Practice makes it perfect.
Be persistent and consistent, don't leave unsolved problems hanging, force yourself to re-solve the older ones you had difficulties with, search TopCoder for the hardest easy problems (lowest acceptance rate).
The rest will follow.
If you became good enough at algorithm design and implementation to reach a yellow rating on TopCoder, you would be much better at algorithm design and implementation than the average programmer. There's a good chance you'd be able to get a job at a selective software company such as Google or Facebook, and you would have a strong ability to write efficient code for processing large amounts of data, which is an important skill.
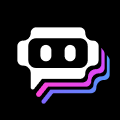
Progressing from Blue to Yellow on TopCoder involves improving your problem-solving skills and understanding of algorithms and data structures. Here are some steps to help you make this transition:
- Understand the Ratings: Familiarize yourself with the rating system. Blue (1200-1399) and Yellow (1400-1599) are the two tiers you are aiming to move between. You'll need to consistently perform well in competitions to increase your rating.
- Practice Regularly:
- Participate in as many contests as you can. Regular competition helps you get used to the pressure and the format of the problems.
- S
Progressing from Blue to Yellow on TopCoder involves improving your problem-solving skills and understanding of algorithms and data structures. Here are some steps to help you make this transition:
- Understand the Ratings: Familiarize yourself with the rating system. Blue (1200-1399) and Yellow (1400-1599) are the two tiers you are aiming to move between. You'll need to consistently perform well in competitions to increase your rating.
- Practice Regularly:
- Participate in as many contests as you can. Regular competition helps you get used to the pressure and the format of the problems.
- Solve problems from past contests, especially those rated around your current level and slightly above. - Focus on Weak Areas:
- Analyze your performance after each contest. Identify the types of problems you struggle with and focus on improving those areas.
- Use platforms like TopCoder’s practice problems and other competitive programming sites (like Codeforces, LeetCode, or HackerRank) to broaden your skills. - Study Algorithms and Data Structures:
- Ensure you have a solid understanding of common algorithms (sorting, searching, dynamic programming, graph algorithms, etc.) and data structures (arrays, linked lists, trees, graphs, etc.).
- Books like "Introduction to Algorithms" by Cormen et al. or "Competitive Programming" by Halim can be very helpful. - Learn from Others:
- Watch tutorials or streams from higher-rated competitors. Observing their thought processes can provide insights into problem-solving techniques.
- Join forums or discussion groups on platforms like TopCoder, where you can ask questions and learn from the community. - Work on Speed and Accuracy:
- In contests, time management is crucial. Practice solving problems within a set time limit to improve both speed and accuracy.
- Focus on reading problems carefully to avoid misinterpretation, which can lead to unnecessary mistakes. - Participate in Marathons and SRMs:
- Engage in Single Round Matches (SRMs) and Marathon Matches. SRMs are timed competitions that can help you improve your speed, while Marathons allow for longer problem-solving sessions. - Stay Motivated and Persistent:
- Progress in competitive programming can be slow and requires persistence. Set small, achievable goals to keep yourself motivated.
- Celebrate milestones, such as solving a particularly difficult problem or improving your rating.
By following these strategies and continuously challenging yourself, you can enhance your skills and work your way up to a Yellow rating on TopCoder. Good luck!
I was asking exactly the same question around a year-and-half back. How can I go from grey/green to blue/yellow on TopCoder.
Now that finally I have touched yellow and crossed 1700 (totally unexpected) I think I can share at least my story.
It goes without saying that it is important to get the basic algorithms and implementation clear. There are tons of lists and resources available online which can help you cover those.
The main reason I couldn’t touch blue/yellow was, I didn’t use pen-paper as much as I should and it resulted in me changing the solution/idea in middle of the code which finally
I was asking exactly the same question around a year-and-half back. How can I go from grey/green to blue/yellow on TopCoder.
Now that finally I have touched yellow and crossed 1700 (totally unexpected) I think I can share at least my story.
It goes without saying that it is important to get the basic algorithms and implementation clear. There are tons of lists and resources available online which can help you cover those.
The main reason I couldn’t touch blue/yellow was, I didn’t use pen-paper as much as I should and it resulted in me changing the solution/idea in middle of the code which finally resulted in nothing but a mess. If this is what happens to you, this answer just might help you.
The biggest help that I got was probably from this link, thanks to Paramdeep. That thread is a gem hidden in TopCoder forums. In the nutshell, following are the key points that helped me:
- Make a spread sheet to keep track of the problems that you solve.
- Make a list of around 50 Div-1 250 problems, and try to solve them all. Try your best to submit the correct solution in one go. Make it a point to submit once and submit correct.
- Once you get comfortable in getting the solution right in one go, make a list of another 50 Div-1 250 problems, and this time try to solve the problems within a set time limit.
- Whenever you read a problem, just leave the keyboard, pick up your pen and start working out the details like, proof of the solution, time limit, corner cases etc. If possible, try to have an outline of the code too, like the functions that you might need, data structure etc. This will help you in reducing the chances of error and also the length of the code. Make sure when you start typing, you have a clear idea about what you are going to implement and why that solution will work.
I agree there is no shortcut to practice but there are always some more productive ways to do so. When someone can reach 1724 from 473, why can’t you :).
Hope it helps !!
Good Luck
I understand your plight, friend. I also wanted to try topcoder after solving codechef and hackerrank for so long.. and I was just as clueless when I first started ;-)
Here are stepwise instructions on how to get started with Topcoder for a beginner:-
- Create a Topcoder account. I'm sure you can figure out how to do that.
- Go to Topcoder Arena. On the top, you'll see four buttons. Click on Practice problems. (Should be the second button).
- Click on filter on the right side. Select the points and difficulty level. As you are a beginner, try selecting Easy and 250 points, and filter it.
- Click on any pro
I understand your plight, friend. I also wanted to try topcoder after solving codechef and hackerrank for so long.. and I was just as clueless when I first started ;-)
Here are stepwise instructions on how to get started with Topcoder for a beginner:-
- Create a Topcoder account. I'm sure you can figure out how to do that.
- Go to Topcoder Arena. On the top, you'll see four buttons. Click on Practice problems. (Should be the second button).
- Click on filter on the right side. Select the points and difficulty level. As you are a beginner, try selecting Easy and 250 points, and filter it.
- Click on any problem to begin with. You'll see a page with a “Problem area” and a “Coding area”.
- The problem area will have a problem statement, definition, constraints, examples and all. Read thoroughly and understand how to solve the problem.
- Move to the coding area. As the name suggests, this is where you'll be writing your code. For now, let's use the inbuilt editor only. You'll see the option of selecting your choice of programming language. Do it. C++/Java would be the standard choices. Also, choose the standard type of editor and show line numbers (for convenience).
- /*Important*/ This part is a bit different compared to other online judges like codechef or hackerrank. In c++, you might be used to writing your code inside the main function and then printing your answer. In Topcoder, however, you have to write your code inside classes and functions and then you have to return the value instead of printing it.
- So, include the necessary header files and specify the namespace you'll be using (for beginners). The name of the class and function prototype will be given in the problem statement itself. Declare a public class with the given name and also a function with the given name and parameters. Write your code inside that function and then return the value.
- Click on the compile button to compile the code. If your code runs fine, submit it by clicking on the submit button and continue. If you pass all test cases correctly, go to the next step. Else, go to step 5.
- Go to step 3, select a new problem, and repeat. Practice makes a programmer perfect ;-)
Thank you for reading. Cheers and peace.
First things first. You can't move from green to blue by practicing Div II Easy problems. These problems are only useful when you're just getting started and trying to master the language (e.g. C++ STL). To be able to solve Div II 500-pointers, my advice would be to stop practicing Div II altogether (with an exception of 1000-pointers). To be able to solve Easy and Medium in the competition setting, you've got to be able to solve Easy + Medium + Hard in the practice SRM simulation. To be able to solve Div II Hard in 30 minutes during the actual SRM, push yourself to solve it in, say 25 minutes
First things first. You can't move from green to blue by practicing Div II Easy problems. These problems are only useful when you're just getting started and trying to master the language (e.g. C++ STL). To be able to solve Div II 500-pointers, my advice would be to stop practicing Div II altogether (with an exception of 1000-pointers). To be able to solve Easy and Medium in the competition setting, you've got to be able to solve Easy + Medium + Hard in the practice SRM simulation. To be able to solve Div II Hard in 30 minutes during the actual SRM, push yourself to solve it in, say 25 minutes, during the practice. This advice can be generalized to learning anything fast. Think of it as hyperclocking for mind. Tim Ferriss talks about this in the episode of 'Trial by Fire' where he learns yabusame (Japanese horse archery) in 5 days. Here's the link to the relevant portion of the video - https://vimeo.com/8611471#t=24m30s.
Second, try to get a good grasp of the main algorithmic themes (dynamic programming, graphs, probability, etc.) by focusing on one topic at a time. You can find categorized problems from UVA on Algorithmist.com, for example. Or better, grow by solving problems on USACO - http://train.usaco.org/usacogate. USACO is great because they made it gradual. You can't skip chapters, to move to the next one - you've got finish the current. You start off with chapters on easy ad-hoc problems, then move on to graph traversals, greedy, number theory, etc.
Third, do the paper problems. Do exercises from COS 226 - Princeton's introductory algorithms course taught by Robert Sedgewick (http://algs4.cs.princeton.edu/home/). You can even take this course on Coursera these days. Personally, Sedgewick's book helped me master algorithms much better than CLRS and Knuth. Thanks to motivated examples, actual implementations of the algorithms presented, detailed illustrations, cool experiments and intuitive mathematical analysis I felt I was growing after every page. A human being is inductive - it's easier to comprehend new material if first presented with examples and the practical side, with the theory and generalizations developed afterwards. Unfortunately, many books and courses teach things the other way around.
Fourth, make solving hard problems a daily habit. You can use Seinfeld’s method. For every day you solve one hard problem, put X over that day in your calendar (or use 42goals.com). Your goal is to keep the chain of X's growing without interrupting it. 21 days later you would overcome the initial friction, and solving a TC problem would be the part of your daily routine.
Most importantly, enjoy the journey! Or how we say it on TopCoder, 'gl&hf!'
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of th
Where do I start?
I’m a huge financial nerd, and have spent an embarrassing amount of time talking to people about their money habits.
Here are the biggest mistakes people are making and how to fix them:
Not having a separate high interest savings account
Having a separate account allows you to see the results of all your hard work and keep your money separate so you're less tempted to spend it.
Plus with rates above 5.00%, the interest you can earn compared to most banks really adds up.
Here is a list of the top savings accounts available today. Deposit $5 before moving on because this is one of the biggest mistakes and easiest ones to fix.
Overpaying on car insurance
You’ve heard it a million times before, but the average American family still overspends by $417/year on car insurance.
If you’ve been with the same insurer for years, chances are you are one of them.
Pull up Coverage.com, a free site that will compare prices for you, answer the questions on the page, and it will show you how much you could be saving.
That’s it. You’ll likely be saving a bunch of money. Here’s a link to give it a try.
Consistently being in debt
If you’ve got $10K+ in debt (credit cards…medical bills…anything really) you could use a debt relief program and potentially reduce by over 20%.
Here’s how to see if you qualify:
Head over to this Debt Relief comparison website here, then simply answer the questions to see if you qualify.
It’s as simple as that. You’ll likely end up paying less than you owed before and you could be debt free in as little as 2 years.
Missing out on free money to invest
It’s no secret that millionaires love investing, but for the rest of us, it can seem out of reach.
Times have changed. There are a number of investing platforms that will give you a bonus to open an account and get started. All you have to do is open the account and invest at least $25, and you could get up to $1000 in bonus.
Pretty sweet deal right? Here is a link to some of the best options.
Having bad credit
A low credit score can come back to bite you in so many ways in the future.
From that next rental application to getting approved for any type of loan or credit card, if you have a bad history with credit, the good news is you can fix it.
Head over to BankRate.com and answer a few questions to see if you qualify. It only takes a few minutes and could save you from a major upset down the line.
How to get started
Hope this helps! Here are the links to get started:
Have a separate savings account
Stop overpaying for car insurance
Finally get out of debt
Start investing with a free bonus
Fix your credit
Don't make a mistake many people are prone to:
Don't make a mistake many people are prone to:
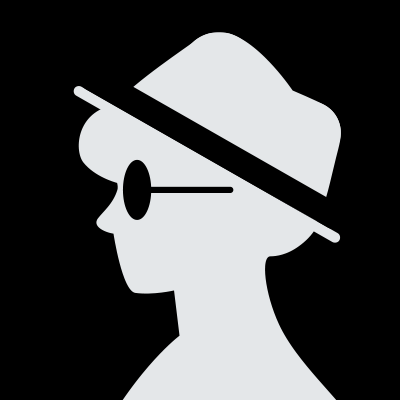
Yes, It took me one and half year since I started learning programming in order to hit yellow.
As mentioned on the other answers to become yellow you just have to solve the 250 in a decent time 200+ is enough I guess.
Here's a list of topics that can help you to become yellow
- Number theory basics (divisibility, prime factorization, gcd, lcm, modular arithmetic) sometimes the 250 relies on those.
- Little knowledge of probability and combinatorics.
- Dynamic programming: this is really your ticket to become yellow, it also can help you if you are still in div2 to solve div2-1000,
- Graph algorithms: tra
Yes, It took me one and half year since I started learning programming in order to hit yellow.
As mentioned on the other answers to become yellow you just have to solve the 250 in a decent time 200+ is enough I guess.
Here's a list of topics that can help you to become yellow
- Number theory basics (divisibility, prime factorization, gcd, lcm, modular arithmetic) sometimes the 250 relies on those.
- Little knowledge of probability and combinatorics.
- Dynamic programming: this is really your ticket to become yellow, it also can help you if you are still in div2 to solve div2-1000,
- Graph algorithms: traversals and their applications (i.e finding strongly connected components), shortest paths algorithms, msts, solving problems on DAGS, network flows can help sometimes as well.
- Game theory: a little game theory can help you get the 250 really fast sometimes.
Good resources for those are topcoder tutorials, Introduction to algorithms 3rd ed., competitive programming book (there's a free copy online for competitive programming 1).
So working on those topics on online judges like UVa, and solving division 2 srms (250, 500, 1000) for one and half year should be enough.
However moving from yellow to red is a lot harder and requires more knowledge and practice I've been stuck at yellow for one and half years so far btw.
Good luck and practice hard 1250 hours of practice can sure get you to yellow.
Thanks for A2A
From discussion with a Top 10 at hackerrank suggests me, that Red on CodeForces is not as easy as top100 in practice at hackerrank. I don’t strongly believe it, but mine is just a view.
If 4 months is enough or not depends on a lot of factors, which I will detail below, in the ‘how’.
Here is a strong plan that will work, to get Red in 4 months:
1. good understanding of maths - 25% would be required - learn as you need, spend 1/2 month
2. good understanding of data structures - 50% - learn this first, as a must, spend 1 & 1/2 months
3. good understanding of algorithms - 25% - learn thi
Thanks for A2A
From discussion with a Top 10 at hackerrank suggests me, that Red on CodeForces is not as easy as top100 in practice at hackerrank. I don’t strongly believe it, but mine is just a view.
If 4 months is enough or not depends on a lot of factors, which I will detail below, in the ‘how’.
Here is a strong plan that will work, to get Red in 4 months:
1. good understanding of maths - 25% would be required - learn as you need, spend 1/2 month
2. good understanding of data structures - 50% - learn this first, as a must, spend 1 & 1/2 months
3. good understanding of algorithms - 25% - learn this last, spend 2 months
As you can see data structures is going to be the crucial.
But, you also need to have few more:
- Good english 100% required
- So you can understand question correct in 2 or 3 times reading
- Most time is wasted in assumptions in reading it wrong due to hurry, etc.
- Patience 100% required
- Even when you feel it is easy, you need to take time & understand challenge in a complete way, this will save a lot of hours of debugging
- Good debugging skills 100% required
- When the question is easy, but you somehow understood it wrong, so you need to fix to get those easy points
- When the question is hard, but you somehow understood it wrong, there is very little you can do, because you would have wasted multiple days, and to come back will look wasting more time. I always left the question if this happened to save time.
- Understanding of various types of Algorithms like Greedy, Dynamic programming, etc. 100% required
- Some times something needs to be solved only by Dynamic programming, if you dont know it, it will take a lot of hours, my first DP took 3 days to solve, since I dint know what DP is, I reinvented the wheel, wasting more time.
- Reverse engineer the solution from test cases
- I have used this for about 4 times for medium/hard questions mainly
- It was kind of fair, because question was so poorly/ambiguously worded that, either I can’t understand the question or I get 2 or 3 meanings from it, though I get a strong feeling that it is solvable easily. After downloading may be 2nd test case and 5th test case, I understand the question & would solve it simply, or left it as it is not understood even then.
- Patch the answer
- I have used this 2 times, for a score of 10
- I wont tell what this is, at least publicly, because it is possible that cleverly written code can beat the system, defeating the purpose of a competitive programming website. This is incorrect to use, I have used for a mere points to verify if this is possible.
- Knowing Algorithm complexity is one of the key things 100% required
- Knowing a lot DS is ok, but knowing each DS’s operation like insert, delete, etc., which are like small algorithms, will become crucial, after solving challenges for 10 to 15 days.
- Steel nerves in terms of metal strength
- There will be times, that will test your belief, if you can ever achieve, if you are doing the right thing, etc. so a strong motivation will help.
- Deep thinking
- Leave the question for coming day, if you are not able to solve, only by solving, not taking help, you can enhance your skills to solve
- Read a book
- I never did, suffered a bit too. A book author pours his/her insights/deep understanding in the book, which will be helpful.
- Make a lot of notes
- On how you solved in your own words
- Rewrite from scratch
- Even if some challenge is similar to last one, rewrite from scratch, when done see back to earlier template you had - this helps in simpling the answer template many times a week
- Solve in practice or earlier completed contents
- To apply theory read from book or online, to have deep understanding of various topics.
- No time to waste
- since 4 months is too less, and you are starting from novice, there will be no time to waste
- so, you will have to do only this, and nothing else. I left my job here, it is one of the biggest risks I have taken.
- Health - a critical one to take care, eat protein diet and do some exercise.
- Solving while standing, and walking will help in solving.
There could be a bit more that will affect your progress, can’t recall now.
Simply, everyday stress will be heavy if you have a deadline like 4 months.
One last thing, I never had a single white hair, but when I achieved 100 rank, I saw 10 white hair in just 4 months, luckily I stopped doing algorithms at that time. You might say only 10 white hair, but I think, we are Not built to do some crash courses to catch-up others. So take care of your health, and, note that, after you succeed to do it or failed to do it, you may realize that, it was not worth to do what you are trying to do now, so think through before even you start on how much this is important for you. Sure, I will help if you need, by providing you links to various topics that you will have to learn & where to learn which I could not share till now in one post.
Best of luck.
I have to say a few things here, regarding different parts of your question.
- Most likely there is no way for you to become red in 6 months. You already participate in contests for a year, there are some rated events on your profile so it will be harder to get red by simply performing well once or twice (you can’t get +900 in a single round now as your cap is lower), therefore you need a lot of performances of a person with strong red skills, and you don’t even have enough time for it - even assuming you’ll reach red level of skills in 6 months, you likely won’t get it displayed by your rating b
I have to say a few things here, regarding different parts of your question.
- Most likely there is no way for you to become red in 6 months. You already participate in contests for a year, there are some rated events on your profile so it will be harder to get red by simply performing well once or twice (you can’t get +900 in a single round now as your cap is lower), therefore you need a lot of performances of a person with strong red skills, and you don’t even have enough time for it - even assuming you’ll reach red level of skills in 6 months, you likely won’t get it displayed by your rating by that time. And it seems that you aren’t going to reach red. Here are my reasons to think so:
- You did it for a year already, from your words it sounds like you didn’t improve fast over that time, therefore there is no reason to think that you have some sort of talent or hardworking skills or anything like that, as a result I think your chances to reach red so fast are around the same as chances of random person who’ll start from scratch, maybe even smaller. And I’m not aware of any example of person making this, so you’ll impress me a lot in case you’ll succeed.
- You are doing competitive programming “to get a job”, and from my experience such people rarely reach any significant results; most of the top performers I know are doing competitive programming because they enjoy solving problems or they love math/algorithms or they love the feel of competition itself or they like to challenge themselves.
- You are asking a question which has been asked in one way or another by multiple people already (I barely see what makes it so different and special that you’ll ask it again instead of reading previous answers to all these “how to become awesome as fast as possible” and using Google). It could mean that you aren’t good in working with information, therefore you’ll be slower learner, which implies longer time to reach red.
- You are looking for shortcuts; once again, from my experience - asking questions like this is an indicator of lower expectations, on average.
- 10 hours a day is actually not too much - it might turn out that you’ll need to spend even more :) But I’d say that you have very little chances in general, so it doesn’t matter anyway. And no, I’m not aware of any “magical lists of problems that will make you cool”. I may suggest, for example, solving 1000 problems from Timus OJ. Does it sound fine for you? I bet you want some shorter list.
- It is very likely that you are making mistake by assuming that getting red rating will give you an awesome job. The red rating itself doesn’t guarantee getting a job (check other answer here already, or some answers at Do you know any red/yellow coders who got rejected in Google/Facebook interviews? or see my story at Bohdan Pryshchenko's answer to Do top ranked (top 100) coders on sites like TopCoder or in programming competitions like ACM ICPC, Google Code Jam, or Facebook Hacker Cup find it easy to get a high-paying job at companies like Google, Facebook, etc.? ). And even if it is true that having red rating is a way to increase your chances - I bet you’d better spend your time working on improving your skills&resume directly, instead of doing activity that has job opportunities as a side effect only. Just think how much you can learn by spending 10 hours a day for 6 months on your self-improvement.
Thanks a lot for the A2A.
As far as the coloring system on Topcoder or Code forces is concerned, it is difficult to become a red on either of these sites. But not IMPOSSIBLE ;)
The only way I know to get the ranking I want on these sites is just a lot of Practice.
How do I become y or r on top coder or code forces basically boils down to performing well in the competitions.
Now will you perform well in competitions if you are well aware of the data structures and algorithms and the tips,tricks and hacks useful in the competitions. Now, what I will tell you is basically the same as what I would
Thanks a lot for the A2A.
As far as the coloring system on Topcoder or Code forces is concerned, it is difficult to become a red on either of these sites. But not IMPOSSIBLE ;)
The only way I know to get the ranking I want on these sites is just a lot of Practice.
How do I become y or r on top coder or code forces basically boils down to performing well in the competitions.
Now will you perform well in competitions if you are well aware of the data structures and algorithms and the tips,tricks and hacks useful in the competitions. Now, what I will tell you is basically the same as what I would tell a newbie. The only difference being that you are aware of what you want to achieve and are motivated enough to get it. So getting straight to the technical stuff, you should practice as much as you can on these topics.
It's also about the ability to think on the spot and come up with a solution. That said, there is a broad range of algorithms that might come in handy achieving such a task. Following are the ones I think most helpful:
- Graph Algorithms: BFS, DFS, Dijsktra, Floyd-Warshall, Bellman-Ford, MST (Prim and kruskal), Heavy-Light Decomposition, SCC and Euler Tour.
- Flow Algorithms: Maximum Flow, Min-Cost Max-Flow, Maximum Bipartite Matching and Hungarian Algorithm
- Dynamic Programming
- Geometry
- Number Theory: GCD, LCM, Extended Euclidean, Euler totient function , sieve of Eratosthenes and Probabilistic Primality Checks.
- String Algorithms: KMP, Boyre Moore, Z-Function and Aho-Corasik
- String Data-structure: Suffix Arrays and Tries.
- Advanced Data-structures: Segment trees, Binary Indexed Trees, Treaps and Skip Lists.
- Combintorial Games: Nim Game and Grundy Numbers.
- Backtracking and pruning.
- Meet in the middle.
Apart from this, do check out this awesome list of data structures and algorithms on code chef
Data Structures and Algorithms
I like to call them the 81 topics of Fame :p
(Practice)^inf
I know that after a certain point, this actually pisses you off because no one tells you From where to practice. So let me just share this awesome website I found that has the question to almost all the important topics and that too difficulty wise. That is freakin amazing !!!
A2 Online Judge
Just a piece of advice from my side. Do not focus on the color red or yellow. Focus on how much do you gain out of every competition. These ranking are super volatile, but your knowledge is not. Just read what Thanh Trung Nguyen has to say about the coloring system
Believe in yourself and do the best that you can. Don't worry too much about rank or color, it does not matter much in real world :)
I used to care A LOT about my rating when I tried to reach red. What I did: Really carefully do the problems that I can solve and get panic of failing system test. I spent more time on reading and testing easier problems than I think I should. When I realize that I was not learning much from contests, I decided to start doing whatever I want. These days I usually open harder problems (D, E div 1) and think about them for a while in very early stages of contest, losing time doing the easier problems (and thus losing points). But I'm learning more this way :)
Happy Coding !!!!
I made a progress from newbie to candidate master in 6 months. There are two essential keys to make progress as far as I understood from my training:
The first one is learning all fundamental topics and practicing them online on sites like CodeForces, CodeChef, HackerRank, etc. While practicing never scare from the difficulty of the problem, somehow frightening problems like a Div.1E problem can be particularly educational for you.
The secondary is math and observation skills. Improve your math skills, also use math olympiad books sometimes! Work math and combinatorics problems from different si
I made a progress from newbie to candidate master in 6 months. There are two essential keys to make progress as far as I understood from my training:
The first one is learning all fundamental topics and practicing them online on sites like CodeForces, CodeChef, HackerRank, etc. While practicing never scare from the difficulty of the problem, somehow frightening problems like a Div.1E problem can be particularly educational for you.
The secondary is math and observation skills. Improve your math skills, also use math olympiad books sometimes! Work math and combinatorics problems from different sites, there are various resources. Furthermore, don’t underestimate the power of observation. In some cases, you can observe the answer to the problem and make an intuitive proof, voila!
Enjoy doing competitive programming! Good luck!
Depending on your skill level and how motivated you are, it can take a considerable amount of time. If you are highly motivated and have a knack for mathematics and problem solving, you can achieve red in roughly 4-5 months if you practice daily.
Now coming to the question of it being too difficult, the answer is: Yes, becoming red is quite difficult and it is very easy to lose hope. But becoming a yellow is as difficult. If you are able to solve Div. 1 250 problems consistently during SRMs, you will find yourself oscillating between blue and yellow.
I have talked to many yellow coders and one c
Depending on your skill level and how motivated you are, it can take a considerable amount of time. If you are highly motivated and have a knack for mathematics and problem solving, you can achieve red in roughly 4-5 months if you practice daily.
Now coming to the question of it being too difficult, the answer is: Yes, becoming red is quite difficult and it is very easy to lose hope. But becoming a yellow is as difficult. If you are able to solve Div. 1 250 problems consistently during SRMs, you will find yourself oscillating between blue and yellow.
I have talked to many yellow coders and one common opinion among them all is that making the jump from yellow to red is very difficult and requires lots ans lots of practice.
Edit: According to Bohdan Pryshchenko (in the comments), it may be possible to become red by consistently solving Div. 1 250 really fast.
Absolutely possible. Just do it. ;)
I detailed some of my experience as a blue and yellow coder here. In a nutshell, to stay a blue coder one should generally be able to solve Div 1 250 problems with some (but not necessarily perfect) consistency. To be a yellow coder one should have enough familiarity/skill with these problems to solve them more quickly and more consistently. These problems are not terribly difficult with a lot of practice but do require some skill (which is developed through practice) to solve quickly and consistently. If your coding or algorithmic ability is already up to sp
Absolutely possible. Just do it. ;)
I detailed some of my experience as a blue and yellow coder here. In a nutshell, to stay a blue coder one should generally be able to solve Div 1 250 problems with some (but not necessarily perfect) consistency. To be a yellow coder one should have enough familiarity/skill with these problems to solve them more quickly and more consistently. These problems are not terribly difficult with a lot of practice but do require some skill (which is developed through practice) to solve quickly and consistently. If your coding or algorithmic ability is already up to speed the transition can be easier.
It took me more than 6 months to get to yellow (I've been on Topcoder for a little more than a year); however, a lot of this was due to starting at low gray (due to a really, really bad start - I was probably close enough to a blue coder as my results were consistently good for the next few events) and flailing as I neared yellow (I attained 1482 in April 2014 and had a few mishaps shortly after). There are a decent number of users that get to yellow with only 6 months experience.
Please don't worry about spending a set amount of time/day to get a specific rating; it's not that productive to set a lot of goals before you actually start doing the work. I highly recommend you play around on Topcoder and see how you will fare with the problems at hand.
This question is impossible to answer. It all depends on how much time you spend practicing per week, how effectively you practice, and your level of aptitude for competitive programming.
I will say however that even if you can only solve the 250 pointer, if you can consistently solve it correctly and quickly, you will become yellow. If you can consistently solve the 250 correctly and quickly and solve the 500 occasionally, you can become red. If you look at it that way, it's not as hard as it might seem at first.
One of my friends on code forces started coding at the same time as I did. Its 1.5 years since then. I am still a pupil and he is Candidate Master. One thing that I noticed is that to become Expert is easy. There are two ways.
First, solve A, B and C consistently for about 3–4 contests. A and B are easy and C requires observation and some easy algo.
Second way, is a little easy. Solve A and B fast a
One of my friends on code forces started coding at the same time as I did. Its 1.5 years since then. I am still a pupil and he is Candidate Master. One thing that I noticed is that to become Expert is easy. There are two ways.
First, solve A, B and C consistently for about 3–4 contests. A and B are easy and C requires observation and some easy algo.
Second way, is a little easy. Solve A and B fast and lock your sol...
My guess is that the problem is your CV. Being yellow at TopCoder is more than enough to be able to show interesting ‘stuff’ on your CV.
What ‘stuff’? You decide. Writing ‘I’m yellow at TopCoder’ is not very meaningful, recruiters might not even know what that is.
What did you do? What are you passionate about? I’m sure you’re able to do interesting projects. Are you presenting them on your CV? My guess is that you’re not.
I was a low yellow contestant at TopCoder and was able to get into top 500 in Code Jam and Hacker Cup, and got a gold medal in my ACM regional. I think these are recognised as
My guess is that the problem is your CV. Being yellow at TopCoder is more than enough to be able to show interesting ‘stuff’ on your CV.
What ‘stuff’? You decide. Writing ‘I’m yellow at TopCoder’ is not very meaningful, recruiters might not even know what that is.
What did you do? What are you passionate about? I’m sure you’re able to do interesting projects. Are you presenting them on your CV? My guess is that you’re not.
I was a low yellow contestant at TopCoder and was able to get into top 500 in Code Jam and Hacker Cup, and got a gold medal in my ACM regional. I think these are recognised as interesting achievements by recruiters. I got interviews at Google and Facebook and passed both of them.
The TopCoder algorithm tutorials cover a lot of the math that is actually needed. It is not advanced stuff—it’s mostly things like modular arithmetic and basic combinatorics that anyone should be able to learn. The challenging part of TopCoder is rarely the math. It’s about dynamic programming, greedy algorithms, graphs, and stuff like that.
I am yet to explore topcoder, but I have been consistent on codeforces for an year now. I reached from pupil to candidate master in this one year. If you just appear for each contest, and upsolve A,B,C which are fairly easy problems, you can get to blue within 6 months. I feel, speed and accuracy are important than complicated algorithms and data structures for getting to blue.
If you are just looking for a magical resource, you can go for a2oj categories and practice codeforces div2, A,B,C .
Many excellent answers has already been posted but I will answer this question as I have been A2A, but in a different context. People have answered what you should do, here's what you shouldn't do, the mistakes I made in my first 6 months:
1.) Lack of knowledge about the websites.
It's important to know which website has what type of questions. You know yourself better than anyone, you know what you're lacking and therefore you should choose website accordingly. For example, if you lacking in dp then you should choose SPOJ and do dp specific problems. Hackerrank won't be good for that and if you
Many excellent answers has already been posted but I will answer this question as I have been A2A, but in a different context. People have answered what you should do, here's what you shouldn't do, the mistakes I made in my first 6 months:
1.) Lack of knowledge about the websites.
It's important to know which website has what type of questions. You know yourself better than anyone, you know what you're lacking and therefore you should choose website accordingly. For example, if you lacking in dp then you should choose SPOJ and do dp specific problems. Hackerrank won't be good for that and if you start solving dp problems on hackerrank you won't have as good exposure and improvement as compared to SPOJ. While Hackerrank is better for getting introduced to new algorithm because of its partial scoring model.
2.) Practice.
Practice a lot and never be content with yourself. They say "be satisfied with where you are", it's not like that while acquiring a skill. Never be satisfied. I always get this feeling that I used to practice less than what I do now and I am sure I will feel the same way 6 months from now when I look back at this present month.
3.) Circulate between websites.
I practiced only on Hackerrank in the start. I didn't knew other websites such as TopCoder, Codeforces and SPOJ even existed. Despite all being competitive programming websites questions on these websites differ, especially on TopCoder. Do not practice only on one website, after solving a significant amount of questions on one move onto other. This will help you familiarize with different types of questions.
4.)Upsolve.
I can't stress this enough, always upsolve questions after the contest. I didn't gave much attention to the problems I was not able to solve during contest and therefore I didn't knew about algorithms and techniques to be used to solve that problem. Upsolving is important for improvement, do it.
5.) Participate regularly.
As I said I didn't even knew TC and CF existed, my contest participation was on mostly on HR and sometimes on Codechef. Apart from your regular trainings participate in contests on all websites as well. The more you take part in contests the more you will learn and improve.
Now your questions,
Topcoder has participants mostly from European countries. Despite the UX of the applet being problem for some, the questions are good and that makes it worth competing. Though configuring it with plugins makes it as easier as other websites. SPOJ is not a contest site, it does not hold contests. It's a repository of questions which have been added through the years. Some of these questions are from on-site contests(like ACM etc). Codechef is an Indian website and most of the participants are from India. It holds regular contests, college contests and mirrors of ACM-ICPC regionals contests.
Just start with hackerrank's "30 days of code" like contests as those are tailored for newbie. Use textbooks only as reference, use editorials and some red-coder's code to learn(find some good coder whose code is easy to read and use that guy's code to learn). Use whichever language you like. ACM only allows C/C++ & java. C++ is the fastest and has STL so I recommend C++.
Thanks for the A2A!
1. Go to www.topcoder.com
2. [Create account and then] log in.
3. Follow first tab: Challenges -> topcoder Arena and the beta version of the arena will be opened.
4. In the top of your screen click on "Practice Problems" (third, green pencil picture).
5. Then you just select a problem name and you may start coding.
I have been using the applet version of the arena up until now, but I tested this web version and it works just fine.
Happy coding! ;)
A2A
As seen in question’s comments, you also said:
“I love competitive programming but never really gave the dedication it requires.”
Dedication is what is required to achieve something big for us.
1 year is a long period of time.
I would try for red even if I want yellow, worst case I will set as yellow.
I would also be willing to learn more Mathematics, as it is somewhat base for both DS & Algo in the 1st few months, like 2 months. All details are available on Topcoder tutorials (Data Science Tutorials), this also includes some mathematics (Mathematics for Topcoders)
Hope that helps.
It depends on your skills.
It can be divided into a number of phases.
- You can solve the easy 250 fast, but still can't solve the 500 in time. At that phase you would be grey rated, or maybe green, I don't know. When I started competing, I was already past that phase.
- You can solve the 250 and 500 in the contest time. Depending on the success rate of your solutions, you will start advancing in Div2 and will reach Div1 just to get back to Div2 on your first contest there.
- You solve the 250 and 500 easily, and sometimes the 1000. At that case, you will be oscillating. A couple of contests at Div2 fol
It depends on your skills.
It can be divided into a number of phases.
- You can solve the easy 250 fast, but still can't solve the 500 in time. At that phase you would be grey rated, or maybe green, I don't know. When I started competing, I was already past that phase.
- You can solve the 250 and 500 in the contest time. Depending on the success rate of your solutions, you will start advancing in Div2 and will reach Div1 just to get back to Div2 on your first contest there.
- You solve the 250 and 500 easily, and sometimes the 1000. At that case, you will be oscillating. A couple of contests at Div2 followed by a couple of contests at Div1.
- Now you can solve the Div1 250. That's a long phase. You are nearly guaranteed to stay in blue rate. And depending on how fast you can solve the 250, your rating will increase till you reach mid yellow. That's the phase I am in now.
- Red: You need to start solving the hard 500.
- Target: You should be able to solve everything.
The first 3 phases are quick, depending on your personal learning curve. I only spent about 15 contests in that phase, till I finally started to solve the Div1 250 easily and fast. As long as you are interested and passionate about problem solving, you will advance quickly and you will have all the basic knowledge and intuition in no time.
Go ahead, do some reading on Algorithms, get some practice. There is already about a hundred questions on this at Quora, not counting several thousands at TopCoder :-)
Well it may not get you a job in Google but am sure it fits pretty well in your Resume and if at all the interviewers ( When you apply for Google ) check your profile and see those achievements then there are a fair chances of you getting your dream job.
Or.
You may also use the term like "Search me on Google" in an active interview which is quite cool when they search you and see those badges which do create a cool impression on your skill set in coding.
Now to the part of how to get these badges.
- Keep coding, May that be watever it takes.
- You will improvise day by day.
- Learn from every s
Well it may not get you a job in Google but am sure it fits pretty well in your Resume and if at all the interviewers ( When you apply for Google ) check your profile and see those achievements then there are a fair chances of you getting your dream job.
Or.
You may also use the term like "Search me on Google" in an active interview which is quite cool when they search you and see those badges which do create a cool impression on your skill set in coding.
Now to the part of how to get these badges.
- Keep coding, May that be watever it takes.
- You will improvise day by day.
- Learn from every single question.( Am sure These site admins will get new problems all the time.
- Keep yourself motivated all the time, To avoid distraction Or to get a distraction maybe( In my case it was MUSIC, I code faster and better with Music on, Yay!)
The amount of dedication and skill is what the top companies like Google, Facebook,etc see in coders from here.
If it is Google that you dream of, Then be sure to show them the skill you have in you through any of those site like Topcoder, Topchef, Hackathon and a lot more.
Happy Coding, All the best.
I recommend always looking at the model solution after you have solved a problem. (If you can’t get AC on the problem, then you might still want to give up after a while and look at the solution, but there’s no easy answer to how long you should keep trying before giving up; it depends on your objectives.)
If the technique described is unfamiliar, then I suggest coding it up yourself, so that you’ll understand it and are more likely to retain it. Yes, you might forget it later, but that’s life.
Continue practicing.... daily work... reading tutorials, talking with friends, reading tons of code.. that's what i am doing. I am still gray too... but i think that both of us are in the correct way!
Good luck
Hii, If you are really interested in programming then TopCoder will help you practice and you can show your skills to the world by competing in various challenges. Head over to learn page in TopCoder and then register. Then you can find lots of challenges. Even recruiters post challenges to find great minds for their company.
Visit
https://www.youronlinecontest.com/posts/top-coder.htmlYour Online Contest site to know more about it and get started.
I don't think there can be a general answer to this question.
- Educational background.
- The time assigned to training.
- Training plan.
- Dedication.
- Eating spaghetti.
These things and many others affect the performance of a person, and they are not the same for everyone.
Pradeep George Mathias's Answer to What is needed to become good algorithmist like top rankers in Topcoder/Spoj/GCJ?
I will answer this question using TopCoder question difficulty levels as benchmarks.
The Div II 250 level kind: This level of questions are meant to be done at the rate of read,think-for-a-moment-atmost,code. They would test at best your ability to not make coding bugs, and to ensure that you can get it right the first time. There is almost never anything fancy in the problems.
What you need? A good enough grasp of the chosen programming language. You don't want bugs like integer
Pradeep George Mathias's Answer to What is needed to become good algorithmist like top rankers in Topcoder/Spoj/GCJ?
I will answer this question using TopCoder question difficulty levels as benchmarks.
The Div II 250 level kind: This level of questions are meant to be done at the rate of read,think-for-a-moment-atmost,code. They would test at best your ability to not make coding bugs, and to ensure that you can get it right the first time. There is almost never anything fancy in the problems.
What you need? A good enough grasp of the chosen programming language. You don't want bugs like integer overflow, or a boundary case left out, or unnecessary inclusion of excess special-cases. Keep it simple.The Div II 500/Div I 250 level kind: These are the brute-force/standard algorithms type of questions. They'd generally ask you to do a little bit of mundane coding (for a fast submitted solution). There would often be efficient ways to solve these questions which are an overkill because they'd be hard to code. Remember, you just need to pass in the required time, not the most efficient solution that is theoretically possible.
What you need? A good knowledge of standard algorithms (like BFS etc) and the ability to code them fast enough. Also, a little bit of discrete math / combinatorics would be useful in quickly reducing the problem to something familiar. Try to solve combinatorial puzzles separately also to acquire the skill to "see-through" the problem statements.The Div I 500 level kind: These kinds of problems are the ones with very neat solutions. They test your thinking to a fairly large extent, and your further implementation sufficiently.
What you need? When it comes to the DP problems, sufficient practice. Not mundane large-quantity, but pick up tricks as you go. Also, in terms of theory, again a fair grasp over combinatorics and basic discrete math, which is required to convert the problem in solvable blocks. Once thats done, theres just fitting everything smoothly together.General Tips:
If you don't know the solution, don't give up. Read an editorial. After you've read the editorial, its not over: Code the solution. Even if it is exactly from the editorial and you feel you've got the gist, practice coding it.Never get intimidated by what you need to code. Its easy to say, "oh I've solved it algorithmically", but thats not whats asked of you, is it?
If you find a kind of general-solution-method, try discussing it with others. You would probably be able to find smarter ways of implementing it, or even more general situations of applying it.
One good thing about places like TopCoder, is you get to read others' code. This can be very helpful in developing a clean coding style.
Keep practicing, keep enjoying!
Even if You are a Green Coder then also you might want to follow his advice.
Topcoder's purpose can be found on it's tagline saying "We Design. We Build. We Solve."
Like every other sport, topcoder improves the speed, accuracy, memory and creativity of the participants.
Participants solve problems that cannot be solved in notebooks such as hard mathematical problems, hard design problems etc., and gets rated. More his rating, more the chance he is good at problem solving.
Anyone with access to internet connection can use topcoder which means anyone can learn and compete.
Humans have various dreams.
An idea from the movie Wall E is now called "Google glass"
Topcoder's purpose can be found on it's tagline saying "We Design. We Build. We Solve."
Like every other sport, topcoder improves the speed, accuracy, memory and creativity of the participants.
Participants solve problems that cannot be solved in notebooks such as hard mathematical problems, hard design problems etc., and gets rated. More his rating, more the chance he is good at problem solving.
Anyone with access to internet connection can use topcoder which means anyone can learn and compete.
Humans have various dreams.
An idea from the movie Wall E is now called "Google glass"
For iron man fans :)
It is impossible to estimate "progress rate" from performance on problems of Div2 A-B/250pt level. These problems hardly ever require anything else than understanding the statement and putting together a loop or two.
Solving problems of next levels (as well as being yellow) require learning algorithms and recognizing when to apply them - which is a completely different skillset. Interpolation doesn't work :-)