Statically Typed
Of all the languages, Haskell is the "most functional": it's functional first and only layers imperative features on top of a solidly functional base. It's lazy and all effects have to be managed by the type system: normal code cannot work on mutable state or have any side effects whatsoever. It has a surprisingly expressive type system with no inheritance or sub-typing. Haskell has an incredible runtime system with top-notch parallelism and concurrency features including some very efficient green threads, software transactional memory and libraries for deterministic paralleli
Statically Typed
Of all the languages, Haskell is the "most functional": it's functional first and only layers imperative features on top of a solidly functional base. It's lazy and all effects have to be managed by the type system: normal code cannot work on mutable state or have any side effects whatsoever. It has a surprisingly expressive type system with no inheritance or sub-typing. Haskell has an incredible runtime system with top-notch parallelism and concurrency features including some very efficient green threads, software transactional memory and libraries for deterministic parallelism.
OCaml has some strong similarities to Haskell. It has similar algebraic data types and full type inference. It has a fast compiler that produces reasonably fast code. Since it's strict, many people find it easier to optimize than Haskell. Compared to the other functional languages I'm familiar with, it's probably the most memory efficient. At the same time, it has some really surprising drawbacks: a global interpreter lock heavily limits its concurrent capabilities, it has the weakest set of libraries of the lot with a very limited standard library and it's syntax is pretty ugly. OCaml supports some object oriented features with structural sub-typing and full type inference; however, most people don't like to use the OO subset of the language. Unlike Haskell, it has pervasive side-effects: mutable refernces, mutable arrays and even mutable strings!
Both OCaml and Haskell compile to native code, with reasonably easy interfaces to C. Interfacing with C++ is also possible but is a bit of a pain.
SML is very similar to OCaml, just generally more elegant. It's one of the very few fully specified languages along with Scheme. Unlike OCaml, it does not have any OO features. For better or for worse, it's been stagnating lately and is mostly used as a teaching language now.
Both OCaml and SML have very powerful module systems, something really missing from Haskell. Many advances in programming with modules and module typing come from SML and OCaml. To some extent, these module systems play the same role in program organization as OO classes do in OO languages.
Scala is much more of a compromise language than Haskell or OCaml. It can't really decide whether it wants to be functional or OO, so why not both? It suffers a lot from trying to be like Java, including unfortunate syntax, nominal sub-typing, limited type inference, no proper tail calls... etc. It has a capable OO system with classes, traits and nominal sub-typing. Being similar to Java and running on the JVM makes interoperation with Java libraries very easy. Since the design of Scala is full of compromises, it isn't very good for more advanced functional programming: read this detailed account by Edward Kmett about the problems they've had with Scala at S&P Capital IQ.
F# is Microsoft's .NET take on OCaml. It throws all of the exciting module features in order to be compatible with C♯ and .NET in general. It has nominal subtyping and more primitive type inference than OCaml et al. On the other hand, it has some really cool features like active patterns and type providers and, of course, integrates really well with the rest of the Microsoft stack. In practice, it feels much more comprable to OCaml than Scala.
Dynamically Typed
Erlang is a language with a very clear design goal: large, fault-tolerant distributed systems. And it does really well at this task, with lightweight process and an extremely resilient runtime system. Erlang is well-known for its let-it-fail philosophy to error handling: if you have a problem, just crash your process and replace with another one. Unfortunately, outside distributed systems, Erlang is quirky and relatively ugly language with odd syntax and surprising shortcomings. (They only had maps added recently!) I would not use it for anything involving non-trivial logic or really anything outside large distributed systems.
Scheme is a very elegant, minimalistic take on Lisp. It's designed to be small, self-contained and multiparadigm. While it supports and often encourages functional programming, it has imperative features at its very core. In practice, I've found code in Scheme is often at least partly imperative. A recent extension is Racket which provides additional libraries and features. Both Scheme and especially Racket are heavily geared towards developing DSLs, even more so than the other languages on this list. It's one of the first languages to include proper tail calls in the standard. Along with SML, it's the only fully specified language on this list.
Clojure is a Lisp for the JVM. It encourages functional programming, immutability and persistent data structures far more than Scheme or Racket. That said, it still has side effects and mutation at its core, it's just that common idioms and practices avoid them. Being on the JVM means it has some similar features to Scala: easy Java interoperability but some execution limits like no proper tail calls at all. It's the only language on this list apart from Haskell that has had any luck with software transactional memory.
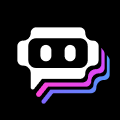
Modern functional programming languages, such as Haskell, Clojure, Scala, Erlang, and F#, each have unique characteristics, paradigms, and ecosystems. Here’s a comparison of their key differences:
1. Haskell
- Type System: Strongly typed with a powerful type inference system. It uses a purely functional programming paradigm.
- Evaluation Strategy: Uses lazy evaluation by default, meaning expressions are not evaluated until their values are needed.
- Syntax: Has a unique syntax that is often considered less approachable for newcomers.
- Ecosystem: Rich libraries for various domains, particularly in academia
Modern functional programming languages, such as Haskell, Clojure, Scala, Erlang, and F#, each have unique characteristics, paradigms, and ecosystems. Here’s a comparison of their key differences:
1. Haskell
- Type System: Strongly typed with a powerful type inference system. It uses a purely functional programming paradigm.
- Evaluation Strategy: Uses lazy evaluation by default, meaning expressions are not evaluated until their values are needed.
- Syntax: Has a unique syntax that is often considered less approachable for newcomers.
- Ecosystem: Rich libraries for various domains, particularly in academia and research.
- Concurrency: Supports concurrency through Software Transactional Memory (STM) and lightweight threads.
2. Clojure
- Type System: Dynamically typed, but supports optional type hints and has a rich set of data structures.
- Evaluation Strategy: Eager evaluation by default.
- Syntax: Based on Lisp syntax (S-expressions), which can be polarizing but offers powerful macro capabilities.
- Ecosystem: Runs on the Java Virtual Machine (JVM), allowing seamless integration with Java libraries and frameworks.
- Concurrency: Emphasizes immutability and provides features like agents, refs, and atoms for concurrent programming.
3. Scala
- Type System: Statically typed with type inference, allowing for both functional and object-oriented programming.
- Evaluation Strategy: Eager evaluation by default.
- Syntax: More Java-like syntax, which can be more familiar to Java developers, but still supports functional programming features.
- Ecosystem: Also runs on the JVM, making it interoperable with Java, with strong support for concurrent and distributed systems (e.g., Akka).
- Concurrency: Provides Futures and Promises for asynchronous programming and has robust libraries for parallel processing.
4. Erlang
- Type System: Dynamically typed with support for hot code swapping (updating code without stopping the system).
- Evaluation Strategy: Eager evaluation.
- Syntax: Prolog-like syntax, which can be unusual for those used to C-style languages.
- Ecosystem: Designed for building concurrent and distributed systems, particularly in telecommunications.
- Concurrency: Uses the Actor model for concurrency, emphasizing lightweight processes and message-passing.
5. F#
- Type System: Statically typed with a strong emphasis on type inference.
- Evaluation Strategy: Eager evaluation by default.
- Syntax: More similar to OCaml and ML languages, with a focus on concise and expressive syntax.
- Ecosystem: Runs on the .NET platform, allowing access to a wide range of libraries and tools.
- Concurrency: Supports asynchronous programming through async workflows and has good integration with .NET's Task Parallel Library.
Summary
- Purity vs. Pragmatism: Haskell is more focused on pure functional programming, while Scala and F# blend functional and object-oriented paradigms. Clojure emphasizes simplicity and immutability, while Erlang is specialized for concurrent systems.
- Type Systems: Haskell and F# are statically typed, while Clojure and Erlang are dynamically typed. Scala has a hybrid approach.
- Ecosystems: Clojure and Scala leverage the JVM, while F# integrates with .NET, and Erlang has its own runtime tailored for concurrent applications.
- Syntax and Learning Curve: Haskell and Clojure can be challenging for newcomers, while Scala and F# may be more accessible for those familiar with Java or C#.
Each of these languages offers distinct advantages depending on the application domain, developer preferences, and specific project requirements.
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
They are actually very different; the only thing they have in common is an emphasis on first-class functions and immutable data. There are a lot of details, but from a very high level:
- Haskell was a research experiment in whether or not a useful language could be completely pure. It is focused on strong, very expressive static typing, lazy evaluation and absolute purity. There is some debate as to how pure the IO monad is, but broadly speaking everyone agree it’s much purer than any other language out there.
- Clojure is focused on a uniform representation of data (i.e. the typical program defines
They are actually very different; the only thing they have in common is an emphasis on first-class functions and immutable data. There are a lot of details, but from a very high level:
- Haskell was a research experiment in whether or not a useful language could be completely pure. It is focused on strong, very expressive static typing, lazy evaluation and absolute purity. There is some debate as to how pure the IO monad is, but broadly speaking everyone agree it’s much purer than any other language out there.
- Clojure is focused on a uniform representation of data (i.e. the typical program defines very few new types and mostly just uses combinations of the standard library ones) and thread-safe mutation control mechanisms (as opposed to preventing mutation as Haskell does). It also has powerful metaprogramming facilities due to being a Lisp; other functional languages have some of that but it’s typically much harder to use.
- Scala is an experiment in mixing typical object-oriented (i.e. Java) concepts with typical functional (i.e. Haskell) ones, mostly at the type level. It has all the tools needed to write functional code, but no specific incentive to do so: it also has all the tools needed to write imperative code through and through. At some level it’s the answer to “how do you add Haskell-like features on top of Java, without removing anything from Java?”. The “without removing” part is what prevents me from considering it a functional language.
- Erlang has been designed for robustness; the core design goal of the language is to make programs that never stop running. Some part of the program may encounter an error, but the program as a whole should always be able to recover. In order to achieve that it has ended up with immutable data throughout, which qualifies it as a functional language. Its focus on reliability has pushed it to create a very interesting model of independent, concurrent “processes” (aka “actors” in other circles) that has later turned out to be great at parallelism (when multicore processors appeared). The Erlang creators had no interest in type theory; the language has a very small core of data structures and not much facilities for creating new types; it is dynamically typed.
I do not know F# well enough to give a good high-level description of the language goals.
A big part of the issue is that they are niche languages so “supply and demand” naturally leads to increased salaries for a smaller pool of developers.
In which case, why would companies even choose these “expensive” languages? In general, because they make certain things easier for smaller teams of developers and because developers are often happier using these languages than more mainstream ones.
Functional languages generally have immutable data at their core and are often built around a more consistent, higher-level of abstraction than mainstream languages.
These two aspects help you avoid a
A big part of the issue is that they are niche languages so “supply and demand” naturally leads to increased salaries for a smaller pool of developers.
In which case, why would companies even choose these “expensive” languages? In general, because they make certain things easier for smaller teams of developers and because developers are often happier using these languages than more mainstream ones.
Functional languages generally have immutable data at their core and are often built around a more consistent, higher-level of abstraction than mainstream languages.
These two aspects help you avoid a large class of bugs (those caused by mutability) and provide more “leverage”, allowing you to write more expressive code and therefore less code, which in turn means less bugs proportionally.
Where I work, we adopted Clojure back in 2011, and gradually rewrote all of our backend systems into Clojure as we needed to touch any existing code or add any new functionality. We’re able to manage a pretty large code base (large for Clojure — 140k lines) with just two developers at this point, and we’re able to turn features around very quickly. Our problem space happens to be very data-driven (online dating with a multi-tenant system that powers ~40 dating sites and apps) which suits Clojure very well, as it excels at data manipulation/transformation.
Matt's answer was pretty biased, but it's much better now :). However, it's obvious he prefers Scala. Consider the perspective of someone who loves to write F# and has done some Scala, but didn't enjoy it very much at all:
Technology stack - The latest editions of mono are quite fast. Very close to the MS CLR in fact. This is mostly due to a new garbage collector. Also, Oracle has shown it is willing to sue others who make small modifications to the stack. And let's not forget about that yahoo toolbar in every Windows install of the JVM. Microsoft on the other hand has been nothing but he
Matt's answer was pretty biased, but it's much better now :). However, it's obvious he prefers Scala. Consider the perspective of someone who loves to write F# and has done some Scala, but didn't enjoy it very much at all:
Technology stack - The latest editions of mono are quite fast. Very close to the MS CLR in fact. This is mostly due to a new garbage collector. Also, Oracle has shown it is willing to sue others who make small modifications to the stack. And let's not forget about that yahoo toolbar in every Windows install of the JVM. Microsoft on the other hand has been nothing but helpful to the Mono team and doesn't push crapware with their Mac Silverlight install.
Libraries - The libraries available for .NET tend to be much better designed than those for the JVM. This is partially due to .NET's much better implementation of generics and partially due to the fact that Microsoft has tools like fxcop. Also, .NET allows you to interface directly with COM, DLLs and C++ and so allows for very fast native implementations to be built and abstracted into nice managed interfaces.
Tools - F# has three well supported IDEs: Visual Studio 2010, Emacs (fsharp-mode) and MonoDevelop. In fact, some members of the F# team primarily use emacs. I've had the unfortunate experiences of using IntelliJ IDEA, Eclipse, and NetBeans in the past and hope very much that I'll never need to again. None of them even approaches what Visual Studio has to offer.
Type Inference - F# has fantastic type inference which greatly simplifies coding once you understand how it works. Scala has type inference on a similar level to C#: That is, only very simple. While new users to F# do get compilation errors due to type inference, this is actually a good thing. This is the compiler telling you that your code is incorrect. It's a safety feature. As they often say in ML, once you get the types right the program writes itself.
As functional languages - Scala is about as functional as Python. That is, it supports many of the constructs but isn't built around them. For example, Scala does not support true tail call optimization. I do agree that Scala is more object oriented and F# is more functional. However, I find my code ends up much more concise and readable when objects are used only occasionally. Perhaps this makes Scala more accessible, but it also locks users in to the old and broken object oriented model.
Tail Call Optimization - As a functional language, one of the biggest problems with Scala on the JVM is the lack of true tail call optimization. As Jon Harrop often points out, this means no fast or deep co-recursion, no untying the recursive knot and no continuation passing style. These are all important things in functional programming which you can't do in Scala on the JVM.
As object-oriented languages - Scala has a lot of object oriented features. Too many in fact. I've been neck deep in several Scala projects that heavily overused its multiple inheritance features and so were quite difficult to understand. F# has all of the standard features that C# has, plus some interesting things like anonymous objects based on interfaces with object expressions. It's just enough to play nicely with object oriented code but keep it out of your clean functional abstractions.
Syntax - Both F# and Scala allow you to define arbitrary operators from any combination of a special set of characters. Actually, none of the operators that come with F# are magic, they are all implemented as very simple library functions. You can see this if you just type (|>);; into the interpreter. You'll get: ('a -> ('a -> 'b) -> 'b). Internally this is implemented as: let inline (|>) x f = f x. They might seem complex at first, but there are just a handful of them. Once you get them down, it's completely unambiguous and way less typing.
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Mos
I once met a man who drove a modest Toyota Corolla, wore beat-up sneakers, and looked like he’d lived the same way for decades. But what really caught my attention was when he casually mentioned he was retired at 45 with more money than he could ever spend. I couldn’t help but ask, “How did you do it?”
He smiled and said, “The secret to saving money is knowing where to look for the waste—and car insurance is one of the easiest places to start.”
He then walked me through a few strategies that I’d never thought of before. Here’s what I learned:
1. Make insurance companies fight for your business
Most people just stick with the same insurer year after year, but that’s what the companies are counting on. This guy used tools like Coverage.com to compare rates every time his policy came up for renewal. It only took him a few minutes, and he said he’d saved hundreds each year by letting insurers compete for his business.
Click here to try Coverage.com and see how much you could save today.
2. Take advantage of safe driver programs
He mentioned that some companies reward good drivers with significant discounts. By signing up for a program that tracked his driving habits for just a month, he qualified for a lower rate. “It’s like a test where you already know the answers,” he joked.
You can find a list of insurance companies offering safe driver discounts here and start saving on your next policy.
3. Bundle your policies
He bundled his auto insurance with his home insurance and saved big. “Most companies will give you a discount if you combine your policies with them. It’s easy money,” he explained. If you haven’t bundled yet, ask your insurer what discounts they offer—or look for new ones that do.
4. Drop coverage you don’t need
He also emphasized reassessing coverage every year. If your car isn’t worth much anymore, it might be time to drop collision or comprehensive coverage. “You shouldn’t be paying more to insure the car than it’s worth,” he said.
5. Look for hidden fees or overpriced add-ons
One of his final tips was to avoid extras like roadside assistance, which can often be purchased elsewhere for less. “It’s those little fees you don’t think about that add up,” he warned.
The Secret? Stop Overpaying
The real “secret” isn’t about cutting corners—it’s about being proactive. Car insurance companies are counting on you to stay complacent, but with tools like Coverage.com and a little effort, you can make sure you’re only paying for what you need—and saving hundreds in the process.
If you’re ready to start saving, take a moment to:
- Compare rates now on Coverage.com
- Check if you qualify for safe driver discounts
- Reevaluate your coverage today
Saving money on auto insurance doesn’t have to be complicated—you just have to know where to look. If you'd like to support my work, feel free to use the links in this post—they help me continue creating valuable content.
Scala isn’t a “functional language”. It’s a generic language that can do functional stuff better than the previous generation.
My hunch is that Scala is going to lose ground to Swift and Kotlin in this space.
Haskell is the language to learn if you care about a) the more advanced theory of FP, and b) the most powerful features of type-systems. It’s also a pretty great language for a bunch real applications these days. It won’t hurt you at all to learn Haskell.
Lisp is a great language with a tonne of good ideas. Since forever. And you can’t go wrong learning a Lisp.
But I personally, and yes, this
Scala isn’t a “functional language”. It’s a generic language that can do functional stuff better than the previous generation.
My hunch is that Scala is going to lose ground to Swift and Kotlin in this space.
Haskell is the language to learn if you care about a) the more advanced theory of FP, and b) the most powerful features of type-systems. It’s also a pretty great language for a bunch real applications these days. It won’t hurt you at all to learn Haskell.
Lisp is a great language with a tonne of good ideas. Since forever. And you can’t go wrong learning a Lisp.
But I personally, and yes, this is my bias, would recommend Clojure which is both
- a) a Lisp (so has 90% of what’s good about any Lisp), and
- b) a proper hardcore functional programming language (ie. with immutability, default laziness for collections etc.) version of Lisp (unlike earlier Lisps which are mixed paradigm).
The other advantages of Clojure are that
- c) like Scala it’s designed to be compatible with the rest of the Java ecosystem, so you can immediately start doing real useful work with it in any context where you’d otherwise use Java
- d) Also, in the browser, ClojureScript with Reagent (a React wrapper) makes writing browser-based UIs very civilized indeed,
- e) is just a beautifully designed language in general. Clojure is as simple and “self evident” as a language like Python. Things generally just work as you’d expect (given the paradigm and the obvious quirks of Lisp syntax). But it’s also more powerful and elegant than Python (code to do the same thing is both shorter and clearer)
So I love Clojure. It’s not perfect. There are times it’s not appropriate. But still I rank it as the best language I’ve ever met. And I would recommend it to anyone who wants to both develop their programming knowledge, and have a useful tool to do real work in.
My background: I've dabbled with Haskell on and off since it appeared almost 25 years ago. I've dabbled with F# a little (I took a workshop on it a couple of years ago and I've attended a few meetups, unsessions, and conference talks about it). I built a small production system in Scala a few years back. I've been doing production Clojure for four years.
As other responses have indicated, if you really want to learn FP for its own sake and in its purest form, Haskell should be your first choice. It is absolutely the gold standard of FP languages at this point and what you learn there can easily
My background: I've dabbled with Haskell on and off since it appeared almost 25 years ago. I've dabbled with F# a little (I took a workshop on it a couple of years ago and I've attended a few meetups, unsessions, and conference talks about it). I built a small production system in Scala a few years back. I've been doing production Clojure for four years.
As other responses have indicated, if you really want to learn FP for its own sake and in its purest form, Haskell should be your first choice. It is absolutely the gold standard of FP languages at this point and what you learn there can easily be applied to any other languages you subsequently choose to learn. It will make you a better programmer. Period.
If you want to ease into FP alongside your current language of choice, then F# is a very good option. F# has a great pedigree -- coming from OCaml -- and you would be able to write parts of your system in F# and integrate that into your C# application very easily. If I worked primarily on a Windows platform, I would be a diehard F# fan, based on my exposure to it so far.
As noted by Reese Currie, Scala is a complex language, with a complex type system (that Odersky et al are working to simplify in the future) and it is also a hybrid OOP/FP language which means you can easily write non-FP code and miss some of the real benefits (and good concepts) of FP. I don't recommend it as a "first FP language" and only partially recommend it as a "better Java". Don't get me wrong: it's a very impressive and capable language but I think you need a solid FP background before you can use it effectively.
Finally, there's Clojure which is unique amongst the languages you list in two ways: it is the only dynamically typed language, and it is the only Lisp. I like it best for those two reasons but it's not for everyone. In the same way that Haskell will make you a better programmer, a Lisp will also make you a better programmer (in different ways, perhaps), because it is a very different idiom from "traditional" programming languages. Everything is data, and in Clojure it is all about the abstractions (sequences, protocols, etc). Clojure combines the best of OOP (several forms of a la carte polymorphism) with the best of FP (immutable data structures, a focus on small pure functions), as well as offering a pragmatic way to deal with mutable state (Software Transactional Memory) for practical, real-world FP. Clojure runs on the JVM, so the whole Java ecosystem is available to you (some view this as a negative point!), and it also targets JavaScript (via ClojureScript), and the CLR (via ClojureCLR) although the latter doesn't have as mature tooling and support as the other two platforms.
If you want to write better essays, it’s helpful to understand the criteria teachers use to score them. Instead of solely focusing on the grade you are given, focus on how you are being graded and how you can improve, even if you are already getting a high grade.
Development of Your Thesis
A thesis is the essence of your paper—the claim you are making, the point you are trying to prove. All the other paragraphs in your essay will revolve around this one central idea. Your thesis statement consists of the one or two sentences of your introduction that explain what your position on the topic at ha
If you want to write better essays, it’s helpful to understand the criteria teachers use to score them. Instead of solely focusing on the grade you are given, focus on how you are being graded and how you can improve, even if you are already getting a high grade.
Development of Your Thesis
A thesis is the essence of your paper—the claim you are making, the point you are trying to prove. All the other paragraphs in your essay will revolve around this one central idea. Your thesis statement consists of the one or two sentences of your introduction that explain what your position on the topic at hand is. Teachers will evaluate all your other paragraphs on how well they relate to or support this statement.
Strong Form
A good essay presents thoughts in a logical order. The format should be easy to follow. The introduction should flow naturally to the body paragraphs, and the conclusion should tie everything together. The best way to do this is to lay out the outline of your paper before you begin. After you finish your essay, review the form to see if thoughts progress naturally. Ensure your paragraphs and sentences are in a logical order, the transitions are smooth so that the paragraphs are coherently connected, and that your body paragraphs relate to the thesis statement.
Style
Just as your clothes express your personality, the style of your essay reveals your writing persona. You demonstrate your fluency by writing precise sentences that vary in form. A mature writer uses various types of sentences, idiomatic phrases, and demonstrates knowledge of genre-specific vocabulary, all the while ensuring the writing reflects your authentic voice.
Conventions
Conventions include spelling, punctuation, sentence structure, and grammar. Having lots of mistakes suggests carelessness and diminishes the credibility of your arguments. Furthermore, because most essays are written on computers these days, there is a lower tolerance for spelling mistakes, which can easily be avoided with spell-checking tools such as Grammarly. Beyond spelling, Grammarly can also help to weed out other major grammatical errors. Follow up with a close reading of your entire paper.
Support and References
Finally, your teacher will examine your resources. Select information from reliable websites, articles, and books. Use quotes and paraphrases to support your ideas, but be sure to credit your sources correctly. Also, always remember that copying five consecutive words or more from any source constitutes plagiarism. If you are concerned about unintentionally quoting your sources, Grammarly Pro offers a plagiarism detector so you can always double-check your work.
The grades you get on your essays are important, but you can never improve your writing if they are the only things you consider. Focus on improving your essays’ overall structure—the thesis development, form, style, conventions, and support. Learning to master these five elements will cause your scores to soar!
I’m with Gerry Taylor in saying that Golang doesn’t belong on this list. Go is the opposite of exotic — as a language, it’s basic. (Its compiler speed is noteworthy, but that’s somewhat outside the scope of the language itself.)
Why are languages, like Scala, Haskell, Erlang, and Golang, considered exotic languages?
The reason that [some programmers] regard Scala, Haskell, and Erlang as exotic is that each of them breaks the only conceptual model of interacting with a computer that these programmers know: the do-this-then-do-that-then-do-the-other-thing model known as imperative programming.
The
I’m with Gerry Taylor in saying that Golang doesn’t belong on this list. Go is the opposite of exotic — as a language, it’s basic. (Its compiler speed is noteworthy, but that’s somewhat outside the scope of the language itself.)
Why are languages, like Scala, Haskell, Erlang, and Golang, considered exotic languages?
The reason that [some programmers] regard Scala, Haskell, and Erlang as exotic is that each of them breaks the only conceptual model of interacting with a computer that these programmers know: the do-this-then-do-that-then-do-the-other-thing model known as imperative programming.
The languages people learn in school or college CS classes, or that they pick up teaching themselves, or that they learn in bootcamp, are with high probability imperative languages. They may or may not also support object-orientation (C does not; Java does; Go does not; …), but the basic structure of a block of code in all is do-this-then-do-that-then-if-we’re-in-this-condition-do-the-other-thing-else-do-the-other-other-thing.
The three languages in the question either do not support an imperative style, or else it is strongly unidiomatic.
Haskell
Haskell, for starters, is entirely declarative — there are some language constructs like the do
-notation syntactic sugar over monadic binds that can feel a bit imperative (since monads are the idiomatic pattern to sequence effectful operations), but experienced Haskellers learn to read them as declarative as well (since an unreferenced value produced by a monadic bind, like everything else in Haskell, will never be instantiated due to laziness).
Take an example like mergesort. This algorithm is pretty easy to express in imperative terms:
Take the sequence you want to sort. Cut it in halves. Recursively sort each half. Then allocate a target sequence of the correct length and pop elements off the front of the sorted halves and push onto the target sequence until both sorted halves are empty.
There are, as usual, a lot of implementation details to get wrong when translating this sequence of human-language instructions into computer language, but that’s not my purpose here. Instead, I want to illustrate how Haskell would express this algorithm entirely declaratively:
- merge :: (Ord t) => [t] -> [t] -> [t]
- merge [] ts = ts
- merge ts [] = ts
- merge (a : as) (b : bs)
- | a <= b = a : (merge as (b : bs))
- | otherwise = b : (merge (a : as) bs)
- mergesort :: (Ord t) => [t] -> [t]
- mergesort [] = []
- mergesort (t : []) = t : []
- mergesort ts =
- let (lefts, rights) = splitAt (div (length ts) 2) ts
- in merge (mergesort lefts) (mergesort rights)
I don’t even write Haskell and I could produce this in a few minutes (most of which was looking up partially remembered syntax). But if you’re coming from C or Java or Python, it’s likely that even trying to read this makes your head hurt — that’s a perfectly normal reaction. It’s not just unfamiliar syntax hiding familiar operations, it’s a necessarily unfamiliar syntax expressing an unfamiliar way of even thinking about programs.
(Note also: this is not a production-ready mergesort, since merge
is not tail-recursive and will self-call length ts
times. This can be avoided, but it would make the example even harder to read.)
Erlang
Erlang does something similar, but very different. It upends the familiar way of structuring a program’s control flow, but not by turning everything into an expression — instead, Erlang leans hard into the Single Responsibility Principle in the sense that if an object needs something done that’s someone else’s responsibility, you send a message to that other object telling them what they need to do and relinquish control flow. Where in traditional imperative programming one would block until the other object finished its part, in Erlang you have to architect the system in such a way that once Object B finishes its part, it knows who to send the job on to next to continue processing.
In a sense, this moves threads of execution onto the heap — the underlying runtime is in charge of scheduling all the objects onto system threads, but there is no one-to-one relationship between conceptual threads of execution and system resources.
What this means for the programmer working in Erlang is, there’s no more do-this-then-call-service-a-to-do-that-then-do-the-other-thing-then-call-service-b-to-do-the-other-other-thing. There is only
- execute my single responsibility; then
- pass the thread along to the next guy and relinquish control.
Scala
Programming in Scala can be a strange hybrid beast, because it enables all three of the herein described patterns/paradigms. (Full disclosure: I write Scala for my day job, and I love it.) You absolutely can write straight-up imperative code in Scala — but if you’re on a professional software team, your teammates will not find this amenable and will point you to the team code standards document.
Scala is designed to support declarative syntax, and the mergesort example above translates readily into Scala (using parentheses and dotted member access instead of whitespace):
- def mergeSort[T: Ordering](ts: List[T]): List[T] = {
- def merge(lefts: List[T], rights: List[T]): List[T] = (as, bs) match {
- case (Nil, _) => rights
- case (_, Nil) => lefts
- case (a :: as, b :: bs) if a <= b =>
- a :: merge(as, rights)
- case (a :: as, b :: bs) =>
- b :: merge(lefts, bs)
- }
- ts match {
- case Nil | (_ :: Nil) => ts
- case _ =>
- val (lefts, rights) = ts.splitAt(ts.len/2)
- merge(mergeSort(lefts), mergeSort(rights))
- }
- }
But Scala also supports an Erlang-style actor-based message passing architecture, enabled via the Akka framework. The language is sufficiently flexible that the Akka library writers could build in Erlang’s “send an object a message” primitive via a domain-specific language; they have since continued to expand the library to support type-safe messaging (where Erlang, inspired by Smalltalk, supports messaging arbitrary values to any receiver).
I’ve heard complaints that Scala is a “kitchen-sink” language, because it enables all these patterns. I haven’t found this to be true in practice. Most small applications don’t benefit from an actor model in the first place; those that do still need the internal logic of each actor — the code that fulfills that actor’s single responsibility — to be written in readable idiomatic Scala. Truly imperative code (that is, functions or blocks that do more than bind some local variables and then do one thing with them) is frowned upon (because it’s harder to debug and maintain). The key is to have a team of people who agree on a style and a standard (and who can identify the problems that the choices in the style guide are meant to prevent) and are committed to a readable, maintainable project.
Scala is evolution not revolution; it has maximum back-compatibility with Java. Haskell is the opposite. Clojure is Lisp.
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off
With today’s modern day tools there can be an overwhelming amount of tools to choose from to build your own website. It’s important to keep in mind these considerations when deciding on which is the right fit for you including ease of use, SEO controls, high performance hosting, flexible content management tools and scalability. Webflow allows you to build with the power of code — without writing any.
You can take control of HTML5, CSS3, and JavaScript in a completely visual canvas — and let Webflow translate your design into clean, semantic code that’s ready to publish to the web, or hand off to developers.
If you prefer more customization you can also expand the power of Webflow by adding custom code on the page, in the <head>, or before the </head> of any page.
Trusted by over 60,000+ freelancers and agencies, explore Webflow features including:
- Designer: The power of CSS, HTML, and Javascript in a visual canvas.
- CMS: Define your own content structure, and design with real data.
- Interactions: Build websites interactions and animations visually.
- SEO: Optimize your website with controls, hosting and flexible tools.
- Hosting: Set up lightning-fast managed hosting in just a few clicks.
- Grid: Build smart, responsive, CSS grid-powered layouts in Webflow visually.
Discover why our global customers love and use Webflow.com | Create a custom website.
The best way to sum up the advantages is “you get more done with less work.” For example, given (approximately) the number of lines of Elixir code to do the same amount of work in Go or Java, you will write 4x as much Go or Java code. But you also spend less time debugging because immutable values, lack of inheritance, collection-oriented functions and (depending on the language) type-checking all eliminate bug-creation.
Shout out to Erlang/Elixir pattern-matching. It is weird at first, coming from OOP, but soon becomes indispensable. And eliminates a bunch of logic. Which means (surprise) fewe
The best way to sum up the advantages is “you get more done with less work.” For example, given (approximately) the number of lines of Elixir code to do the same amount of work in Go or Java, you will write 4x as much Go or Java code. But you also spend less time debugging because immutable values, lack of inheritance, collection-oriented functions and (depending on the language) type-checking all eliminate bug-creation.
Shout out to Erlang/Elixir pattern-matching. It is weird at first, coming from OOP, but soon becomes indispensable. And eliminates a bunch of logic. Which means (surprise) fewer bugs.
So if you want to accomplish more with less code and fewer bugs, go functional. I took to the BEAM VM over the Java VM, so I went Elixir.
I can speak only of Haskell vs Erlang, and only about hobbyists, because for professional developers the overwhelming reasons of A vs B are as mundane as:
* The management forces you to use A
* There is an offer (a job or a project) for A
For managers and technical leadership the reasons are slightly different but there are similarly “absurd” reasons you probably didn’t want to hear when you aske
I can speak only of Haskell vs Erlang, and only about hobbyists, because for professional developers the overwhelming reasons of A vs B are as mundane as:
* The management forces you to use A
* There is an offer (a job or a project) for A
For managers and technical leadership the reasons are slightly different but there are similarly “absurd” reasons you probably didn’t want to hear when you asked this question:
* The customer forces you to use A
* The competitors use A so nobody would blame you for choosing A
* There is a team for A
* The company has an inhouse libraries, project templates and workflows/processes for A
* A has a “killer” library relevant for the particular project
You see, there are things programmers think they do, and the things they actually do. People imagine that they do algorithms, problem solving and algebra, but in my project we are currently discussing about enabling some previously disabled functions by adding items to an already implemented whitelist. The developer who will amend the whitelist won’t do any of the above, any junior able to find the whitelist in the codebase can do it, no fancy knowledge is involved on his side as the management figured out the solution and the solution is dumb simple.
So, back to the choice of languages, the fancy process of actually comparing languages for their flaws and advantages and using whatever turned out better in the review, is not what actually happens. Of course, people do actually use algebra, there are even people doing it every day ten times before lunch, but it’s not tr...
“Is Haskell easier to learn than other popular functional languages like Scala or Clojure?”
Haskell should be easier to learn. Scala is a very complex language. I had no end of trouble with Clojure, in particular trying to understand the namespaces.
Haskell is a simple language. Unfortunately, far too many tutorials are unreadable, because at some time the author decided to show off their Haskell skills, rather than helping other people to improve their skills.
Hence all too often, Haskell feels like an exercise in attrition - you versus the machine.
In terms of language features?
CL is like dynamically typed C++ — it’s old and encompasses everything. Imperative features, higher-order functions, (unhygienic) macros, signals system (like exceptions but more advanced) and the most advanced and complex object system (with multiple inheritance and multimethods plus Meta-Object Protocol). That’s why it, much like C++, is rather cumbersome to use in practice.
That’s also why more recent Lisp dialects like Clojure cherry-pick just a subset of its features that are more coherent and easier to use. Plus Clojure has first-class principled support for
In terms of language features?
CL is like dynamically typed C++ — it’s old and encompasses everything. Imperative features, higher-order functions, (unhygienic) macros, signals system (like exceptions but more advanced) and the most advanced and complex object system (with multiple inheritance and multimethods plus Meta-Object Protocol). That’s why it, much like C++, is rather cumbersome to use in practice.
That’s also why more recent Lisp dialects like Clojure cherry-pick just a subset of its features that are more coherent and easier to use. Plus Clojure has first-class principled support for concurrency which was not an issue at the time of CL inception.
And Haskell is nothing like that, nothing like that at all. Just don’t even try to compare Haskell to Lisps or other dynamically typed functional languages. It’s two completely different kinds of “functional programming”. Almost all of Haskell features are about types (ADT, GADT, higher-kinded types, rank-n types, existential types, type families and so on). And most of its abstractions are Algebra-based and type-driven. You have almost nothing like that in dynamic languages.
The most important way in which Haskell is different from other widely know functional languages is that it uses lazy evaluation (values are only computed when they are required by another computation) rather than the more common “eager” or “strict” evaluation strategy where expressions are evaluated immediately.
A side effect of lazy evaluation is that there’s no obvious way to perform effects like printing to the terminal, communicating over a socket or even changing a value in an array. What is the value of the expression print("hello, world.")
? When is this value required? In most languages
The most important way in which Haskell is different from other widely know functional languages is that it uses lazy evaluation (values are only computed when they are required by another computation) rather than the more common “eager” or “strict” evaluation strategy where expressions are evaluated immediately.
A side effect of lazy evaluation is that there’s no obvious way to perform effects like printing to the terminal, communicating over a socket or even changing a value in an array. What is the value of the expression print("hello, world.")
? When is this value required? In most languages, this is a nonsense question. The print function returns void or in some languages it returns a nil value intended to be discarded (in some languages it returns the number of bytes printed, but this value, too, is often discarded).
In the context of laziness, if the value is never needed, the expression doesn’t execute. Without going into too much detail, Haskell’s solution is that effects produce a value, and the effect doesn’t actually happen until you unwrap the value by chaining effects together in a sequence of callbacks which invoke some runtime magic to perform the effect (this is what the fuss over monads is about).
This may sound like a limitation in the language and that is true—the positive side is that this limitation encourages separating your computational core from the parts of the code that do effects—something you should be doing in every language anyway.
Most popular functional languages use eager evaluation and effects just happen in order wherever they appear in the code without any special unwrapping required
Another way in which Haskell is different from some functional languages is that it has a types system from the ML tradition, like SML, OCaml, F#, Scala and some others. Rust also has such a type system, though it is not functional.
In this system, types are conceived of as sets of values, where new types can be defined in terms of products or unions of other sets of values. Because the compiler knows every value which belongs to every type, pattern matching (a kind of fancy switch case) can be used to ensure every possible case has been matched.
In practical terms, this means you’ll have an explicit code path for every possible program or function input. This can drastically decrease the number of possible fatal errors at runtime, even eliminating them entirely in most cases (aside from cases like running out of memory)
Just to throw in my 2-bits, I wanted to reacquaint myself with functional programming several years back and looked at Scala and F#, among other languages.I went with F# because object-oriented development is secondary and have used it regularly for the last 2+ years. I disagree with Matt that extensive object-oriented support is beneficial. Here's my thinking...
For "gently" diving in to functional programming, pick whatever language works with your regular environment -- Scala on JVM, F# or C# on .NET, or even Python and, if you really want, C++. But I think this is a mistake.
IMHO the real b
Just to throw in my 2-bits, I wanted to reacquaint myself with functional programming several years back and looked at Scala and F#, among other languages.I went with F# because object-oriented development is secondary and have used it regularly for the last 2+ years. I disagree with Matt that extensive object-oriented support is beneficial. Here's my thinking...
For "gently" diving in to functional programming, pick whatever language works with your regular environment -- Scala on JVM, F# or C# on .NET, or even Python and, if you really want, C++. But I think this is a mistake.
IMHO the real benefit to learning a "functional language" is learning to "think" functionally -- data is immutable, functions are first-class elements and applied TO data opposed to being bound together with data, and so on. Reading Chris Okasaki's book Purely Functional Data Structures was the eye-opener for me. For making this mental switch, object-oriented support is useful for utilizing existing libraries, but can end up being a crutch. If one is using features like multiple inheritance, again IMHO, the mental switch hasn't been made.
All that said, I think the choice of language is much less important than learning a new way to think and look at a problem. It's a good skill to have and opens up alternative solutions to many problems.
As languages facilitating functional programming, they are actually quite similar. They're both strictly evaluated "object-oriented" languages, with two very important differences:
F# has better type inference. Scala's type system is more complex, hence inference is more difficult to implement. In Scala, inference generally flows pretty well from arguments to results, but not so well from results to arguments. F# also has better metaprogramming facilities (so far).
Scala has higher-kinded types. Also known as type-constructor polymorphism, or higher-order generics. This is an absolutely indispen
As languages facilitating functional programming, they are actually quite similar. They're both strictly evaluated "object-oriented" languages, with two very important differences:
F# has better type inference. Scala's type system is more complex, hence inference is more difficult to implement. In Scala, inference generally flows pretty well from arguments to results, but not so well from results to arguments. F# also has better metaprogramming facilities (so far).
Scala has higher-kinded types. Also known as type-constructor polymorphism, or higher-order generics. This is an absolutely indispensable feature in my opinion. Whereas in Scala you can write libraries that use type constructors (such as monads) parametrically, the only way to do this in F# is with code duplication or code generation.
However, OCaml (another ML variant like F#) has recently added type constructor polymorphism, so we may see this in F# at some point.
Common Lisp isn't limited to being purely functional, although it does permit it. CL is mature, has amazing debugging and development features, is quite efficient, and has been ported to many environments natively, as well as running on the JVM (and work in progress for JavaScript).
Haskell is mature, has fair debugging features, is quite efficient, purely functional, and runs natively on a few environments.
Clojure is immature, and in my opinion has serious flaws in its implemetation which require a deep understanding of both Clojure quirks and those of the underlying environment (Java, or Java
Common Lisp isn't limited to being purely functional, although it does permit it. CL is mature, has amazing debugging and development features, is quite efficient, and has been ported to many environments natively, as well as running on the JVM (and work in progress for JavaScript).
Haskell is mature, has fair debugging features, is quite efficient, purely functional, and runs natively on a few environments.
Clojure is immature, and in my opinion has serious flaws in its implemetation which require a deep understanding of both Clojure quirks and those of the underlying environment (Java, or JavaScript), is nightmarish to debug, and runs only on the most popular virtual machines.
NB “nightmarish to debug” by comparison to eg, Common Lisp or native JavaScript, not perhaps as compared to, say, Assembly language.
Francis King has provided a nice answer. I’m not sure I’d call Kotlin a functional language—though perhaps it is “more functional” than Java, but it lacks functional data structures in the standard library. It is a nice language for the JVM in any event. He otherwise mentions Clojure, Erlang and Elixir—all fine languages.
I add some additions:
- OCaml is a direct predecessor of both Scala and F#, and a cousin of Haskell. It still holds its own as a statically typed functional language, especially for programmers on a Unix-like platform. It’s known for balancing theoretical soundness and an advance
Francis King has provided a nice answer. I’m not sure I’d call Kotlin a functional language—though perhaps it is “more functional” than Java, but it lacks functional data structures in the standard library. It is a nice language for the JVM in any event. He otherwise mentions Clojure, Erlang and Elixir—all fine languages.
I add some additions:
- OCaml is a direct predecessor of both Scala and F#, and a cousin of Haskell. It still holds its own as a statically typed functional language, especially for programmers on a Unix-like platform. It’s known for balancing theoretical soundness and an advanced type system with pragmatic features for real-world applications. It also supports classical OO—and is actually one of the best implementations I’ve seen with thoroughgoing structural typing, though it isn’t used much in practice.
- Standard ML is more or less the sister language to OCaml. It perhaps has a greater emphasis on purity than OCaml or F#, though not to the same extent as Haskell. It lacks any OO features, which many people see as a good, but it also lacks many of the other goodies of OCaml (GADTs, polymorphic variants, first-class modules, etc.)
- Racket is a dialect of Lisp. It could almost be consider a non-standard Scheme implementation with many extensions and quality-of-life features—and indeed, it uses the Chez Scheme compiler as a backend. Racket’s primary usecase is as a platform for prototyping new programming language.
- Common Lisp is a standardized definition of the Lisp language. It has MANY features and, though dynamically typed, leading implementations like Allegro and SBCL can produce incredibly well-performing code.
I think, aside from those mentioned in the question and those mentioned by Francis in his answer, these are the major ones. However, there are many other functional languages which might be useful for various niches.
PureScript, Elm, and ReScript are statically typed functional languages which compile to JavaScript for use in the web browser or with Node.js. PureScript is a purely functional language which is very similar to Haskell. Elm is also inspired by Haskell, but a bit farther away. Elm comes with its own frontend framework for purely functional DOM interaction. ReScript is a language inspired by (and originally forked from) OCaml which has great bindings for working with React.
Agda, Gallina, Idris and ATS are dependently typed languages in which formal proofs of correctness can be constructed for functions. Agda and Gallina are more specifically for theorem proving applications (Gallina is specifically for specifying proofs in the Coq theorem prover). Idris and ATS have more general programming goals in mind. Idris looks similar to Haskell and is the more user friendly of the two. ATS looks more like ML and is too complicated for me to grok, but has performance comparable to C++.
Futhark is a statically typed, purely functional language for writing parallel code that runs on CUDA, OpenCL or multi-threaded processors. It’s great for advanced vector mathematics, and it compiles to object code which can be called from other languages.
There are many other functional languages. Clean is another one that comes to mind. It’s lazy and pure like Haskell, but has an entirely different system for dealing with effects.
Haskell, as a general rule, is best to test your knowledge of FP concepts. The language has advanced features that enforce things are done in an FP style ie pure functions.
Haskell, in production, is best for web-servers and backend development, as well as academic research.
Scala runs on the JVM and so has interop with Java libraries and language. The language supports both OOP and FP paradigms. The styles can be easily mixed, and therefore it is hard to define a specific paradigm. Scala does not enforce an FP style ie pure functions.
Scala is best at backend development but can run almost anywh
Haskell, as a general rule, is best to test your knowledge of FP concepts. The language has advanced features that enforce things are done in an FP style ie pure functions.
Haskell, in production, is best for web-servers and backend development, as well as academic research.
Scala runs on the JVM and so has interop with Java libraries and language. The language supports both OOP and FP paradigms. The styles can be easily mixed, and therefore it is hard to define a specific paradigm. Scala does not enforce an FP style ie pure functions.
Scala is best at backend development but can run almost anywhere the JVM is. One distinct difference of Scala over Haskell is the that has access to all of the Java’s libraries, so if there is not Scala library, a Java one can be used instead.
F# runs on .NET and is a functional first language. The language paradigm encourages an FP style but supports an OOP style. F# is a great language for learning FP, as the laziest/shortest implementation in F# is generally a functional one. F# does not enforce FP style specifically pure functions.
F# has full interop with C#, so if a library is not available in F#, a C# one can be used.
F# has seen a lot of success on the backend, though the language can also do front-end development with the fable compiler and react. The language has tools for data-science, and can even call into the R programming language through a feature called the type-providers. F#, through the use of Xamarin, can be used to build a native app iOS, Android, UWP (Windows 10) and Linux. F# also supports windows desktop development using WPF.
Also .NET now runs on any platform including Mac and Linux. Visual Studio is now available on Mac too.
I prefer Haskell for learning FP, F# for production use. F# is functional to avoid bugs but pragmatic enough to ship quality code on any platform.
Simple answer, they are not "pure" functional languages. Assuming your question implying why we have languages that use functional elements which are not so niche (i.e. mainstream), its because of a number of reasons..
1. As stated before, they aren't "pure" functional languages. Scala mixes imperative and OO (which deals with modules, packaging, namespaces etc etc) with functional elements. Clojure is likewise similar (although clojure has its own definition of OO (i.e. multilevel dispatch), it has added a lot of stuff to the standard LISP)
2. They made compromises to leverage existing ecosyst
Simple answer, they are not "pure" functional languages. Assuming your question implying why we have languages that use functional elements which are not so niche (i.e. mainstream), its because of a number of reasons..
1. As stated before, they aren't "pure" functional languages. Scala mixes imperative and OO (which deals with modules, packaging, namespaces etc etc) with functional elements. Clojure is likewise similar (although clojure has its own definition of OO (i.e. multilevel dispatch), it has added a lot of stuff to the standard LISP)
2. They made compromises to leverage existing ecosystems. Both run on JVM, and both have very good interopt with Java libraries.
3. Sought of in tangent with #1 and #2, both designers of the language (and hence the languages themselves) have a more pragmatic rather than theoretical approach. Both languages clearly admit the merits of functional programming, however admit at the same time that FP isn't the solution to everything (for a wide variety of reasons)
4. Both languages use the FP paradigm to solve problems that are currently very relevant, and play to FP's biggest strength, i.e. distributed programming with explicit management of minimal (or even stateless) systems. This is mainly in the backend, web server space. As a counter example, you will notice that languages like Haskell have attempted to "solve" IO in many different ways (on the basic level with Monads, and then using concepts like arrows and FRP) which haven't really caught on, even though they have been researched for a long time.
They have a cult among programmers that DON’T use them regularly. People who do tend to be moderate as they have already learned the downsides.
As an anecdote, I only know an Erlang fan who can deliver systems in Erlang. I also doubt F# fans exist because it’s so second-class language on dotnet.
The most obvious benefit is the bragging rights and the feeling of exclusivity and being different.
The se
They have a cult among programmers that DON’T use them regularly. People who do tend to be moderate as they have already learned the downsides.
As an anecdote, I only know an Erlang fan who can deliver systems in Erlang. I also doubt F# fans exist because it’s so second-class language on dotnet.
The most obvious benefit is the bragging rights and the feeling of exclusivity and being different.
The second benefit is the talent retention.
They have a cult following because they allow for abstraction the ways mainstream languages don’t, so it’s possible to write interesting code in it and solve complex problems elegantly.
Unfortunately, this is not...
For practical purposes, Scala easily beats Haskell. It's compatible with Java, it's pluggable, it will work on any JVM, including your Android.
For more "scientific" purpose, Haskell is more pure, and, regarding types handling, definitely more correct. If you know Haskell, you can write tiny little apps that are amazingly efficient.
But somehow almost all Haskell people I know have to write in Java or C++ while the sun is up.
On the other hand, Martin said today, that Scala should be renamed to Haskellator, being a gateway drug for Haskell.
Not sure what you’d consider a traditional programming language, but all those languages that you mention here are functional (or also functional). This makes them more flexible and expressive ― as a case in point, imagine that you can pass functions as arguments to functions ― how flexible this can make your code and designs, and how better it may mirror your thoughts, if you’ve not been brain-washed too much into totalitarian object oriented paradigms.
The more mathematically inclined you are, this would be more enticing to you. This stands in stark contrast to languages like Java, which are
Not sure what you’d consider a traditional programming language, but all those languages that you mention here are functional (or also functional). This makes them more flexible and expressive ― as a case in point, imagine that you can pass functions as arguments to functions ― how flexible this can make your code and designs, and how better it may mirror your thoughts, if you’ve not been brain-washed too much into totalitarian object oriented paradigms.
The more mathematically inclined you are, this would be more enticing to you. This stands in stark contrast to languages like Java, which are not functional, yet have been very aggressively popularized for several decades. Even in Java 8 and 9 you cannot properly practice functional programming, and you are still encouraged to model everything through object metaphors, and if it doesn’t fit ― force it harder, which is many times misaligned with the nature of the problem at hand.
Another advantage is that most these languages encourage immutable values and data, which make your CPU work a bit harder but reduces the chances of you writing code that blows up under concurrency.
Some of the languages you mention have additional advantages ― mainly in areas like concurrency and scalability ― where they offer safe building blocks for concurrency (mainly Erlang, Elixir and Clojure, but much less so truthfully scala).
Of course these languages also have downsides, i.e. you might be too young or old to adapt, and of course they tend to have a lesser footprint in the commercial developer community (except maybe Erlang in Telco). Some of them sport less ready-made and well supported libraries to choose from. With scala you also have backward compatibility issues between versions of the languages and such additional earth-like considerations you’d want to explore before making very strategic choices. Also you need to know when to use mutable data and a bit of OO design even when using these languages, depending on the nature of what it is that you need to accomplish.
Don’t believe what a lot of the other answers are saying: functional programming languages are hard. People say “they are not hard, it just requires a different way of thinking”, well getting your head around a different way of thinking is hard almost by definition. Some of the concepts involved in FP can be really difficult to get your head round but then once you do, it suddenly becomes beautifully elegant and simple and you forget the pain you went through.
With that in mind, Scala is a nice half way house from Java to hardcore functional programming. You can view it as a not quite full blow
Don’t believe what a lot of the other answers are saying: functional programming languages are hard. People say “they are not hard, it just requires a different way of thinking”, well getting your head around a different way of thinking is hard almost by definition. Some of the concepts involved in FP can be really difficult to get your head round but then once you do, it suddenly becomes beautifully elegant and simple and you forget the pain you went through.
With that in mind, Scala is a nice half way house from Java to hardcore functional programming. You can view it as a not quite full blown FPL or a better Java.
However, the main reason for its popularity, in my opinion is that it is a drop in replacement for Java. You an even run Java and Scala in the same JVM and they can talk to each other natively. This means you can leverage all of the massive Java ecosystem with Scala.
There’s also the Play framework which is to Scala as Rails is to Ruby or Django is to Python and although it works best with Scala, Play integrates nicely with Java too for the reasons given above.
Functional languages
- help you write code which is easy to reason about
- achieves Referential Transparency though the use of immutable data structures
- are best for parallel programming
- are more declarative (What need to done instead of How)
- do not mess with Time (as entity)
- has lot less incidental complexity (compared to OO) and hence help you attack at the problem statement directly
The problem with a question like this, is that the answer is going to be so subjective its utility will be based almost entirely on the perceived authority of the person answering, no?
My experience of "why people choose Scala" has almost always been that they were already using Java and the transition was seen as a natural evolution, and into a language that for better or worse is seen as "Enterprise" by people making decisions without actually being at the coalface of software development. That's not to say that Scala is bad, or even not as good as Clojure or Erlang, just that it has the gre
The problem with a question like this, is that the answer is going to be so subjective its utility will be based almost entirely on the perceived authority of the person answering, no?
My experience of "why people choose Scala" has almost always been that they were already using Java and the transition was seen as a natural evolution, and into a language that for better or worse is seen as "Enterprise" by people making decisions without actually being at the coalface of software development. That's not to say that Scala is bad, or even not as good as Clojure or Erlang, just that it has the greater momentum in the wider sphere of the IT world. Scala does appear, for what it may be worth, to be vey well suited to the kind of message queue handling and event-based system creation that is characterised by large, high-availability, connected systems, and this would be bourn out by looking at how Twitter used Scala to scale their "streams".
I can't comment with any authority on Erlang, except to say that its origins do seem to speak volumes as to where it might be most useful - telecommunications, and so to boil it down to brass tacks, message passing, transmission, receipt etc. The fail early, fail often, without stopping (?) approach to technical design that Erlang empowers is a powerful proposition - if you have lots and lots of throughput and you need to "stay up" regardless of what may happen, then by all accounts you could do worse than choose Erlang.
Clojure is, if I may borrow the words of a wiser man, the programming environment / toolset most able to mitigate risk in software development endeavours. The features of the language when coupled with REPL-driven development and the ease of testing a functional language that also imposes immutable state, can lead to a great deal of productivity with greatly reduced risk or uncertainty, if done well at any rate.
The answer to the question, really, is there is more at stake than the language that you choose. How available are people with the knowledge and skills that you will need to adopt the tools that you want to use? How compatible with your use-case(s) are the existing toolchain for the language that you want to use? Has anyone solved a similar problem before with the language that you are thinking of using?
Scala, Erlang and Clojure are all versatile, powerful tools, well suited to many aspects of the wider domain of software engineering, so in the end rather than picking the one that is "best for X", pick the one that is best for you, in every way possible, and it is likely that you will have a good outcome.
Scala's type system is pretty complex, which can be a hindrance to those who don't take the time to understand it or a help to those who do. It is more multiparadigm than strictly functional, meaning, you can mix imperative, object-oriented, and functional code. This enables you to ease into functional programming, but may also prove to be a hindrance as you may frequently be tempted to just go imperative if you can't figure out a functional approach. There are good books available like Functional Programming in Scala by Paul Chiusano and Rúnar Bjarnason. Scala plays well with Java, making
Scala's type system is pretty complex, which can be a hindrance to those who don't take the time to understand it or a help to those who do. It is more multiparadigm than strictly functional, meaning, you can mix imperative, object-oriented, and functional code. This enables you to ease into functional programming, but may also prove to be a hindrance as you may frequently be tempted to just go imperative if you can't figure out a functional approach. There are good books available like Functional Programming in Scala by Paul Chiusano and Rúnar Bjarnason. Scala plays well with Java, making it a helpful thing to fit into my toolkit.
I can't comment with any expertise on the others you've listed but I'll give you some lightweight subjective impressions.
I like Lisps and gave Clojure a try but for some reason I just can't get into it; it seems hard to find information on how to do things, and I was daunted by having to learn yet another build system (leinengen) just to make anything. Other alternatives are Common Lisp, Scheme, and Racket. Learning one of the Lisps is extremely worthwhile for the perspective it gives you on programming. I find it makes you better in any language.
I played with F# a little but I'm a Linux guy and not so interested in Microsoft stuff. I did like what I saw, though. It's a derivative of OCaml, which on Linux is a remarkably fast language compared to other FP languages I've tried, rivalling and sometimes beating C.
Haskell is very purist; the temptation to code in a non-functional way would not be available to you, and it also has a few really good tutorials. I may come back to it someday if I have time. Nothing quite gets the love of FP fans that Haskell does; I'd almost say it is like a cult following without intending any disrespect to Haskell lovers. From what I can see their devotion to Haskell is well deserved.
For marketability I suspect Scala and F# are near the top of the FP heap IMO. Marketability has never been a good measure of the best tools, though.
Thanks for the A2A, hope it helps.
Common Lisp is much of the inspiration of Clojure. Clojure IS a Lisp. Clojure allows you to leverage things from the Java world though, which is a big advantage and to run on the JVM which is also a pretty strong thing. All other Lisps have their own runtime environments as well.
However, Lisp is fairly fast and loose about types and Haskell is damn strict about types.
Otherwise, functions wrapped in functions. Code is data. Kind of the same at that point.
The main (only?) reason is that Scala runs on the Java Virtual Machine and can use all of the libraries there and integrate (relatively) easily with projects that use Java. F# has a similar role in the .NET framework.
Haskell is most definitely the best language for teaching and learning functional programming as it literally provides no other options.
Scala in my honest opinion is just a pimped out Java. It's more useful than Java, and, even Java being more practical than Haskell for most tasks, means Scala is more practical than Haskell for almost any single project aside from those tailored specifically to Haskell.
I say the above having used both Java and Haskell for real projects.
The main reason to use Erlang or Elixir is that you have a highly process-level concurrent problem that needs high availability and reliability. The real secret sauce is that these languages run on the BEAM VM, which is tailored for just this kind of system. This comes from a Clojure fan, but one who has great respect for Armstrong’s team at Erickson and what they accomplished with their software architecture.
This is a limited choice. Here is my personal ranking:
- Haskell: It is a great language but if you are into theoretical stuff (Mathematics, logic, algebra, etc.). It is the only remaining strictly pure functional language. It is very good for parallelism also.
- Ocaml: It is not a strictly functional language (you can do loops and so on). It now has reasonable tooling (dune, opam, tuareg, etc.) but is still not wonderful. It is mostly on Unix.
- F#: It is the Windows version of Ocaml by Microsoft and is fully integrated with the Windows environment and APIs.
- Erlang/Elixir: It is why I answer here. Erla
This is a limited choice. Here is my personal ranking:
- Haskell: It is a great language but if you are into theoretical stuff (Mathematics, logic, algebra, etc.). It is the only remaining strictly pure functional language. It is very good for parallelism also.
- Ocaml: It is not a strictly functional language (you can do loops and so on). It now has reasonable tooling (dune, opam, tuareg, etc.) but is still not wonderful. It is mostly on Unix.
- F#: It is the Windows version of Ocaml by Microsoft and is fully integrated with the Windows environment and APIs.
- Erlang/Elixir: It is why I answer here. Erlang is very good but as far as I know, Elixir is strictly better than Erlang and is fully compatible with Erlang (It works on the same Erlang Virtual Machine). Erlang/Elixir is very good for servers handling a lot of traffic (For example Whatsapp is in Erlang). It has the Phoenix web environment.
- Scala: a JVM language. Very powerful but relatively complex language. Can be used in all areas where Java is used.
- Clojure: a JVM language that is Lisp-like. Same use case as Java but you have also ClojureScript a cross compiler to Javascript.
So, it all depends on your use case.
I am very fond of lisp family languages so I am biased towards Clojure.
Scala is too much like Java which is not a virtue based exclusively on my emotional scars.
Erlang is fun but a little quirky for my aesthetic biases
Most importantly is that none of these languages are that popular in large organizations where I usually end ...
Take all the answers you got from everyone else and add these 3 reasons why YOU (and not necessarily everyone else) should immediately dive deeply into F#:
- XAMARIN - Learn F#, and you can use your "functional-first" programming skills to do native app development for Android, iOS, OSX and Windows.
- ASP.NET CORE 1.0 - Core 1.0 is a complete rewrite of asp.net, and it lets you use F# to write web apps, and those web apps run 600 percent faster than Node.
- WebSharper - Compile F# to JavaScript and build great web apps.
Because it is the only language that runs in web browsers, JavaScript has been a
Take all the answers you got from everyone else and add these 3 reasons why YOU (and not necessarily everyone else) should immediately dive deeply into F#:
- XAMARIN - Learn F#, and you can use your "functional-first" programming skills to do native app development for Android, iOS, OSX and Windows.
- ASP.NET CORE 1.0 - Core 1.0 is a complete rewrite of asp.net, and it lets you use F# to write web apps, and those web apps run 600 percent faster than Node.
- WebSharper - Compile F# to JavaScript and build great web apps.
Because it is the only language that runs in web browsers, JavaScript has been a necessarily evil. However, it wasn't until Node.JS that it's full stack potential was realized, making JavaScript THE hot langauge of the day.
But F#... it can now do full stack web, and native apps. That is a LOT more benefit than learning any other FP anyone could name.
I adore Elm, a pure FP that compiles to HTML, CSS and JavaScript, but I also adore getting stuff done in the smartest way practical, and .NET CORE and Xamarin have just vaulted F# to the top of the REALLY smart langauge to learn list.