expected output:- 19(0 + 2 + 5 + 3 + 9)
forEach:- The forEach()
method executes a provided function once for each array element. Unlike map(), forEach()
always returns undefined and is not chainable. forEach()
does not mutate the array on which it is called, but the function provided as callbackFn
can.
- const arr = [2, 5, 9];
- arr.forEach(element => console.log(element));
- // expected output:- 2 5 9
There are other methods like at, every, fill, find, findIndex, includes, slice, splice, and many more. You can read more about them here:- Array - JavaScript | MDN
If you like it don’t forget to upvote.
Have a good day.
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Mos
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Most people just stick with the same insurer year after year, but that’s what the companies are counting on. This guy used tools like Coverage.com to compare rates every time his policy came up for renewal. It only took him a few minutes, and he said he’d saved hundreds each year by letting insurers compete for his business.
Click here to try Coverage.com and see how much you could save today.
2. Take advantage of safe driver programs
He mentioned that some companies reward good drivers with significant discounts. By signing up for a program that tracked his driving habits for just a month, he qualified for a lower rate. “It’s like a test where you already know the answers,” he joked.
You can find a list of insurance companies offering safe driver discounts here and start saving on your next policy.
3. Bundle your policies
He bundled his auto insurance with his home insurance and saved big. “Most companies will give you a discount if you combine your policies with them. It’s easy money,” he explained. If you haven’t bundled yet, ask your insurer what discounts they offer—or look for new ones that do.
4. Drop coverage you don’t need
He also emphasized reassessing coverage every year. If your car isn’t worth much anymore, it might be time to drop collision or comprehensive coverage. “You shouldn’t be paying more to insure the car than it’s worth,” he said.
5. Look for hidden fees or overpriced add-ons
One of his final tips was to avoid extras like roadside assistance, which can often be purchased elsewhere for less. “It’s those little fees you don’t think about that add up,” he warned.
The Secret? Stop Overpaying
The real “secret” isn’t about cutting corners—it’s about being proactive. Car insurance companies are counting on you to stay complacent, but with tools like Coverage.com and a little effort, you can make sure you’re only paying for what you need—and saving hundreds in the process.
If you’re ready to start saving, take a moment to:
- Compare rates now on Coverage.com
- Check if you qualify for safe driver discounts
- Reevaluate your coverage today
Saving money on auto insurance doesn’t have to be complicated—you just have to know where to look. If you'd like to support my work, feel free to use the links in this post—they help me continue creating valuable content.
At the core they are all loops which go through each item of an array, perform an operation on that item and return something.
Where they are different is in what they return and on the operation performed on each item.
map will produce an array of the same size as the input array. The callback function will transform the item into something else, which will be in the resulting array in the same pos
At the core they are all loops which go through each item of an array, perform an operation on that item and return something.
Where they are different is in what they return and on the operation performed on each item.
map will produce an array of the same size as the input array. The callback function will transform the item into something else, which will be in the resulting array in the same position.
For example:
var a = [1,2,3,4,5,6]; a.map(d => d * 2); // [2,4,6,8,10,12]
filter will return an array with identical items as the input array, but with some items possibly removed. The callback function will return true (or a truthy value) for items that should be kept, else the item will be removed.
a.filter(d => d > 3); // [4,5,6]
reduce can return anything: a single number, an object, an array even. Its syntax is slightly different. The function it uses is called a reducer and the reduce method can also take an extra param...
map()
The map() method creates a new array with the results of calling the function once for every element of the array. Example - Finding square root of the numbers
Without map
- var numbers = [4, 9, 16, 25];
- var result = [];
- for (let i = 0; i < numbers.length; i++) {
- result.push(Math.sqrt(numbers[i]));
- }
- console.log(result);
With map
- var numbers = [4, 9, 16, 25];
- var x = numbers.map(Math.sqrt);
- console.log(x);
reduce()
The reduce() method reduces the array to a single value and called once for every element of the array. Example - Subtract the numbers in the array, starting from the beginning
Without reduc
map()
The map() method creates a new array with the results of calling the function once for every element of the array. Example - Finding square root of the numbers
Without map
- var numbers = [4, 9, 16, 25];
- var result = [];
- for (let i = 0; i < numbers.length; i++) {
- result.push(Math.sqrt(numbers[i]));
- }
- console.log(result);
With map
- var numbers = [4, 9, 16, 25];
- var x = numbers.map(Math.sqrt);
- console.log(x);
reduce()
The reduce() method reduces the array to a single value and called once for every element of the array. Example - Subtract the numbers in the array, starting from the beginning
Without reduce
- var numbers = [175, 50, 25];
- var sum = numbers[0];
- for (let i = 1; i < numbers.length; i++) {
- sum = sum - numbers[i];
- }
- console.log(sum);
With reduce
- function myFunc(total, num) {
- return total - num;
- }
- var numbers = [175, 50, 25];
- var data = numbers.reduce(myFunc);
- console.log(data);
filter()
The filter() method creates an array filled with all array elements that qualified a test scenario. Example - Filtering with age >= 18
Without filter
- var ages = [32, 33, 16, 40];
- var result = [];
- for (let i = 0; i < ages.length; i++) {
- if (ages[i] >= 18)
- result.push(ages[i]);
- }
- console.log(result);
With filter
- function checkAdult(age) {
- return age >= 18;
- }
- var ages = [32, 33, 16, 40];
- var data = ages.filter(checkAdult);
- console.log(data);
I hope it’s clear to you, the difference it makes using these functions rather than the traditional way !
I think the answer is a matter of style and way of thinking. Using [math]map[/math] enables a more declarative, functional style.
One the one hand, [math]map[/math] is more declarative. You convey the intent to perform an operation over a collection (the what) whatever this may be (array, map, set, list, etc) versus actually looping and indexing each element (the how). The first approach abstracts over the collection used, whereas the second exposes the type of collection used—[math]map[/math] is an abstraction.
In addition, using [math]map[/math] takes advantage of functors. Functors are immutable operations over types in that the “container” us
I think the answer is a matter of style and way of thinking. Using [math]map[/math] enables a more declarative, functional style.
One the one hand, [math]map[/math] is more declarative. You convey the intent to perform an operation over a collection (the what) whatever this may be (array, map, set, list, etc) versus actually looping and indexing each element (the how). The first approach abstracts over the collection used, whereas the second exposes the type of collection used—[math]map[/math] is an abstraction.
In addition, using [math]map[/math] takes advantage of functors. Functors are immutable operations over types in that the “container” used to store the information (array, map, set, list, etc.) is not mutated.
Also, [math]map[/math] is portable. Because it’s meant to abstract the underlying iterator and looping syntax, it’s portable to any programming language.
There’s some theoretical stuff in computer science that involves the study of algebraic monoids. A list or string is what’s known as a “free monoid” over some set of symbols—called the alphabet, it’s basically the type of the elements in a list.
There are lots of different concepts you can study with monoids, and it turns out many of them are pretty useful in programs. For example, “mapping” from one list to another—this is creating a new list by applying a function to every element of an old list and preserving the sequence.
It also turns out that essentially all of these operations are specifi
There’s some theoretical stuff in computer science that involves the study of algebraic monoids. A list or string is what’s known as a “free monoid” over some set of symbols—called the alphabet, it’s basically the type of the elements in a list.
There are lots of different concepts you can study with monoids, and it turns out many of them are pretty useful in programs. For example, “mapping” from one list to another—this is creating a new list by applying a function to every element of an old list and preserving the sequence.
It also turns out that essentially all of these operations are specific variations on a more general pattern, called a “fold”. The basic idea of a fold is to take a function that takes two arguments, call them type A and type B, and returns a type A, along with an element of type A and a list of type B, and applies the function to each element in the list with the “current” A element to produce a new A element. The fold then returns the final A element.
Mapping over a list, summing a list of numbers, computing the length of a list, filtering a list to keep only elements that match some predicate, and many many other operations are examples of folds.
The reduce method is an implementation of “fold” for JavaScript arrays being used to represent lists.
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off to developers.
If you prefer more customization you can also expand the power of Webflow by adding custom code on the page, in the <head>, or before the </head> of any page.
Trusted by over 60,000+ freelancers and agencies, explore Webflow features including:
- Designer: The power of CSS, HTML, and Javascript in a visual canvas.
- CMS: Define your own content structure, and design with real data.
- Interactions: Build websites interactions and animations visually.
- SEO: Optimize your website with controls, hosting and flexible tools.
- Hosting: Set up lightning-fast managed hosting in just a few clicks.
- Grid: Build smart, responsive, CSS grid-powered layouts in Webflow visually.
Discover why our global customers love and use Webflow.com | Create a custom website.
map:
- Purpose: Transforms each element in an array by applying a provided function.
- Syntax:
array.map(function(currentValue, index, arr), thisValue)currentValue
: The current element being processed.index
(optional): The index of the current element.arr
(optional): The original array.thisValue
(optional): A value to use asthis
during the callback execution. - Return value: A new array with the transformed elements.
- Example:
- const numbers = [1, 2, 3, 4, 5];
- const doubledNumbers = numbers.map(function(number) {
- return number * 2;
- }); // doubledNumbers: [2, 4, 6, 8, 10]
filter:
- Purpose: Creates a new arra
map:
- Purpose: Transforms each element in an array by applying a provided function.
- Syntax:
array.map(function(currentValue, index, arr), thisValue)currentValue
: The current element being processed.index
(optional): The index of the current element.arr
(optional): The original array.thisValue
(optional): A value to use asthis
during the callback execution. - Return value: A new array with the transformed elements.
- Example:
- const numbers = [1, 2, 3, 4, 5];
- const doubledNumbers = numbers.map(function(number) {
- return number * 2;
- }); // doubledNumbers: [2, 4, 6, 8, 10]
filter:
- Purpose: Creates a new array containing only elements that pass a test implemented by a provided function.
- Syntax:
array.filter(function(currentValue, index, arr), thisValue)
Same parameters asmap
. - Return value: A new array with the filtered elements.
- Example:
- const evenNumbers = numbers.filter(function(number) {
- return number % 2 === 0;
- }); // evenNumbers: [2, 4]
Key differences and use cases:
- Transformation vs. Selection:
map
transforms every element, whilefilter
chooses specific elements based on a condition. - New Array Creation: Both methods create a new array; they don't modify the original array.
- Chaining: You can often chain
map
andfilter
together for more complex data manipulation.
Additional considerations:
- Arrow functions provide a concise syntax for callback functions.
- Consider using
forEach
for side effects without creating a new array. reduce
can be used for more complex transformations and aggregations.
Remember, choosing the right method depends on your specific data manipulation needs. If you have further questions or require more tailored examples, feel free to ask!
Yes, I do. This is the list of reasons:
- Is faster (according to the book “High Performance Javascript”).
- Is more general than function-based iteration.
- Can be stopped.
But, those functions can make your life easier in many cases.
I saw this question and thought, is it ? Is it really ? I had a co-worker that refused to acknowledge any information on a similar issue, he was certain that a backwards for loop would always be faster. I will give the guy a credit, he is super smart, I will not deny that perhaps at very early ages, pre javascript compilers that it might have been, so his code always uses backward for loops, always. Extremely annoying. I made a huge array, and did tests running a few million times, and they were equal.
So, I think that people don’t know how to write for loops.
I honestly think it is because peop
I saw this question and thought, is it ? Is it really ? I had a co-worker that refused to acknowledge any information on a similar issue, he was certain that a backwards for loop would always be faster. I will give the guy a credit, he is super smart, I will not deny that perhaps at very early ages, pre javascript compilers that it might have been, so his code always uses backward for loops, always. Extremely annoying. I made a huge array, and did tests running a few million times, and they were equal.
So, I think that people don’t know how to write for loops.
I honestly think it is because people check length in every loop. I made two version, first I make an array:
- a = [];
- for (var i = 0, c = 10000; i < c; i++) { a.push(i); }
Then I do a for loop
- forloop = function() {
- b1 = new Date();
- for (var i=0, c=a.length; i<c; i++) {};
- b2 = new Date();
- console.log("time : ", b2.getTime()-b1.getTime());
- }
Then I do a map loop
- maploop = function() {
- b1 = new Date();
- a.map(function(i) {});
- b2 = new Date();
- console.log("time : ", b2.getTime()-b1.getTime());
- }
I of course run each loop a number of times. And frankly I am not seeing much if any difference.
- > forloop()
- time : 0
- ….
- > maploop()
- time : 0
Each operation takes 0–1 milli second. I think the once in a while 1ms time is frankly the date creation part, not the loops by themselves.
I am quite sure you can find more scientific ways.
This is how way to many people do for loops
- var i = 0;
- // a is declared like in the first code
- for (i = 0; i < a.length; i++) {};
This example will check the length of a array for every iteration.
“experience has suggested”? Strange words.
I would understand more “I have measured”… :-)
Actually, my own experience suggests… the contrary. ;-) And I don’t trust my own judgment more than your! :-D
You don’t use map, filter and all to have speed gains. You use them because they are readable, easier to understand, more compact, etc.
Beside, it probably totally depends on the browser, its JS engine, its version (they improve it daily, etc.
Why it could be slower? Perhaps because it does the same job, but adds a function call on each loop!
Why it could have the same speed? Because the JS engine might
“experience has suggested”? Strange words.
I would understand more “I have measured”… :-)
Actually, my own experience suggests… the contrary. ;-) And I don’t trust my own judgment more than your! :-D
You don’t use map, filter and all to have speed gains. You use them because they are readable, easier to understand, more compact, etc.
Beside, it probably totally depends on the browser, its JS engine, its version (they improve it daily, etc.
Why it could be slower? Perhaps because it does the same job, but adds a function call on each loop!
Why it could have the same speed? Because the JS engine might be smart enough to inline this function call…
Why it could be faster? Because the engineers recognized it becomes a frequent pattern, and spent some time to particularly optimize this use case, or trigger the Jit compiler early, etc.
Why it is not important (or rarely)? Because you will rarely notice a real difference in speed in the real world. User won’t see if an operation was completed in 2 ms instead of 3!
This can be relevant if you process gigabytes of data on Node.js, but then you will use a real profile, not gut feelings… ;-) And beware, because some optimizations can be made irrelevant by a new version of the engine!
Last note: I don’t think this applies to Array.prototype.map and similar, ie. the native JS functions. But if you use Lodash’s functions instead, and chain them, it can indeed be faster.
By chaining, I mean instead of doing var a = _.filter(data, f1); var b = _.map(a, f2);
etc., you do var r = _(data).filter(f1).map(f2).value();
It can be faster because Lodash will shortcut some operations, avoiding to make intermediary arrays, etc.
.map() allows you to create a new array by iterating over the original array and allowing you to run some sort of custom conversion function. The output from .map() is a new array.
.reduce() allows you to iterate over an array accumulating a single result or object.
These are specialized iterators. You can use them when this type of output is exactly what you want. They are less flexible than a ‘for’ loop (for example, you can't stop the iteration in the middle like you can with a ‘for’ loop), but they are less typing for specific types of operations and for people that know them, they are likel
.map() allows you to create a new array by iterating over the original array and allowing you to run some sort of custom conversion function. The output from .map() is a new array.
.reduce() allows you to iterate over an array accumulating a single result or object.
These are specialized iterators. You can use them when this type of output is exactly what you want. They are less flexible than a ‘for’ loop (for example, you can't stop the iteration in the middle like you can with a ‘for’ loop), but they are less typing for specific types of operations and for people that know them, they are likely a little easier to see the code's intent.
I have not myself tested the performance of .map() and .reduce() versus a ‘for’ loop, but have seen tests for .forEach() which showed that .forEach() was actually slower in some browsers. This is perhaps because each iteration of the loop with .forEach() has to call your callback function, whereas in a plain for loop, you do not have to make such a function call (the code can be directly embedded there). In any case, it is rare that this type of performance difference is actually meaningful and you should generally use whichever construct makes clearer, easier to maintain code.
If you really wanted to optimize performance, you would have to write your own test case in a tool like jsperf and then run it in multiple browsers to see which way of doing things was best for your particular situation.
Another advantage of a for loop is that it can be used with array-like objects such as an HTMLCollection which are not actual arrays and thus don't have methods like .reduce() and .map().
There are two ways of changing data. The first method is to directly mutate the values stored in a variable.
The second way is to create a new object and copy the values of the first variable with the desired changes. This is a concept known as immutability. This second technique is what methods like slice() and map() do.
Avoiding data mutations allows programmers to keep references to older versions of the object and allows them to change to different states when needed.
One technique is is not necessarily better than the other. Here’s a discussion similar to the question you’re asking on SE.
There are two ways of changing data. The first method is to directly mutate the values stored in a variable.
The second way is to create a new object and copy the values of the first variable with the desired changes. This is a concept known as immutability. This second technique is what methods like slice() and map() do.
Avoiding data mutations allows programmers to keep references to older versions of the object and allows them to change to different states when needed.
One technique is is not necessarily better than the other. Here’s a discussion similar to the question you’re asking on SE.
If immutable objects are good, why do people keep creating mutable objects?
If you only need those two things, I would not recommend using a library.
That said, I strongly recommend familiarizing yourself with Lodash. It is packed with very powerful utility functions that can often be combined to perform complex operations on your datasets, using very little code.
The creator of Lodash, John Dalton, is so obsessed with performance that Microsoft hired him to be in charge of JavaScript benchmarks, I think on their Edge browser.
Lodash is used by nearly everyone in the JavaScript community. As with most popular libraries, the common practice is to first attempt to use the
If you only need those two things, I would not recommend using a library.
That said, I strongly recommend familiarizing yourself with Lodash. It is packed with very powerful utility functions that can often be combined to perform complex operations on your datasets, using very little code.
The creator of Lodash, John Dalton, is so obsessed with performance that Microsoft hired him to be in charge of JavaScript benchmarks, I think on their Edge browser.
Lodash is used by nearly everyone in the JavaScript community. As with most popular libraries, the common practice is to first attempt to use the native methods. If they're available, it will use them and exit the library. If they're not available, it will provide code to achieve the desired outcome.
Guys like John have made it their job to ensure your operations run as fast as possible, consistently, and without issue across browsers. I recommend outsourcing that labor by using a library like Lodash.
Array.prototype.filter() - Array.prototype.map()
Filter, as the name implies, takes each element of an array, and returns a resulting array, where some elements of the original array might have been filtered out.
It takes a predicate, a function telling for each element if it must be kept or not. If the function returns a falsy value, the element is filtered out.
Here, since it returns contact.email, it filters out contact objects without an e-mail field (or with a null or empty value).
Map takes each element of an array, and transforms it. It returns a resulting array, of same size than the orig
Array.prototype.filter() - Array.prototype.map()
Filter, as the name implies, takes each element of an array, and returns a resulting array, where some elements of the original array might have been filtered out.
It takes a predicate, a function telling for each element if it must be kept or not. If the function returns a falsy value, the element is filtered out.
Here, since it returns contact.email, it filters out contact objects without an e-mail field (or with a null or empty value).
Map takes each element of an array, and transforms it. It returns a resulting array, of same size than the original, with the transformed elements.
Here, for each contact, it creates a React element, using the contact's information.
Let's say we have the text for the State of the Union address and we want to count the frequency of each word. You could easily do this by storing each word and its frequency in a dictionary and looping through all of the words in the speech. However, imagine if we have the text for all of Wikipedia (say, a billion words) and we wanted to do the same thing. Our poor computer would be stuck looping for ages!
MapReduce is a programming model that allows tasks (like counting frequencies) to be simultaneously (parallel) performed on many (distributed) computers. Now, instead of one computer having
Let's say we have the text for the State of the Union address and we want to count the frequency of each word. You could easily do this by storing each word and its frequency in a dictionary and looping through all of the words in the speech. However, imagine if we have the text for all of Wikipedia (say, a billion words) and we wanted to do the same thing. Our poor computer would be stuck looping for ages!
MapReduce is a programming model that allows tasks (like counting frequencies) to be simultaneously (parallel) performed on many (distributed) computers. Now, instead of one computer having to loop through a billion words we can now have 1,000 computers simultaneously looping through only a million words each -- that's a 1000x time improvement!
There are two parts to MapReduce: map(), and reduce().
Map() takes in a word and "emits" a key and value pair. In this case, key = a word, and value = 1 (we just have one instance of the word). For instance, the phrase "bright yellow socks" would emit ("bright", 1), ("yellow", 1), and (“socks”, 1). Map() is called for every single word -- all one billion of them.
(You might be asking why we did this very redundant step. Bear with me for a second!)
Now, we have one billion key-value pairs. What do we do with these?
This is where Reduce() comes in. All of the key-value pairs with the same key are combined and fed into Reduce(). Let's take "hello" for instance, and say that there are 500,000 occurrences of the word in Wikipedia. Then, we have 500,000 key-pairs that are passed into a single Reduce() call. Now, all we have to do is loop through 500,000 ("hello", 1) pairs and sum up all of the values. This is much easier than having to loop through a billion. Reduce() then "emits" another key-value pair; this time, it's ("hello", 500000), or the word, and the total sum of the values associated with that word. Repeat the Reduce() call for every other word, and we've got the frequency for every word!
By distributing this massive job to many computers, if any one of these computers fail for any reason, we can just restart the specific segment it was working on. Imagine if this happened on a single computer!
Note that this is a very simple example. MapReduce has been used on many problems that can be fit into this Map()/Reduce() model, such as Google's search index, machine learning, and statistical analysis.
MapReduce is a distributed computing paradigm. It has gained a lot of popularity as it provides a simple model for distributed computing, while ensuring data persistence and availability.
Conceptual Model:
As the name suggests, MapReduce consists of 2 components:
- Map
- Reduce
A programmer needs to specify:
- The input file. It contains a set of 1 or more key value pairs.
- The Map Function.
- The reduce Function.
Map:
- Input: The Map function takes a range of <key, value> pairs as input.
- Output: 0, 1 or more intermediate <key, value> pairs. The Keys outputted from Map function are different from input keys.
MapReduce is a distributed computing paradigm. It has gained a lot of popularity as it provides a simple model for distributed computing, while ensuring data persistence and availability.
Conceptual Model:
As the name suggests, MapReduce consists of 2 components:
- Map
- Reduce
A programmer needs to specify:
- The input file. It contains a set of 1 or more key value pairs.
- The Map Function.
- The reduce Function.
Map:
- Input: The Map function takes a range of <key, value> pairs as input.
- Output: 0, 1 or more intermediate <key, value> pairs. The Keys outputted from Map function are different from input keys.
The Map function will also group the intermediate <key, value> pairs based on the key.
Reduce:
- Input: Intermediate set of <key, value> pairs, grouped by the key, as outputted from Map function.
- Output: The reduce function will combine the values for each distinct key. It will output a set of distinct key and their corresponding combined value, <distinct key, combined value>. It can combine the keys using any mathematical function, like addition, multiplication, etc.
Example:
We have a huge document, split across several machines/nodes. We want to determine the number of times each word appears in the document.
For this example, the input <key, value> is the document and the document content.
The Mapreduce system will split the entire document into several chunks.
The chunks are split across several nodes. To ensure availability and persistence, each chunk is replicated 2x or 3x. Mapreduce implementations, like Google File System (GFS), Hadoop Distributed File System (HDFS), ensure this step.
Map Function:
- The map function will parse through the entire chunk and read each word.
- For each word, it will provide a count of 1.
- Output of map function will be a set of words, each with a count of 1. Thus, the key is a word in the file chunk, the value is 1.
- The MapReduce system will also automatically group the <key, value> pairs by the key, using a partition function (usually a hash function).
Reduce Function:
- For each word, the reduce function will add all the counts.
- The Output of reduce will be a set of distinct words, with its total count across the document.
Source: Mining Massive Datasets - Jure Leskovec, Anand Rajaraman, Jeff Ullman
map() transforms the elements of an array returning a new array with the same length, filter() filters the elements of an array into a new array containing the elements that match the filter condition, reduce() returns a single value based on the values of the elements in the array.
It always depends upon the use case, there are 2 popular iterative mechanisms available for iterating over an array.
forEach and Map.
forEach can be helpful and effective in a situation where we don’t need any return values/array after execution instead we just manipulate its element values as per need. Whereas map can be helpful in situation where we need to return a result array after performing some operations.
See below video
Using the array.forEach( ) function
- let arrayOfObjects = [
- {
- name: "node1",
- value: 5
- },
- {
- name: "node2",
- value: 5
- },
- {
- name: "node3",
- value: 5
- },
- ]
- arrayOfObjects.forEach( ( object, index ) => {
- object.value += index;
- } )
Array prototype functions are not required at all to use arrays. You could be stubborn and insist on making your life harder and your code less maintainable by reinventing the wheel, but why do this:
- let array = [1,2,3,4,5];
- let array2 = [];
- for(let i = 0; i < array.length; ++i)
- array2[i] = array[i] * array[i];
when you can instead do the much more simple and idiomatic:
- let array = [1,2,3,4,5];
- let array2 = array.map(x => x * x);
Don’t try to be clever. Cleverness is poison. Clean, idiomatic, readable, maintainable code is the way to go.
Idioms are essentially “code memes”. They’re recognizable pat
Array prototype functions are not required at all to use arrays. You could be stubborn and insist on making your life harder and your code less maintainable by reinventing the wheel, but why do this:
- let array = [1,2,3,4,5];
- let array2 = [];
- for(let i = 0; i < array.length; ++i)
- array2[i] = array[i] * array[i];
when you can instead do the much more simple and idiomatic:
- let array = [1,2,3,4,5];
- let array2 = array.map(x => x * x);
Don’t try to be clever. Cleverness is poison. Clean, idiomatic, readable, maintainable code is the way to go.
Idioms are essentially “code memes”. They’re recognizable patterns that increase readability. Mostly by virtue of repeated use.
TL;DR - because what you think is clever big brain uber hacker code is actually garbage and your peers will hate you for it. Let’s see less of that and more of memes.
Define “better”?
I mean, I guess it depends on whether you have callbacks that capture references to those arrays, and you don’t want an already-scheduled callback to have stuff change from under it… this can lead to some really nasty bugs…
But if you’re writing a game or something, in-place and a for
loop is probably the way to go - the JIT compiler can optimize this better, you just have to make sure it’s correct.
Here is one reason.
If you access an array with an index, the run-time must first check whether the index is within the bounds of the array. And it has to do that for every single read and write.
If the runtime did not have this precaution, you could end up reading or writing outside the legal bounds of the array, and break stuff, over write code.
This frequent index-bounds-checking is expensive.
Map functions (etc) are guaranteed to be legitimate. There’s no possibilty for out-of-bounds addressing - so this costly step can be eliminated.
what are you talking about? those are easy. if you want simpler at least for arrays you can do this […arr]; the rest are just loops with different auto magical functions that would be annoying to do yourself.
Code sample using the reduce function:
- const myArrayOfObjects = [
- { myKey: 1, data: 'some data' },
- { myKey: 2, data: 'some data' },
- { myKey: 3, data: 'some data' },
- { myKey: 4, data: 'some data' },
- { myKey: 5, data: 'some data' },
- { myKey: 6, data: 'some data' },
- { myKey: 7, data: 'some data' },
- ];
- const myMap = myArrayOfObjects.reduce(
- (accumulator, currentObject) => ({
- ...accumulator,
- [currentObject.myKey]: currentObject,
- }),
- {}
- );
- console.log(myMap);
- // output:
- // {
- // '1': { myKey: 1, data: 'some data' },
- // '2': { myKey: 2, data: 'some data' },
- //
Code sample using the reduce function:
- const myArrayOfObjects = [
- { myKey: 1, data: 'some data' },
- { myKey: 2, data: 'some data' },
- { myKey: 3, data: 'some data' },
- { myKey: 4, data: 'some data' },
- { myKey: 5, data: 'some data' },
- { myKey: 6, data: 'some data' },
- { myKey: 7, data: 'some data' },
- ];
- const myMap = myArrayOfObjects.reduce(
- (accumulator, currentObject) => ({
- ...accumulator,
- [currentObject.myKey]: currentObject,
- }),
- {}
- );
- console.log(myMap);
- // output:
- // {
- // '1': { myKey: 1, data: 'some data' },
- // '2': { myKey: 2, data: 'some data' },
- // '3': { myKey: 3, data: 'some data' },
- // '4': { myKey: 4, data: 'some data' },
- // '5': { myKey: 5, data: 'some data' },
- // '6': { myKey: 6, data: 'some data' },
- // '7': { myKey: 7, data: 'some data' }
- // }
Repeated keys will be overwritten, so take into account that if you have repeated keys only the last one will remain.
The map(). filter() and reduce() are the functions which brings a shorthand approach to python programming. These functions could be replaced by list comprehensions or loops. All of these functions are releated to the lamda functions as they use function object as first argument, and this object could be from a pre-defined function like [def add(x, y)].
We’ll discuss these functions in brife but with complete knowldge about their functionality with an example for each:
- The map() Function : The map() function iterates through all items in the given iterable and executes the function we passed as
The map(). filter() and reduce() are the functions which brings a shorthand approach to python programming. These functions could be replaced by list comprehensions or loops. All of these functions are releated to the lamda functions as they use function object as first argument, and this object could be from a pre-defined function like [def add(x, y)].
We’ll discuss these functions in brife but with complete knowldge about their functionality with an example for each:
- The map() Function : The map() function iterates through all items in the given iterable and executes the function we passed as an argument on each of them, and the result will be in boolean where the condition gets staisfied ouput will be True else it will be False.
Syntax like :
- map(function, iterable(s))
with this function we can pass as many itretables to the functions we want. A peice of code to help you understand better:
- def findA(s):
- return s[0] == "A"
- fruit = ["Apple", "Peach", "Pear", "Apricot", "Lemon"]
- map_result = map(findA, fruit)
- print(list(map_result))
Output looks like:
- [True, False, False, True, False]
2. The filter() Function: Just like map() filter() function also take two arguments first one is a function and second is an itretables. Output consists of only the condition which is satisfied.
- def findA(s):
- return s[0] == "A"
- fruit = ["Apple", "Peach", "Pear", "Apricot", "Lemon"]
- map_result = map(findA, fruit)
- print(list(map_result))
Output looks like:
- ['Apple', 'Apricot']
Which is a shorter list compared to map()
3. The redue() Function: It does not return a new list based on the function and iterable we've passed. Instead, it returns a single value. But in python 3 reduce is not a inbuilt function you can import it from functools module.
reduce() works by calling the function we passed for the first two items in the sequence. The result returned by the function is used in another call to function alongside with the next (third in this case), element.
- from functools import reduce
- def add(x, y):
- return x + y
- list = [2, 5, 10, 1]
- print(reduce(add, list))
Output looks like:
- 18
JavaScript doesn’t have a “map” type, because it doesn’t need one. Every object is already a map from its attribute names to their values. You can do this:
- dict = {
- first: 'John',
- middle: 'Q',
- last: 'Smith'
- }
- console.log(dict.first) // will log John
- console.log(dict['first']) // same thing
- dict = new Object()
- dict['first'] = 'John'
- dict['middle'] = 'Q'
- dict.last = 'Smith'
- console.log(dict.first) // will log John
- console.log(dict['first']) // same thing
Objects map keys (names) to values, so you can look up or replace a value if you know its name.
Arrays are sequences of values in a particular ord
JavaScript doesn’t have a “map” type, because it doesn’t need one. Every object is already a map from its attribute names to their values. You can do this:
- dict = {
- first: 'John',
- middle: 'Q',
- last: 'Smith'
- }
- console.log(dict.first) // will log John
- console.log(dict['first']) // same thing
- dict = new Object()
- dict['first'] = 'John'
- dict['middle'] = 'Q'
- dict.last = 'Smith'
- console.log(dict.first) // will log John
- console.log(dict['first']) // same thing
Objects map keys (names) to values, so you can look up or replace a value if you know its name.
Arrays are sequences of values in a particular order, so you can look up or replace a value by its index.
Arrays are almost just objects where the keys are numbers starting from 0. However, you stick an extra prototype, Array
, into the chain ahead of Object
(or get that automatically when you use the […]
syntax), which means you get some extra array-specific methods.
- arr = [10, 20, 30]
- console.log(arr[1]) // will log 20
- arr = new Array()
- arr.push(10) // Don't have to specify that this is element 0
- arr.push(20) // or that this is element 1
- arr.push(30) // or that this is element 2
- console.log(arr[1]) // same again
- // Can't do this with a mapping; it wouldn't make sense
- console.log(arr.join('/')) // 10/20/30
In case of performance bottlenecks. i.e. .reduce() declares new variables with every run, .map() and .filter() creates a whole new arrays. It can cause garbage collector performance issues in case of long iterations.
Although there are lodash functions that solve such issues and still eliminate a need to use loops (i.e. you might use _.transform instead of _.reduce).
Have you tried running it?
It'll produce the array [7, 'ate', 9]
.
The filter() method creates a new array with only the elements that pass the test implemented by the callback.
So what happens behind the scenes is that we make a new empty array and iterate through the values in [7, "ate", "", false, 9]
. Each time we're running a test to see if that element should go into the result array. The test could be anything, but in this case it's just casting to boolean, ie it'll be true, and the value will get included, if and only if it counts as "truthy" in javascript.
An empty string isn't truthy, nei
Have you tried running it?
It'll produce the array [7, 'ate', 9]
.
The filter() method creates a new array with only the elements that pass the test implemented by the callback.
So what happens behind the scenes is that we make a new empty array and iterate through the values in [7, "ate", "", false, 9]
. Each time we're running a test to see if that element should go into the result array. The test could be anything, but in this case it's just casting to boolean, ie it'll be true, and the value will get included, if and only if it counts as "truthy" in javascript.
An empty string isn't truthy, neither of course is the boolean false. Those are the only elements which don't get included.
So that's why that's that.
As to why the bouncer() function is being passed a second parameter of a empty string... who knows, eh.
The choice of using a map or array in JavaScript depends on the specific use case. Maps are good for storing key-value pairs, while arrays are good for storing ordered collections of values.
In general, if you need to store key-value pairs and access them by key, a map is a better choice. If you need to store a large amount of data in a sorted order, an array is a better choice.
Here are some additional things to consider when choosing between a map and an array:
- The size of the data. If the data is small, an array may be a better choice, as it is easier to work with. If the data is large, a map
The choice of using a map or array in JavaScript depends on the specific use case. Maps are good for storing key-value pairs, while arrays are good for storing ordered collections of values.
In general, if you need to store key-value pairs and access them by key, a map is a better choice. If you need to store a large amount of data in a sorted order, an array is a better choice.
Here are some additional things to consider when choosing between a map and an array:
- The size of the data. If the data is small, an array may be a better choice, as it is easier to work with. If the data is large, a map may be a better choice, as it is more efficient.
- The need for iteration. If you need to iterate over the data, an array may be a better choice, as it is easier to do. If you only need to access elements by key, a map may be a better choice.
- The need for sorting. If you need to sort the data, an array may be a better choice, as it is easier to do. If you do not need to sort the data, a map may be a better choice.
Map, filter and reduce are “high-order functions”, which means a function that takes a function as an input argument or returns a function as output. Map, filter and reduce all take a function as an argument. This is a technique which originated in functional programming, but is now common in many languages.
Another reason these functions are “functional” is that they do sequence operations without any observable side effects. In imperative programming, you normally operate on the members of a sequence with a loop, which requires mutating values in place. In complex loops, state can potentially
Map, filter and reduce are “high-order functions”, which means a function that takes a function as an input argument or returns a function as output. Map, filter and reduce all take a function as an argument. This is a technique which originated in functional programming, but is now common in many languages.
Another reason these functions are “functional” is that they do sequence operations without any observable side effects. In imperative programming, you normally operate on the members of a sequence with a loop, which requires mutating values in place. In complex loops, state can potentially become difficult to keep track of. Factoring sequence operations through map, filter and reduce will often help produce a solution that requires fewer observable side effects and mutations. ideally, it means that sequence operations will become referentially transparent and hopefully easier to reason about—though I have seen some crazy uses of reduce
, which, though referentially transparent, were anything but easy to reason about.